Can someone help me with this in C++? I have to implement a stack linked list to get these tests on my driver.cpp to work. //////////////////////////////////////////////////////////////////////// driver.cpp //////////////////////////////////////////////////////////////////////// #include #include #include "stackLL.h" #include "queueLL.h" #include "priorityQueueLL.h" using namespace std; int main() { /////////////Test code for stack /////////////// ///////////// Do not modify anything below /////////////// stackLL stk; stk.push(5); stk.push(13); stk.push(7); stk.push(3); stk.push(2); stk.push(11); cout << "Popping: " << stk.pop() << endl; cout << "Popping: " << stk.pop() << endl; stk.push(17); stk.push(19); stk.push(23); while (!stk.empty()) { cout << "Popping: " << stk.pop() << endl; } // output order: 11,2,23,19,17,3,7,13,5 stackLL stkx; stk.push(5); stk.push(10); stk.push(15); stk.push(20); stk.push(25); stk.push(30); stk.insertAt(-100, 3); stk.insertAt(-200, 7); stk.insertAt(-300, 0); //output order: -300,30,25,20,-100,15,10,5,-200 while (!stk.empty()) cout << "Popping: " << stk.pop() << endl; //////////////////////////////////////////////////////////////////////// stackLL.h //////////////////////////////////////////////////////////////////////// class stackLL { private: class node { public: //put what you need in here }; node * top; public: stackLL() {} //Take care of memory leaks... ~stackLL() {} //return true if empty, false if not bool empty() {} //add item to top of stack void push(int x) {} //remove and return top item from stack int pop() {} //add item x to stack, but insert it //right after the current ith item from the top //(and before the i+1 item). void insertAt(int x, int i) {} };
Can someone help me with this in C++? I have to implement a stack linked list to get these tests on my driver.cpp to work.
////////////////////////////////////////////////////////////////////////
driver.cpp
////////////////////////////////////////////////////////////////////////
#include <iostream>
#include <string>
#include "stackLL.h"
#include "queueLL.h"
#include "priorityQueueLL.h"
using namespace std;
int main()
{
/////////////Test code for stack ///////////////
///////////// Do not modify anything below ///////////////
stackLL stk;
stk.push(5);
stk.push(13);
stk.push(7);
stk.push(3);
stk.push(2);
stk.push(11);
cout << "Popping: " << stk.pop() << endl;
cout << "Popping: " << stk.pop() << endl;
stk.push(17);
stk.push(19);
stk.push(23);
while (!stk.empty())
{
cout << "Popping: " << stk.pop() << endl;
}
// output order: 11,2,23,19,17,3,7,13,5
stackLL stkx;
stk.push(5);
stk.push(10);
stk.push(15);
stk.push(20);
stk.push(25);
stk.push(30);
stk.insertAt(-100, 3);
stk.insertAt(-200, 7);
stk.insertAt(-300, 0);
//output order: -300,30,25,20,-100,15,10,5,-200
while (!stk.empty())
cout << "Popping: " << stk.pop() << endl;
////////////////////////////////////////////////////////////////////////
stackLL.h
////////////////////////////////////////////////////////////////////////
class stackLL
{
private:
class node
{
public:
//put what you need in here
};
node * top;
public:
stackLL()
{}
//Take care of memory leaks...
~stackLL()
{}
//return true if empty, false if not
bool empty()
{}
//add item to top of stack
void push(int x)
{}
//remove and return top item from stack
int pop()
{}
//add item x to stack, but insert it
//right after the current ith item from the top
//(and before the i+1 item).
void insertAt(int x, int i)
{}
};

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

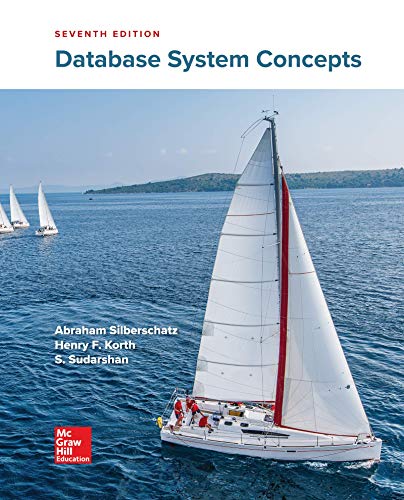
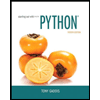
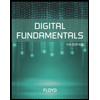
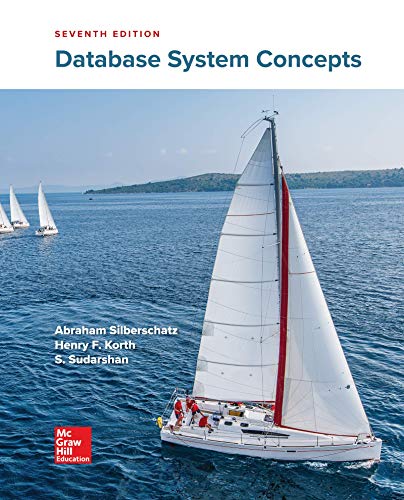
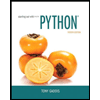
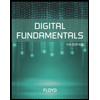
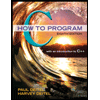
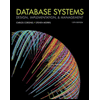
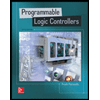