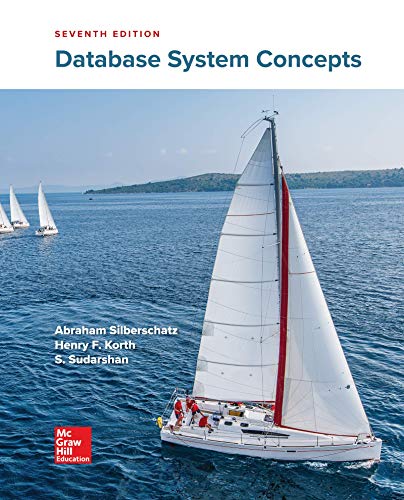
Concept explainers
I need to provide an implementation for the InternPhone constructor and provide an implementation for the InternPhone Write(). Attached is the below code I wrote earlier with the InterPhone and Write() constructor.
#include <iostream>
using namespace std;
enum PhoneType { HOME, OFFICE, CELL };
class Phone {
private:
int areaCode;
int number;
PhoneType type;
public:
Phone(int newAreaCode, int newNumber, PhoneType newType);
void Write();
};
Phone::Phone(int newAreaCode, int newNumber, PhoneType newType) {
areaCode = newAreaCode;
newNumber = newNumber;
type = newType;
}
void Phone::Write() {
cout << "Area code : " << areaCode << endl;
cout << "Number : " << number << endl;
cout << "Phone type : " << type << endl;
}
class internPhone :public Phone {
private:
int countrycode;
public:
internPhone(int newCountryCode, int newareaCode, int newNumber, PhoneType newType);
void write();
};
internPhone::internPhone(int newCountryCode, int newareaCode, int newNumber, PhoneType newType) :Phone(newareaCode, newNumber, newType) {
countrycode = newCountryCode;
}
void internPhone::write() {
cout << "Country Code :" << countrycode << endl;
Phone::Write();
}
int main()
{
internPhone ip(+1, 615, 2156584, OFFICE);
ip.write();
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Consider the Speaker interface shown below: public interface Speaker{ public void speak();} For this problem, you will create 2 classes Human and Dog, both of which will implement the Speaker interface. The classes will not contain any properties; they will only implement the speak method, as dictated by the Speaker interface. The speak method will simply print a message in the "native language" of that speaker: For a Human, the speak method will print "Hello!" For a Dog, the speak method will print "Bark!"arrow_forwardDesign ADT for a cave and a cave system. An archeologist should be able to add a newly discovered cave to a cave system and to connect two caves together by a tunnel. Duplicate caves—based on GPS coordinates—are not permitted. Archeologists should also be able to list the caves in a given cave system. Specify each ADT operation by stating its purpose, describing its parameters, and writing a pseudocode version of its header. Then Java interface for a cave's methods and one for the methods of a cave system. Include javadoc-style comments in your code. Java programarrow_forwardI'm learning java in college and I missed one homework assignment. Since then it has been difficult to keep up with homework because they were based on the one I missed. Here's the prompt: Design a class Phone with one data member as long phoneNumber. Add constructors, get/set methods to class Phone. Then design a class InternationalPhone as subclass of class Phone, with one extra data member as int countryCode. Add constructors, get/set methods to class InternationalPhone. Write a main program to test these two classes. (We use netbeans btw) I can't get any credit for this so there's no rush but I would be very happy to recieve an answer before the end of the semester. Thank you in advance :)arrow_forward
- B elow for each class you find a UML and description of the public interface. Implementing the public interface as described is madatory. There's freedom on how to implement these classes.The private properties and private methods are under your control.. There are multiple ways of implementing all these classes. Feel free to add private properties and methods. For each object, it's mandatory to create a header file (.h), implementation file (.cpp) and a driver. Blank files are included. The header should have the class definition in it. The implementation file should contain the implementations of the methods laid out in the header fine. And finally the Driver should test/demonstrate all the features of the class. It's best to develop the driver as the class is being written. Check each section to see if there are added additional requirements for the driver. Two test suites are included so that work can be checked. It's important to implement the drivers to test and demonstrate…arrow_forwardIn C++ write the definition of the class professorType, inherited from the class personType, defined in Chapter 10, with an additional data member to store a professor’s highest degree. Add appropriate constructors and member functions to initialize, access, and manipulate the data members. Write a test program to initialize, access, and manipulate the data members. For example, the mathProfessor holds a Doctorate degree.arrow_forwardIn the class diagram below we have a parking charge class for an object-oriented parking system that is to be designed using java. Briefly explain any implementation decisions and the reasoning behind those without writing the complete code. N.B explain how the implementation will proceed instead of writing codearrow_forward
