import sys import json class Process: def __init__(self, name, duration, arrival_time, io_frequency): self.name = name self.duration = duration self.arrival_time = arrival_time self.io_frequency = io_frequency def schedule_processes(processes): # Implement your scheduling algorithm here schedule = [] # Sort processes based on arrival time processes.sort(key=lambda x: x.arrival_time) current_time = 0 for proc in processes: while current_time < proc.arrival_time: current_time += 1 schedule.append(proc.name) for time in range(proc.duration): current_time += 1 # Check for IO interruptions if proc.io_frequency > 0 and time > 0 and time % proc.io_frequency == 0 and time < proc.duration - 1: schedule.append(f"!{proc.name}") return ' '.join(schedule) def main(): # Check if the correct number of arguments is provided if len(sys.argv) != 2: return 1 input_file_name = f"Process_List/{config['dataset']}/{sys.argv[1]}" # Define the number of processes num_processes = 0 data_set = [] # Open the file for reading try: with open(input_file_name, "r") as file: # Read the number of processes from the file num_processes = int(file.readline().strip()) # Read process data from the file and populate the data_set list for _ in range(num_processes): line = file.readline().strip() name, duration, arrival_time, io_frequency = line.split(',') process = Process(name, int(duration), int(arrival_time), int(io_frequency)) data_set.append(process) except FileNotFoundError: print("Error opening the file.") return 1 # Get the schedule based on the implemented algorithm output = schedule_processes(data_set) # Open a file for writing try: output_path = f"Schedulers/template/{config['dataset']}/template_out_{sys.argv[1].split('_')[1]}" with open(output_path, "w") as output_file: # Write the final result to the output file output_file.write(output) except IOError: print("Error opening the output file.") return 1 return 0 if __name__ == "__main__": exit_code = main() sys.exit(exit_code) fix this code, the scheduler does not print out the correct number of processes on the followin input 34 AB,30,0,0 AC,48,10,0 BJ,41,12,0 AJ,50,15,0 AF,33,16,15 AI,49,22,15 AD,35,33,0 AY,47,61,22 AN,32,64,11 AW,32,87,0 AE,34,94,17 AT,43,97,14 AX,43,98,5 AH,44,128,0 AG,41,131,0 AM,32,164,0 AK,45,205,22 AL,33,251,3 BA,31,294,12 AO,42,305,15 AS,43,347,13 AZ,33,420,14 AP,46,440,0 AR,43,462,0 BF,38,463,18 BH,49,547,0 BI,35,559,11 AQ,33,564,0 BC,33,591,0 AU,40,681,12 AV,34,703,3 BE,33,717,4 BD,34,987,0 BG,49,1140,0 it prints 99 instead of 1328
import sys import json class Process: def __init__(self, name, duration, arrival_time, io_frequency): self.name = name self.duration = duration self.arrival_time = arrival_time self.io_frequency = io_frequency def schedule_processes(processes): # Implement your scheduling algorithm here schedule = [] # Sort processes based on arrival time processes.sort(key=lambda x: x.arrival_time) current_time = 0 for proc in processes: while current_time < proc.arrival_time: current_time += 1 schedule.append(proc.name) for time in range(proc.duration): current_time += 1 # Check for IO interruptions if proc.io_frequency > 0 and time > 0 and time % proc.io_frequency == 0 and time < proc.duration - 1: schedule.append(f"!{proc.name}") return ' '.join(schedule) def main(): # Check if the correct number of arguments is provided if len(sys.argv) != 2: return 1 input_file_name = f"Process_List/{config['dataset']}/{sys.argv[1]}" # Define the number of processes num_processes = 0 data_set = [] # Open the file for reading try: with open(input_file_name, "r") as file: # Read the number of processes from the file num_processes = int(file.readline().strip()) # Read process data from the file and populate the data_set list for _ in range(num_processes): line = file.readline().strip() name, duration, arrival_time, io_frequency = line.split(',') process = Process(name, int(duration), int(arrival_time), int(io_frequency)) data_set.append(process) except FileNotFoundError: print("Error opening the file.") return 1 # Get the schedule based on the implemented algorithm output = schedule_processes(data_set) # Open a file for