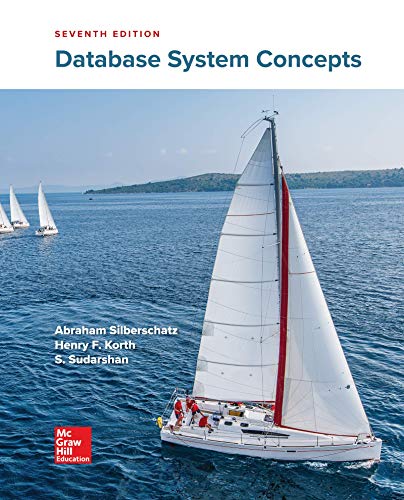
In this java program please run and explain the output, thank you
Source Code:
import java.util.Scanner;
public class Main
{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String name, glt;
System.out.print("Welcome to informatics Grading System");
System.out.println("\n");
System.out.print("Name of the student: ");
name = sc.nextLine();
System.out.print("Grade level and Track: ");
glt = sc.nextLine();
System.out.println("\n");
System.out.print("SUBJECTS:\nMath: ");
int math = sc.nextInt();
System.out.print("Science: ");
int science = sc.nextInt();
System.out.print("English: ");
int english = sc.nextInt();
System.out.print("Filipino: ");
int filipino = sc.nextInt();
System.out.print("Computer Education: ");
int computer = sc.nextInt();
double avgGrade = (math+science+english+filipino+computer)/5;
System.out.println("The Grade Average is: "+avgGrade);
if(avgGrade>74)
System.out.println("Remarks: Congratulations! You are Passed!");
else
System.out.println("Remarks: Sorry! You are Failed!");
System.out.println();
}
}
Thank you.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- Please take look at this java code and help me complete this code to compile together. //MainValidatorA3 public class MainA3 { public static void main(String[] args) { System.out.println("Welcome to the Validation Tester application"); // Int Test System.out.println("Int Test"); ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100); int num = intValidator.getIntWithinRange(); System.out.println("You entered: " + num + "\n"); // Double Test System.out.println("Double Test"); ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: "); double dbl = doubleValidator.getDoubleWithinRange(); System.out.println("You entered: " + dbl + "\n"); // Required String Test System.out.println("Required String Test:"); ValidatorString stringValidator = new ValidatorString("Enter a required string: "); String…arrow_forwardType the program's output import java.util.Scanner; public class NumberSearch { public static void findNumber(int number, int lowVal, int highVal, String indentAmt) { int midVal; midVal = (highVal + lowVal) / 2; System.out.print(indentAmt); System.out.print(midVal); if (number == midVal) { System.out.println(" a"); } else { if (number < midVal) { System.out.println(" b"); findNumber(number, lowVal, midVal, indentAmt + " "); } else { System.out.println(" c"); findNumber(number, midVal + 1, highVal, indentAmt + " "); } } System.out.println(indentAmt + "d"); } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int number; number = scnr.nextInt(); findNumber(number, 0, 8, ""); } } Input 5arrow_forwardCreate a Flowchart //Java Source Code :- import java.util.Scanner; public class bExpert { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("\nVALUES"); System.out.print("Value 1: "); int v1 = sc.nextInt(); System.out.print("Value 2: "); int v2 = sc.nextInt(); System.out.print("Value 3: "); int v3 = sc.nextInt(); System.out.println("\nPROCESS"); System.out.println("\n1. Summation"); System.out.println("2. Product"); System.out.println("3. Min / Max"); System.out.println("4. Odd / Even"); char st = 'y'; while(st != 'n'){ System.out.print("\nCHOICE: "); int ch = sc.nextInt(); switch(ch) { case 1: int sum = v1 + v2 + v3; System.out.println("\nSum = "+sum); break; case 2: int prod =…arrow_forward
- This is the code that needs to be corrected: // This application gets a user's name and displays a greeting import java.util.Scanner; public class DebugThree3 { public static void main(String args[]) { String name; name = getName(); displayGreeting(name); } public static String getName(name) { String name; Scanner input = new Scanner(System.in); System.out.print("Enter name "); name = input.nexlLine(); return name; } public static displayGreeting(String name) { System.outprintln("Hello, " + name + "!"); } }arrow_forwardTASK 5. Methods Overloading. Review Methods, Implement the following code, test it with different input, make sure it runs without errors.arrow_forwardusing