In this c language program, please explain everyline of this code. Source Code: #include #include //structures struct Date { int year; int month; int day; }; struct Product { char product_name [50]; struct Date manufacturing_date; struct Date expiry_date; }; int main() { //create two Product struct Product p1,p2,p3; strncpy(p1.product_name,"Product 1",15); p1.manufacturing_date.year = 2022; p1.manufacturing_date.month = 4; p1.manufacturing_date.day = 22; p1.expiry_date.year = 2024; p1.expiry_date.month = 5; p1.expiry_date.day = 22; strncpy(p2.product_name,"Product 2",15); p2.manufacturing_date.year = 2022; p2.manufacturing_date.month = 5; p2.manufacturing_date.day = 20; p2.expiry_date.year = 2024; p2.expiry_date.month = 8; p2.expiry_date.day = 15; //display product information printf("%s\n",p1.product_name); printf("manufacturing_date : %d-%d-%d\n",p1.manufacturing_date.year,p1.manufacturing_date.month,p1.manufacturing_date.day); printf("expiry_date : %d-%d-%d\n",p1.expiry_date.year,p1.expiry_date.month,p1.expiry_date.day); printf("\n%s\n",p2.product_name); printf("manufacturing_date : %d-%d-%d\n",p2.manufacturing_date.year,p2.manufacturing_date.month,p2.manufacturing_date.day); printf("expiry_date : %d-%d-%d\n",p2.expiry_date.year,p2.expiry_date.month,p2.expiry_date.day); return 0; }
In this c language program, please explain everyline of this code.
Source Code:
#include <stdio.h>
#include<string.h>
//structures
struct Date {
int year;
int month;
int day;
};
struct Product {
char product_name [50];
struct Date manufacturing_date;
struct Date expiry_date;
};
int main()
{
//create two Product
struct Product p1,p2,p3;
strncpy(p1.product_name,"Product 1",15);
p1.manufacturing_date.year = 2022;
p1.manufacturing_date.month = 4;
p1.manufacturing_date.day = 22;
p1.expiry_date.year = 2024;
p1.expiry_date.month = 5;
p1.expiry_date.day = 22;
strncpy(p2.product_name,"Product 2",15);
p2.manufacturing_date.year = 2022;
p2.manufacturing_date.month = 5;
p2.manufacturing_date.day = 20;
p2.expiry_date.year = 2024;
p2.expiry_date.month = 8;
p2.expiry_date.day = 15;
//display product information
printf("%s\n",p1.product_name);
printf("manufacturing_date : %d-%d-%d\n",p1.manufacturing_date.year,p1.manufacturing_date.month,p1.manufacturing_date.day);
printf("expiry_date : %d-%d-%d\n",p1.expiry_date.year,p1.expiry_date.month,p1.expiry_date.day);
printf("\n%s\n",p2.product_name);
printf("manufacturing_date : %d-%d-%d\n",p2.manufacturing_date.year,p2.manufacturing_date.month,p2.manufacturing_date.day);
printf("expiry_date : %d-%d-%d\n",p2.expiry_date.year,p2.expiry_date.month,p2.expiry_date.day);
return 0;
}
Thank you!

Step by step
Solved in 2 steps

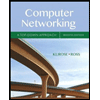
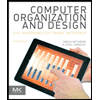
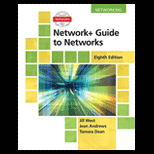
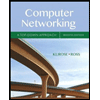
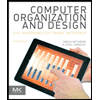
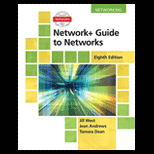
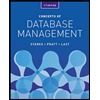
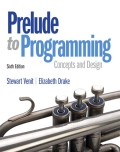
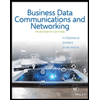