Design and implement a service that simulates PHP loops. Each of the three loop variants should be encapsulated in an object. The service can be controlled via a parameter and execute three different simulations. The result is returned as JSON. The input is an array consisting of the letters $characters = [A-Z]. -The For loop should store all even letters in an array. -The Foreach loop simulation is to create a backward sorted array by For loop, i.e. [Z-A] . -The While loop should write all characters into an array until the desired character is found. Interface: -GET Parameter String: loopType ( possible values: REVERSE, EVEN, UNTIL ) -GET parameter String: until (up to which character) Output: JSON Object: {loopName: , result: }
Design and implement a service that simulates PHP loops. Each of the three loop variants should be encapsulated in an object. The service can be controlled via a parameter and execute three different simulations. The result is returned as JSON.
The input is an array consisting of the letters $characters = [A-Z].
-The For loop should store all even letters in an array.
-The Foreach loop simulation is to create a backward sorted array by For loop, i.e. [Z-A] .
-The While loop should write all characters into an array until the desired character is found.
Interface:
-GET Parameter String: loopType ( possible values: REVERSE, EVEN, UNTIL )
-GET parameter String: until (up to which character)
Output:
JSON Object: {loopName: <string>, result: <array> }

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

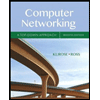
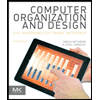
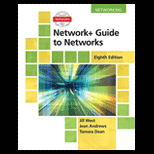
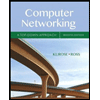
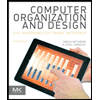
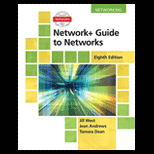
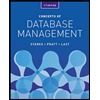
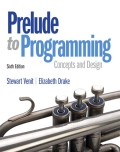
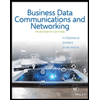