/* fill in the code to declare a list_node_t pointer called head */
Help fill in code!
#if !defined(PRODUCE_H_INCL)
#define PRODUCE_H_INCL
#define PRODUCE_NAME 21
typedef struct produce {
char name[PRODUCE_NAME];
int inven;
} produce_t;
typedef struct list_node {
produce_t data;
struct list_node *restp;
} list_node_t;
typedef struct produce_list {
/* fill in the code to declare a list_node_t pointer called head */
int size;
} produce_list_t;
void read_produce_file (char filename[], produce_list_t *p);
void print_produce (produce_t prod);
void print_produce_list (produce_list_t p);
void write_produce_file (char filename[], produce_list_t p);
list_node_t * search_produce_list (produce_list_t p, char name[]);
int update_produce_list (produce_list_t *p, produce_t prod);
void print_produce_linked_list (produce_list_t p);
#endif

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

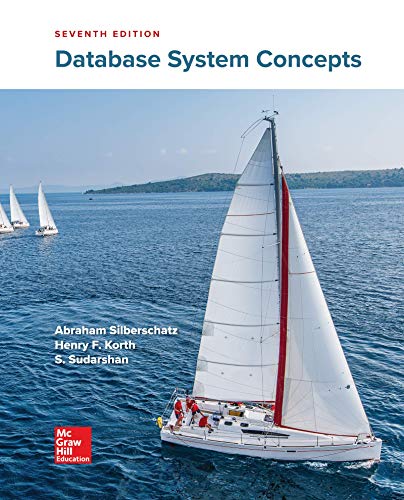
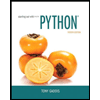
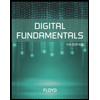
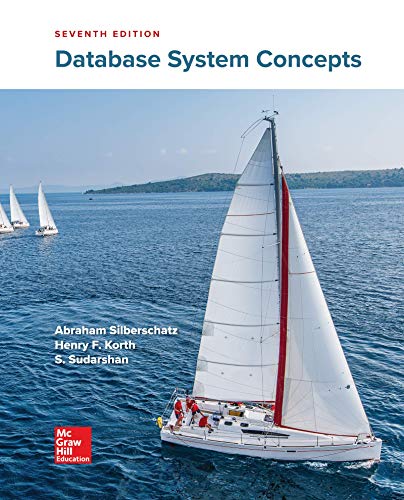
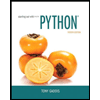
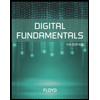
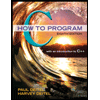
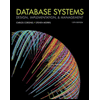
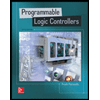