- javascript Need help defining a function frequencyAnalysis that accepts a string of lower-case letters as a parameter. frequencyAnalysis should return an object containing the amount of times each letter appeared in the string. example frequencyAnalysis('abca'); // => {a: 2, b: 1, c: 1}arrow_forwardImplement a nested class composition relationship between any two class types from the following list: Advisor Вook Classroom Department Friend Grade School Student Teacher Tutor Write all necessary code for both classes to demonstrate a nested composition relationship including the following: a. one encapsulated data member for each class b. inline default constructor using constructor delegation for each class c. inline one-parameter constructor for each class d. inline accessors for all data members e. inline mutators for all data membersarrow_forward3. Design a user-defined class called Course. Each course object will hold data about a course. The data includes courseID, courseName, credits and instructorName. Define the instance variables required of the appropriate type. Provide only get methods along with toString() and equals() methods. Design another user-defined class called Student. Each student object will hold data on name, GPA and courseList. Make sure to define courseList as an ArrayList holding references to Course objects Implement a constructor that will take values to initialize name, and gpa while courseList is initialized to an ArrayList() object. Provide the following get methods: getStudent ID ( ), getName(), getGPA (), get Gender(), and get CourseList() Note: the getCouresList() method will return a copy of the coureList arraylist with copies of course objects. Provide the following set methods: setName(), setGPA (), setGender() Another method: addCourse () that will take a Course object and add it to the…arrow_forward
- Write a graphical application that contains a class named RV whose objects are the recreational vehicle designed and digitized as described in Knowledge Exercises 20 and 21 (you can design your version as well. Simple car shape is will be appreciated. The class’s private data members should be the vehicle’s body color and (x, y) location. a) Give the UML diagram for the class. It should include a three-parameter constructor, a toString method, a method to input the values of all of an object’s data members, and a show method to draw the RV at its current (x, y) location. b) Progressively implement and test the RV class by adding a method and verifying it before adding the next method. A good order to add the methods to the class is the three-parameter constructor, followed by the toString method, the show method, and finally the inputmethod. The client code should create an RV object using the three-parameter constructor to test all of the methods as they are progressively added to the…arrow_forwardIn C++ Create a new project named lab9_1 . You will need to implement a Course class. Here is its UML diagram: Course - department : string- course_num : string- section : int- num_students : int- is_full : bool + Course()+ Course(string, string, int, int)+ setDepartment(string) : void+ setNumber(string) : void+ setSection(int) : void+ setStudents(int) : void+ getDepartment() const : string+ getNumber() const : string+ getSection() const : int+ getStudents() const : int+ print() const : void Create a sample file to read from: CSS 2A 1111 35 Additional information: The Course class has two constructors. Make sure you have default values for your default constructor. Each course maxes out at 40 students. Therefore, you need to make sure that there aren’t more than 40 students in a Course. You can choose how you handle situations where more than 40 students are added. Additionally, you should automatically set is_full to false or true, based on the number of…arrow_forwardIn C++ Create a new project named lab8_1. You will be implementing two classes: A Book class, and a Bookshelf class. The Bookshelf has a Book object (actually 3 of them). You will be able to choose what Book to place in each Bookshelf. Here are their UML diagrams Book class UML Book - author : string- title : string- id : int- count : static int + Book()+ Book(string, string)+ setAuthor(string) : void+ setTitle(string) : void+ print() : void+ setID() : void And the Bookshelf class UML Bookshelf - book1 : Book- book2 : Book- book3 : Book + Bookshelf()+ Bookshelf(Book, Book, Book)+ setBook1(Book) : void+ setBook2(Book) : void+ setBook3(Book) : void+ print() : void Some additional information: The Book class also has two constructors, which again offer the option of declaring a Book object with an author and title, or using the default constructor to set the author and title later, via the setters . The Book class will have a static member variable…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
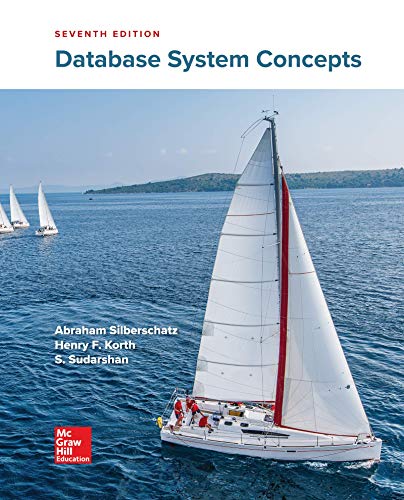
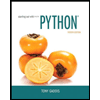
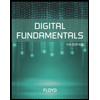
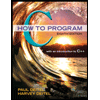
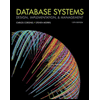
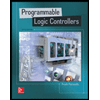