writing try: output_path = f"Schedulers/template/{config['dataset']}/template_out_{sys.argv[1].split('_')[1]}" with open(output_path, "w") as output_file: # Write the final result to the output file output_file.write(output) except IOError: print("Error opening the output file.") return 1 return 0 if __name__ == "__main__": exit_code = main() sys.exit(exit_code) fix this code, the scheduler does not print out the correct number of processes on the followin input 34 AB,30,0,0 AC,48,10,0 BJ,41,12,0 AJ,50,15,0 AF,33,16,15 AI,49,22,15 AD,35,33,0 AY,47,61,22 AN,32,64,11 AW,32,87,0 AE,34,94,17 AT,43,97,14 AX,43,98,5 AH,44,128,0 AG,41,131,0 AM,32,164,0 AK,45,205,22 AL,33,251,3 BA,31,294,12 AO,42,305,15 AS,43,347,13 AZ,33,420,14 AP,46,440,0 AR,43,462,0 BF,38,463,18 BH,49,547,0 BI,35,559,11 AQ,33,564,0 BC,33,591,0 AU,40,681,12 AV,34,703,3 BE,33,717,4 BD,34,987,0 BG,49,1140,0 it prints 99 instead of 1328
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
import sys
import json
class Process:
def __init__(self, name, duration, arrival_time, io_frequency):
self.name = name
self.duration = duration
self.arrival_time = arrival_time
self.io_frequency = io_frequency
def schedule_processes(processes):
# Implement your scheduling algorithm here
schedule = []
# Sort processes based on arrival time
processes.sort(key=lambda x: x.arrival_time)
current_time = 0
for proc in processes:
while current_time < proc.arrival_time:
current_time += 1
schedule.append(proc.name)
for time in range(proc.duration):
current_time += 1
# Check for IO interruptions
if proc.io_frequency > 0 and time > 0 and time % proc.io_frequency == 0 and time < proc.duration - 1:
schedule.append(f"!{proc.name}")
return ' '.join(schedule)
def main():
# Check if the correct number of arguments is provided
if len(sys.argv) != 2:
return 1
input_file_name = f"Process_List/{config['dataset']}/{sys.argv[1]}"
# Define the number of processes
num_processes = 0
data_set = []
# Open the file for reading
try:
with open(input_file_name, "r") as file:
# Read the number of processes from the file
num_processes = int(file.readline().strip())
# Read process data from the file and populate the data_set list
for _ in range(num_processes):
line = file.readline().strip()
name, duration, arrival_time, io_frequency = line.split(',')
process = Process(name, int(duration), int(arrival_time), int(io_frequency))
data_set.append(process)
except FileNotFoundError:
print("Error opening the file.")
return 1
# Get the schedule based on the implemented algorithm
output = schedule_processes(data_set)
# Open a file for writing
try:
output_path = f"Schedulers/template/{config['dataset']}/template_out_{sys.argv[1].split('_')[1]}"
with open(output_path, "w") as output_file:
# Write the final result to the output file
output_file.write(output)
except IOError:
print("Error opening the output file.")
return 1
return 0
if __name__ == "__main__":
exit_code = main()
sys.exit(exit_code)
fix this code, the scheduler does not print out the correct number of processes on the followin input
34
AB,30,0,0
AC,48,10,0
BJ,41,12,0
AJ,50,15,0
AF,33,16,15
AI,49,22,15
AD,35,33,0
AY,47,61,22
AN,32,64,11
AW,32,87,0
AE,34,94,17
AT,43,97,14
AX,43,98,5
AH,44,128,0
AG,41,131,0
AM,32,164,0
AK,45,205,22
AL,33,251,3
BA,31,294,12
AO,42,305,15
AS,43,347,13
AZ,33,420,14
AP,46,440,0
AR,43,462,0
BF,38,463,18
BH,49,547,0
BI,35,559,11
AQ,33,564,0
BC,33,591,0
AU,40,681,12
AV,34,703,3
BE,33,717,4
BD,34,987,0
BG,49,1140,0
AB,30,0,0
AC,48,10,0
BJ,41,12,0
AJ,50,15,0
AF,33,16,15
AI,49,22,15
AD,35,33,0
AY,47,61,22
AN,32,64,11
AW,32,87,0
AE,34,94,17
AT,43,97,14
AX,43,98,5
AH,44,128,0
AG,41,131,0
AM,32,164,0
AK,45,205,22
AL,33,251,3
BA,31,294,12
AO,42,305,15
AS,43,347,13
AZ,33,420,14
AP,46,440,0
AR,43,462,0
BF,38,463,18
BH,49,547,0
BI,35,559,11
AQ,33,564,0
BC,33,591,0
AU,40,681,12
AV,34,703,3
BE,33,717,4
BD,34,987,0
BG,49,1140,0
it prints 99 instead of 1328
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
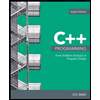
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
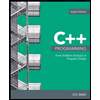
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning