In Python, implement a hashing table data structure of size 20, which can cope with collisions. Thus, you must implement the closed collision system in its three modes: Linear scan Quadratic scan Double hashing scan. For halt use halt(k)= (k mod (m - 1)) + 1, where m is the size of the table. The data to be managed are students from a certain university who have a 5-digit registration number and it is unique. In addition, the student has a name, age and address. Create a .txt file with 10 students (one for each line) and have them loaded into your program. Build a menu of options where you can: - Enter a new student. For this option, that only allows entering the 5-digit license plate and that random data is automatically generated for the name, age and address. Return the position where it was entered and if it is not possible to enter it, print a message that explains why the entry was not obtained.
In Python, implement a hashing table data structure of size 20, which can cope with collisions. Thus, you must implement the closed collision system in its three modes:
Linear scan
Quadratic scan
Double hashing scan. For halt use halt(k)= (k mod (m - 1)) + 1, where m is the size of the table.
The data to be managed are students from a certain university who have a 5-digit registration number and it is unique. In addition, the student has a name, age and address. Create a .txt file with 10 students (one for each line) and have them loaded into your program. Build a menu of options where you can:
- Enter a new student. For this option, that only allows entering the 5-digit license plate and that random data is automatically generated for the name, age and address. Return the position where it was entered and if it is not possible to enter it, print a message that explains why the entry was not obtained.

Step by step
Solved in 2 steps

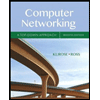
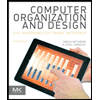
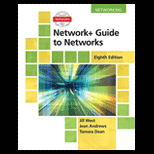
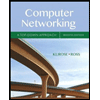
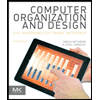
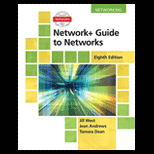
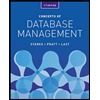
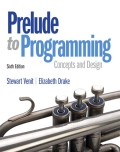
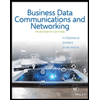