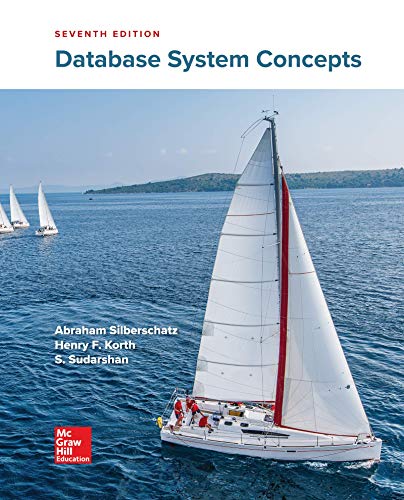
In early implementations of FORTRAN language, a compiler may choose to use static
allocation (i.e., allocation in the static area) for local variables and parameters, effectively arranging
for the variables of different invocations to share the same locations, and thereby avoiding
any run-time overhead for creation and destruction of stack frames. However, such an implemen-
tation changes the meaning of recursive function calls.
Provide a simple example and explain how its meaning changes under the “FORTRAN” semantics
as stated above, compared with standard semantics found in languages like C, Python, and Java.

1) Static allocation refers to the process of reserving memory for variables during compile-time. The memory allocation is determined before the program is executed and remains fixed throughout the program's runtime. This means that the size and type of data to be stored in the variable are known at compile-time.
2) In statically allocated memory, variables have fixed addresses that are determined at compile-time. This allocation strategy is commonly used for global variables and local variables with the static keyword in languages like C and C++. It is also used for arrays whose size is known at compile-time.
3) Dynamic allocation refers to the process of allocating memory for variables during runtime, as the program is executing. This allows for the creation of variables whose size and type may not be known until the program is actually running.
4) In dynamically allocated memory, variables are assigned memory addresses during program execution, and their size and characteristics can be changed as needed. Languages like C++ (with new and delete operators), C (with malloc() and free() functions), and Java (with the new keyword) support dynamic allocation.
Step by stepSolved in 4 steps

- code in C++arrow_forwardElaborate a C program of a circular static queue, where you can add an element, remove an element, show the queue, check if the queue is full, check if the queue is empty, and exit the program. Specify what is the structure of the queue, what are the function prototypes and what is the main function.arrow_forwardIn a programming language (Pascal), the declaration of a node in a singly linked list is shown in Figure P6.7(a). The list referred to for the problem is shown in Figure P6.7(b). Given P to be a pointer to a node, the instructions DATA(P) and LINK(P) referring to the DATA and LINK fields, respectively, of node P are equivalently represented by P↑. DATA and P↑. LINK in the programming language. What do the following commands do to the logical representation of the list T? i) ii) TYPE POINTER=NODE; NODE RECORD DATA: integer; LINK: POINTER END; VAR P, Q R: T POINTER (a) Declaration of a node in a singly linked list T 31 TE 57 12 (b) A singly linked list T iii) R₁.LINK: = Q iv) R₁.DATA: = P₁.DATA: Q₁.DATA + R₁.DATA Q: = P |R Figure P6.7. (a and b) Declaration of a node in a programming language and the logical representation of a singly linked list T Q.LINK.DATA + 10 91 Linked Lists 189arrow_forward
- In a concurrent environment, what precautions should be taken when using the Comparable interface to avoid race conditions and synchronization issues during sorting?arrow_forwardYour task for this assignment is to implement a stack data structure in C++. This may be accomplished by utilizing the C++ standard template library (STL) or by utilizing a user-defined class. 1. 2. 3. 4. Implement a stack data structure using C++. The program will be interactive. Data transactions will be entered as a batch of transactions in a text file at the command line using redirection. The result of each transaction will be displayed on the console. For example: $ prog3 < prog3.dat Each input transaction will contain an arithmetic expression in post-fix format. Assume that each operand and operation (+,-, *, /, %, ^) is a single character in each arithmetic expression without spacing. You may assume that each arithmetic expression is correctly formatted. However, your program should not attempt the following: a. Divide by zero with / or % ... Instead, your program should display an error message and then begin evaluating a new input transaction. b. Perform % with non-integer…arrow_forwardNonscalar types, such as arrays, records (structures), and variant records (unions) also contain information. The rules for information flow classes for these data types are built on the scalar types. Consider the array a: array 1 .. 100 of int; First, look at information flows out of an element a[i] of the array. In this case, information flows from a[i] and from i, the latter by virtue of the index indicating which element of the array to use. Information flows into a[i] affect only the value in a[i], and so do not affect the information in i. Thus, for information flows from a[i], the class involved is lub{ a[i], i }; for information flows into a[i], the class involved is a[i]. Above is the infromation flow security mechanism for arrays similarly write one for structures/Records. Below is the small info on Structures. The structure consists of several fields, each of which acts like a variable. Say you have a structure with two integer fields, x and y. You want to assign the value…arrow_forward
- Please help me with this Principles of programming language homework questionarrow_forwardwrite a recursive function in F#, named indexWiseMax, that takes two list of integers and outputs the index-wise maximum values of two lists. use pattern matching to receive the arguments, lst1, and lst2 and return a list that has the max element for each index of both lists. let rec indexWiseMax lst1 lst2 = match lst1, lst2 witharrow_forwardIn the study of formal languages state-transition diagrams are often usedto visualize changes in a machine’s configuration as it acts on input. To visualize amachine’s configuration think of its parts: Commonly a finite set of states, a finiteinput alphabet Σ, Perhaps storage devices (a stack, input/output tapes). The states arepictured as named circles sometimes decorated with symbols to denote special states,e.g. start and final states. Changes in configuration are denoted by labeled edges andperhaps changes in storage.1. Describe how edges are labeled and their meaning for finite state machines.2. Describe how edges are labeled and their meaning for pushdown automata.3. Describe how edges are labeled and their meaning for Turing machines.arrow_forward
- There have been reports of hanging and wild pointers being an issue with the pointers? provide a good illustrationarrow_forwardI have to create a small library in the C programming language to do Minifloat sensible representation and stay consistent throughout a library.The internal representation (e.g. a struct) does not have to match the external representation (e.g. just a binary pattern, or a pointer to an abstract data type). do not return a pointer to a local static variables in a function. Instead, allocate on the heap with malloc and then return the pointer to that. The following pictrures are more of the requirements.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
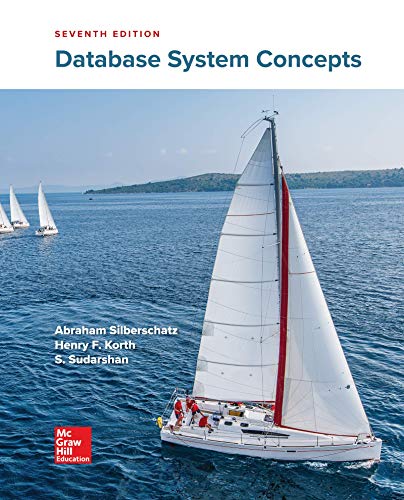
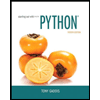
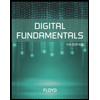
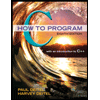
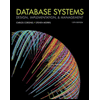
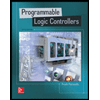