System; class Program{ // Define PrintBoard method public static void PrintBoard() { Console.WriteLine(" X | | "); Console.WriteLine(" | | "); Console.WriteLine(" | O | "); Console.WriteLine(); } public static void Main(string[] args) { PrintBoard(); // Call PrintBoard method PrintBoard(); // Call it again PrintBoard(); // And again! }} This program is really simple and basic to print a tic tac toe board for the user to eventually play the computer in C#. How can it be modified a little bit to print the board and have each tile labeled with a number to identify the space? I'm a beginner and would like to take this step by step in modifying the code. Thank you!arrow_forward
- Correct Code for Java: Enter an integer >> 11 Enter another integer >> 23 The sum is 34 The difference is -12 The product is 253 import java.util.Scanner; public class DebugTwo2 { public static void main(String args[]) { int a, b; Scanner input = new Scanner(System.in); System.out.print("Enter an integer >> "); a = nextInt (); System.out.print("Enter b = nextInt (); System.out.println("The System.out.println("The System.out.println("The } } another integer >> "); sum is " + (a + b)); difference is " + (a - b)); product is + (a*b));arrow_forwardHi, I am struggling a bit with this problem. (This is in Java by the way.) Provided code: import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userText; // Add more variables as needed userText = scnr.nextLine(); // Gets entire line, including spaces. /* Type your code here. */ }}arrow_forwardJava - Help Please How do I output this statement as a horizontal statement with a newline? Everytime I use System.out.println, it makes the entire output vertical instead of horizontal. Program below. import java.util.Scanner; public class LabProgram { public static void main(String[] args) { int x; int y; Scanner scan = new Scanner(System.in); x = scan.nextInt(); y = scan.nextInt(); if(y >= x){ for(int i=x; i<=y; i = i + 5) { System.out.print(i+ " "); (The problem line) } } else{ System.out.print("Second integer can't be less than the first."); } }}arrow_forward
- JAVA CODE: check outputarrow_forwardimport java.util.Scanner; public class TriangleArea { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Triangle triangle1 = new Triangle(); Triangle triangle2 = new Triangle(); // TODO: Read and set base and height for triangle1 (use setBase() and setHeight()) // TODO: Read and set base and height for triangle2 (use setBase() and setHeight()) System.out.println("Triangle with smaller area:"); // TODO: Determine smaller triangle (use getArea()) // and output smaller triangle's info (use printInfo()) }} public class Triangle { private double base; private double height; public void setBase(double userBase){ base = userBase; } public void setHeight(double userHeight) { height = userHeight; } public double getArea() { double area = 0.5 * base * height; return area; } public void printInfo() { System.out.printf("Base:…arrow_forwardimport java.util.Scanner; public class leapYearLab { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int userYear; boolean LeapYear; System.out.println("Enter the year"); userYear = scnr.nextInt(); isLeapYear(userYear); //if leap year if (isLeapYear){ System.out.println(userYear + " - leap year"); } else{ System.out.println(userYear + " - not a leap year"); } scnr.close(); } public static boolean isLeapYear(int userYear){ boolean LeapYear; /* Type your code here. */ if ( userYear % 4 == 0) { // checking if year is divisible by 100 if ( userYear % 100 == 0) { // checking if year is divisible by 400 // then it is a leap year if ( userYear % 400 == 0) LeapYear = true; else LeapYear = false; } // if the year is not divisible by 100 else…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
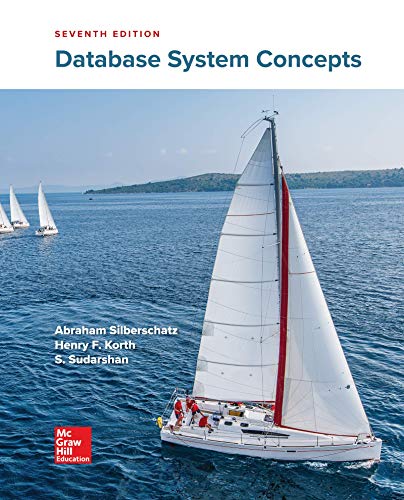
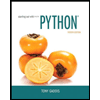
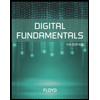
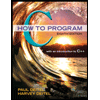
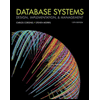
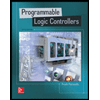