In c++: Bracket Matcher Given a text file, your program will determine if all the parentheses, curly braces, and square brackets match, and are nested appropriately. Your program should work for mathematical formulas and most computer programs. Your program should read in the characters from the file, one at a time, but ignore all characters except for the following: { } ( ) [ ] Your program should use the provided IntStack implementation (IntStack.h and IntStack.cpp). The general algorithm is to use the stack to store the opening unmatched brackets as they are encountered in the input. When a closing bracket is encountered, check it against the one on top of the stack (pop it off)--make sure it matches. When you are finished, if all the brackets match then there should be no unmatched brackets left on the stack. (Note: it might be easier to store the expected closing character on the stack when an opening bracket is encountered; this simplifies the matching when a closing bracket is encountered). Input/Output: Your program must prompt the user to enter a filename. It should then try to open the file and then check it make sure the brackets all match appropriately. If they all match, the program should output a message saying so. If not, the program should output an appropriate error message as soon as the first error is encountered and then exit the program. There are three types of errors that can happen (and they can happen with any kind of bracket): missing } : if you reach the end of the file, and there is an opening { that was never matched, like: int main () { x[size]=10; expected } but found ) : this is a wrong closing bracket, like: {x[i]=10;)... unmatched } : this occurs if there is a closing bracket but not an opening bracket (not even one of the wrong kind), like: int main () { x[i]=10; } }... See the test cases below for exact wording of the prompt and error messages. NOTES: The stack is an int stack but you will probably want to put characters on the stack. You can push a character directly on the int stack (it will automatically be converted to an int). When you pop I recommend popping directly into a char variable (this will automatically convert the int back into a character). Beware of stack underflow! Do NOT try to pop an empty stack! If you get an error message like this when you run your program: Assertion failed: (!isEmpty()) then you are popping an empty stack: your program should prevent this and output its own appropriate error message instead.
In c++:
Bracket Matcher
Given a text file, your program will determine if all the parentheses, curly braces, and square brackets match, and are nested appropriately. Your program should work for mathematical formulas and most computer programs.
Your program should read in the characters from the file, one at a time, but ignore all characters except for the following: { } ( ) [ ]
Your program should use the provided IntStack implementation (IntStack.h and IntStack.cpp).
The general
Input/Output:
Your program must prompt the user to enter a filename. It should then try to open the file and then check it make sure the brackets all match appropriately. If they all match, the program should output a message saying so. If not, the program should output an appropriate error message as soon as the first error is encountered and then exit the program.
There are three types of errors that can happen (and they can happen with any kind of bracket):
- missing } : if you reach the end of the file, and there is an opening { that was never matched, like: int main () { x[size]=10;
- expected } but found ) : this is a wrong closing bracket, like: {x[i]=10;)...
- unmatched } : this occurs if there is a closing bracket but not an opening bracket (not even one of the wrong kind), like: int main () { x[i]=10; } }...
See the test cases below for exact wording of the prompt and error messages.
NOTES:
- The stack is an int stack but you will probably want to put characters on the stack. You can push a character directly on the int stack (it will automatically be converted to an int). When you pop I recommend popping directly into a char variable (this will automatically convert the int back into a character).
- Beware of stack underflow! Do NOT try to pop an empty stack! If you get an error message like this when you run your program: Assertion failed: (!isEmpty()) then you are popping an empty stack: your program should prevent this and output its own appropriate error message instead.

Step by step
Solved in 2 steps with 11 images

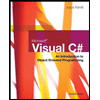
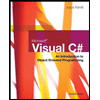