IN C# Given the following integer array: int[] intNumbers = new int[] {13, ‐42, ‐13, 1963, 0, 39, 42, 10, 1974, 3691, ‐17, 1226, ‐99}; a) output all elements in the array b) output the first and last elements in the array c) output the given array in the order shown d) output the given array in reverse order e) sort and output the array in ascending order f) sort and output the array in descending order g) output the value of the largest element in the array h) output the value of the smallest element in the array. For this program, you should not assume that you know the number of elements in the array. Remember the .Length property of the array will tell you how many there are. If you hard code 13 in your program you will get an “R”. Hint: Console.WriteLine(intNumbers.Length);
IN C# Given the following integer array:
int[] intNumbers = new int[] {13, ‐42, ‐13, 1963, 0, 39, 42, 10, 1974, 3691, ‐17, 1226, ‐99};
a) output all elements in the array
b) output the first and last elements in the array
c) output the given array in the order shown
d) output the given array in reverse order
e) sort and output the array in ascending order
f) sort and output the array in descending order
g) output the value of the largest element in the array
h) output the value of the smallest element in the array.
For this program, you should not assume that you
know the number of elements in the array.
Remember the .Length property of the array will
tell you how many there are. If you hard code 13 in
your program you will get an “R”.
Hint: Console.WriteLine(intNumbers.Length);

Step by step
Solved in 2 steps with 1 images

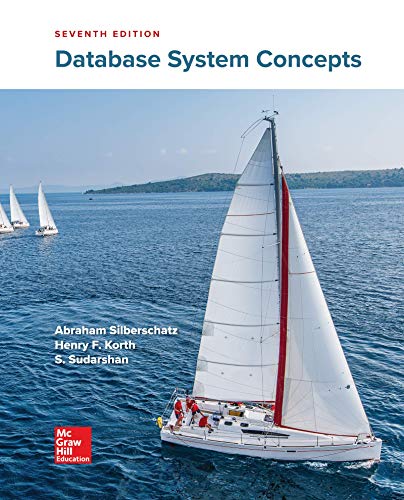
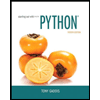
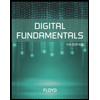
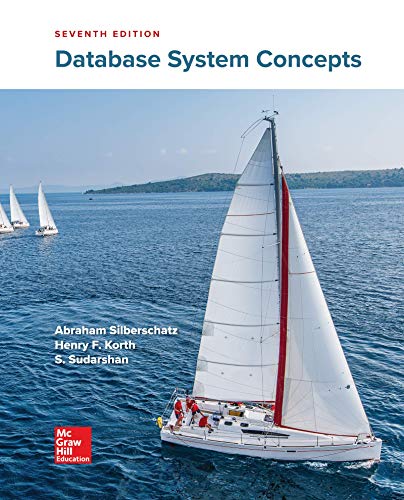
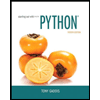
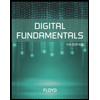
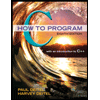
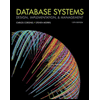
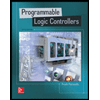