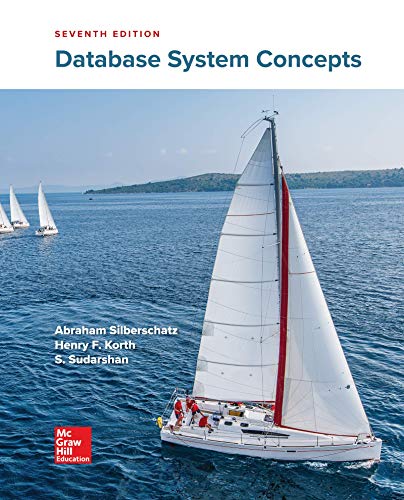
in python. Please include docstring, so I can understand each step to this program. Thanks.
you will import the json module.
Write a class named NeighborhoodPets that has methods for adding a pet, deleting a pet, searching for the owner of a pet, saving data to a JSON file, loading data from a JSON file, and getting a set of all pet species. It will only be loading JSON files that it has previously created, so the internal organization of the data is up to you.
- The init method takes no parameters and initializes all the data members, which must all be private.
- The add_pet method takes as parameters the name of the pet, the species of the pet, and the name of the pet's owner. If a pet has the same name as a pet that has already been added, then the function should raise a DuplicateNameError (you'll need to define this exception class).
- The delete_pet method takes as a parameter the name of the pet and deletes that pet.
- The get_owner method takes as a parameter the name of the pet and returns the name of its owner.
- The save_as_json method takes as a parameter the name of the file and saves it in JSON format with that name. You can assume the extension (if any) will be part of the provided name. You can organize your JSON file however you want.
- The read_json method takes as a parameter the name of the file to read and loads that file. This will replace all of the pets currently in memory.
- The get_all_species method takes no parameters and returns a Python set of the species of all pets.
For example, your class could be used like this:
If you implement a Pet class (which is a natural option, but not required), then when you save it, you'll need to translate the information into one of the built-in object types the json module recognizes, and translate it back the other way when you read it.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

this code is missing the duplicate name error. How can this be added?
this code is missing the duplicate name error. How can this be added?
- No written by hand solutionarrow_forwardWritten in Python with docstring please if applicable Thank youarrow_forwardFor this project you will use the file included in the attached zip file. It is a series of fictional exam records. You must create a Python application that reads this file, assigns a letter grade to each student based on the scale below, outputs the student name, grade, and letter grade to the screen, and outputs that same information into a comma separated values file. You must use functions, parameters, returns, loops, collections and conditions, and some kind of design (pseudocode or flowcharts). Naming and organization counts. Functions to consider: opening a file, closing a file, reading records, writing records, determining letter grade, and writing to console. Grade scale to use in the application: A: >95% A-: >91 and <95 B+: >87 and <91 B: >83 and <87 B-: >80 and <83 C+: >78 and <80 C: >75 and <78 D: >70 and <75 F: <70arrow_forward
- python: def pokehelp(poke_name, stat_min): """ Question 4 - API You are looking for a Pokemon that can help you with your programming homework. Since Porygon is made of code, you decide to look into the species. Using the given API, return a dictionary that returns the name of each of Porygon's stats with a base stat GREATER THAN the given number, mapped to their value. Do not hardcode Porygon's stats into the results! It is not the only test case. Base URL: https://pokeapi.co/ Endpoint: api/v2/pokemon/{poke_name} Args: Pokemon name (str), stat minimum (int) Returns: Dictonary of stats >>> pokehelp("porygon", 65) {'defense': 70, 'special-attack': 85, 'special-defense': 75} >>> pokehelp("koraidon", 100) {'attack': 135, 'defense': 115, 'speed': 135} """ # pprint(pokehelp("porygon", 65)) # pprint(pokehelp("koraidon", 100))arrow_forwardPython Please. and the data file is: 2121211143534722arrow_forwardhow to write a method that reads in any csv file and extracts the data from the csv file into header and the remaining data using the csv module, and stores them in instance variables. also how to write a code to handle potential errors with file content such as empty file and file not found in python?arrow_forward
