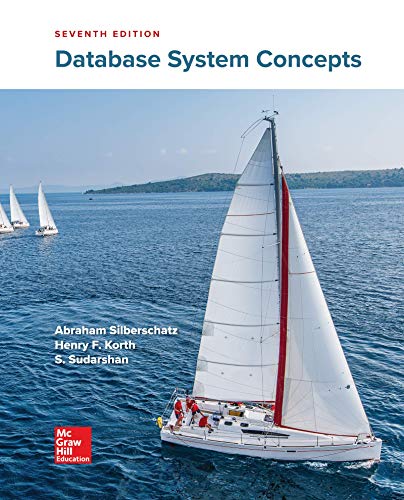
Complete the program 'Scatter-Reduce.c' to compute the product of all elements in a given array.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <mpi.h>
int *Random_Num_Generator(int Num_Elements);
int Compute_Product(int *array, int num_elements);
int main(int argc, char* argv[])
{
int comm_sz, my_rank;
int i, Result;
int Num_Per_Proc = atoi(argv[1]);
// Seed the random number generator to get different results each time
srand(time(NULL));
MPI_Init(&argc, &argv);
MPI_Comm_rank(MPI_COMM_WORLD, &my_rank);
MPI_Comm_size(MPI_COMM_WORLD, &comm_sz);
// Generate a random array of elements on process 0
int *Random_Nums = NULL;
if (my_rank == 0)
{
Random_Nums = Random_Num_Generator(Num_Per_Proc * comm_sz);
printf("The random numbers generated: \n");
for (i = 0; i < Num_Per_Proc * comm_sz; i++)
printf("%d; ", Random_Nums[i]);
printf("\n");
}
// Generate a buffer for holding a subset of the entire array
int *Sub_Random_Nums = (int *)malloc(sizeof(int) * Num_Per_Proc);
// Scatter the random integer numbers from process 0 to all processes
MPI_Scatter(Random_Nums, Num_Per_Proc, MPI_INT, Sub_Random_Nums, Num_Per_Proc, MPI_INT, 0, MPI_COMM_WORLD);
// Compute the product value of a subset array on each process
int Sub_Product = Compute_Product(Sub_Random_Nums, Num_Per_Proc);
// Reduce the total product value of all elements to process 0
MPI_Reduce(&Sub_Product, , 1, MPI_INT, , 0, MPI_COMM_WORLD);
if (my_rank == 0)
printf("Total product of all elements is %d\n", Result);
// Clean up
if (my_rank == 0)
free(Random_Nums);
free(Sub_Random_Nums);
MPI_Finalize();
return 0;
}
// Create an array of random integer numbers ranging from 1 to 10
int *Random_Num_Generator(int Num_Elements)
{
int *Rand_Nums = (int *)malloc(sizeof(int) * Num_Elements);
int i;
for (i = 0; i < Num_Elements; i++)
Rand_Nums[i] = (rand() % 10) + 1;
return Rand_Nums;
}
// Computes the product of an array of numbers
int Compute_Product(int *array, int num_elements)
{
int product = 1;
int i;
for (i = 0; i < num_elements; i++)
product *= array[i];
return product;
}

Step by stepSolved in 2 steps with 1 images

- C++arrow_forwardC code that prints the elements of a 5 * 4 integer array whose elements are generated randomly.arrow_forwardPython Please **Bold is code** Recap of two-dimensional arrays and their numpy implementation import numpy as np my_2d_array = np.array([[1,2],[3,4],[5,6]]) print("This is my_2d_array:") print(my_2d_array) print("This array has shape " + str(my_2d_array.shape) + " as there are " + str(my_2d_array.shape[0]) + " rows and " + str(my_2d_array.shape[1]) + " columns.") print("The entry of the array with row index " + str(1) + " and column index " + str(1) + " has value " + str(my_2d_array[1,1])) 1) In this problem, implement a TwoDArray class that is meant to mimic (some of) the functionality of a two-dimensional numpy array. DO NOT use numpy at any point. 1A) Give the class an __init__ method Make sure that the variable array is a valid input. This means you should a) Make sure that array is a list of lists. and b) Make sure that each of the inner lists has the same length. You do not need to check for anything else. Rise the appropriate error(s) if these conditions are not met. It is up…arrow_forward
- Using C++ Language Need help on below code, I have the code but need to compute maximum of the array. Code: #include<iostream> using namespace std; int main() { int size; cout<<" Enter array size: "; cin>>size; int *numList=new int[size]; cout<<"Enter "<<size<<" elements: "; for(int i=0;i<size;i++) { cin>> *(numList+i); } delete numList; return 0; }arrow_forwardUsing C++ languagearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
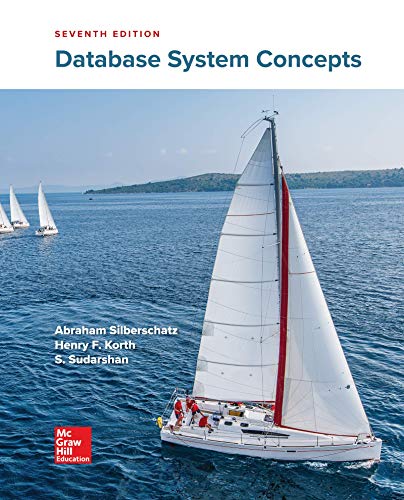
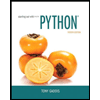
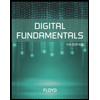
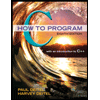
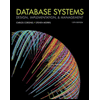
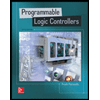