In this java program please explain everyline of this code. Source Code: // Online Java Compiler // Use this editor to write, compile and run your Java code online import java.lang.*; import java.util.*; public class HelloWorld { public static double ln(double x, double y) { double lnX = Math.log(x); double lnY = Math.log(y); double ln = lnX + lnY * 2; return ln; } // function to round to 2 decimal place // there is no function round(val,2) like python // to round to 2 decimal place // intead we use this public static double round(double a,int dec){ return Math.round(a * 100.0) / 100.0; } public static void main(String[] args) { System.out.print("Enter lower limit: "); Scanner sc = new Scanner(System.in); double lower = sc.nextInt(); System.out.print("Enter higher limit: "); double higher = sc.nextInt(); double iterations = (double)(higher - 1) / 0.05; double y = 1.05; double x = 0; double final_answer = 0; System.out.println("Function\t | Lower Limit\t | Upper Limit | Trapezoid Application (width=0.05) |"); for (int i = 0; i < iterations; i++) { double ans = ln(lower, y); lower += 0.05; y = lower + 0.05; final_answer += ans; x = round(lower, 2); x = round(x, 2); y = round(y, 2); System.out.println("_____________________________________________________" + "____________________________________________________________"); System.out.println("Iteration:" + round(x, 2)); System.out.println("(ln(x)+ln(x)/2)1 | x=" + round(x, 2) + " \t | x=" + round(y, 2) + " \t| (ln(" + x + ")+ln(" + y + ")/2)1 | Answer: " + ans); } System.out.println("_________________________________________________________"+ "________________________________________________________"); System.out.println(" Total Iterations:" + round(x, 2)); System.out.println("Final Answer/Summation of all iterations:" + final_answer); } }
In this java program please explain everyline of this code.
Source Code:
// Online Java Compiler
// Use this editor to write, compile and run your Java code online
import java.lang.*;
import java.util.*;
public class HelloWorld {
public static double ln(double x, double y) {
double lnX = Math.log(x);
double lnY = Math.log(y);
double ln = lnX + lnY * 2;
return ln;
}
// function to round to 2 decimal place
// there is no function round(val,2) like python
// to round to 2 decimal place
// intead we use this
public static double round(double a,int dec){
return Math.round(a * 100.0) / 100.0;
}
public static void main(String[] args) {
System.out.print("Enter lower limit: ");
Scanner sc = new Scanner(System.in);
double lower = sc.nextInt();
System.out.print("Enter higher limit: ");
double higher = sc.nextInt();
double iterations = (double)(higher - 1) / 0.05;
double y = 1.05;
double x = 0;
double final_answer = 0;
System.out.println("Function\t | Lower Limit\t | Upper Limit | Trapezoid Application (width=0.05) |");
for (int i = 0; i < iterations; i++) {
double ans = ln(lower, y);
lower += 0.05;
y = lower + 0.05;
final_answer += ans;
x = round(lower, 2);
x = round(x, 2);
y = round(y, 2);
System.out.println("_____________________________________________________" +
"____________________________________________________________");
System.out.println("Iteration:" + round(x, 2));
System.out.println("(ln(x)+ln(x)/2)1 | x=" + round(x, 2) + " \t | x=" + round(y, 2) + " \t| (ln(" + x
+ ")+ln(" + y + ")/2)1 | Answer: " + ans);
}
System.out.println("_________________________________________________________"+
"________________________________________________________");
System.out.println(" Total Iterations:" + round(x, 2));
System.out.println("Final Answer/Summation of all iterations:" + final_answer);
}
}
thank you

Step by step
Solved in 3 steps

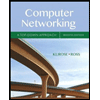
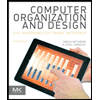
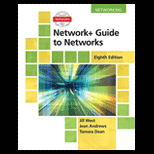
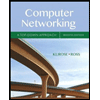
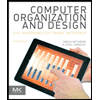
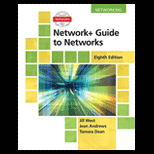
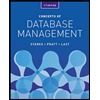
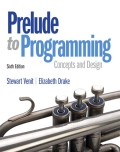
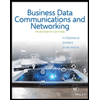