- The goal for Lab06b is to use the provided Student class (ATTACHED IN IMAGE) and create an array of Student objects that are stored in a School object. This program uses a Student class that is provided and shown below. The class is placed in its own separate file and should not be altered. This program sequence started with Lab06a. It is a reminder that it is the same Student file used by Lab06a. This lab will add data processing to the earlier Lab06a. This program will continue with the Lab06b program that performs some data processing on the Student records. For this lab 10 Student objects need to be constructed and placed in a students array, which is stored in a School object. You actually did this already for Lab06a. You also need to complete the School constructor, addData method and toString Method, which were in Lab06a. Feel free to just copy them over. You need to complete three bubbleSort methods; one that sorts according to the student gpa., one for age and one for name.…arrow_forwardThank you very much for explaining the absyract concept, it was very helpful and easier to understanc after you explained it. Today we are asked to modify the GridWriter class by adding additional collection style functionality, to throw an exception in case of an error. The GridWriter class should get two new methods: public int size() should return the number of GridItems stored in the GridWriter public GridItem get(int index) should return the stored GridItems by index. Consider the following code. The first line creates a GridWriter object. Then two items are added to the GridWriter. The index of the items will be 0, and 1. Notice how the for loop uses the size and get methods to print out the areas of the two items GridWriter gw = new GridWriter(40, 50); gw.add(new MyCircle(10, 10, 9)); gw.add(new MyRectangle(40, 0, 10, 10)); for (int i = 0; i < gw.size(); i++) { System.out.println(gw.get(i).getArea()); } Once you have these two methods working you…arrow_forward/*TASK 5. Implement part 1 of the report. You need to read data from input.txt file, store it into array of items (called items) and write it in a formatted form to the output file. Code, debug and present it in the best possible format. Hint: It might be helpful to format the String coming from toString( ) method using String.format( ) method, which works similar to System.out.prinf. Hint2: You might have to close and delete existing output .doc or .txt file from its folder * public class YourUserName_hw7 { static Item [ ] items = new Item [200];//an array of Items static EdibleItem [ ] edibleItems;//an array of Edible Items static int countEdibleitems = 0;//count edible items static String pageHeader; //save the header //main( ) method runs the program public static void main(String[ ] args) throws FileNotFoundException { double sum = 0, average = 0; //Optional - create a new input file //CreateInput.createInputFile( );…arrow_forward
- in c++ codearrow_forwardAssume that you are designing a simple text editor (like Notepad) that supports inserting text, copy, paste and editing for any size file (including book-length files). Between the String objects and StringBuffer objects, which one is more appropriate and why? O String, because StringBuffer is read-only String, because it will execute more efficiently String, because only simple operations are needed StringBuffer, because it allows for changing the characters stored in the object StringBuffer, because it has copy and paste methodsarrow_forwardAssume that all source files are in the same package. Note that some lines may wrap, but are still considered on one line. Examine the code below. Recall that variables have four kinds of scope: static, instance, local, and block. For each variable listed below, identify its scope (static, instance, local, or block) and describe the scope using complete sentences. An example description is provided, after the code listing, for the ounces variable in the Beverage2 class. Please describe the scope for the following variables: The num variable in the Beverage2 class The price variable in the Beverage2 class The shots variable in the Espresso class The b variable in the TestBeverage2 class The j variable in the TestBeverage2 class public abstract class Beverage2 { private static int num; private int ounces; private double price; protected Beverage2(int ounces, double price) { this.ounces = ounces; this.price = price;…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
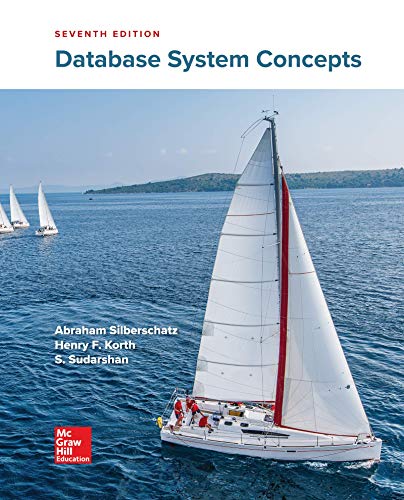
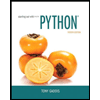
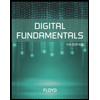
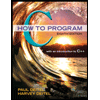
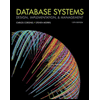
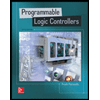