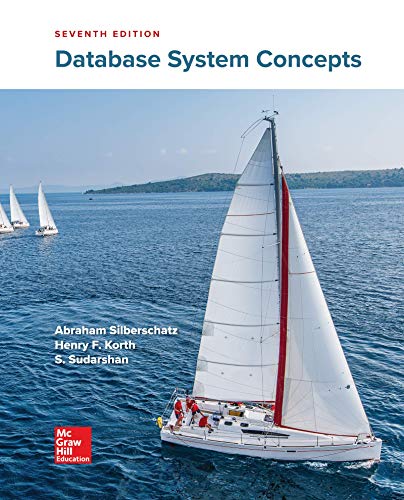
Implement the Solver class. In doing so, you are allowed to define other classes to help you (as well as use “built-in” Java classes or the book’s classes). The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of booleans and returns a char array containing a minimal sequence of moves that will lead to the solved board (all the cells around the edges being filled). The board configuration is passed in as a 5-by-5 boolean array of Booleans with exactly 16 true cells (filled) and 9 false cells (empty). The solve method then returns an array of characters representing a minimal sequence of moves that solves the puzzle. In other words, if the characters from the returned array are used in order as input to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. Furthermore, the solution must be minimal in the sense that there are no solutions that use fewer moves (although there could be other solutions that use the same number of moves). If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. The class also has a no argument constructor that will be called to construct/initialize the solver object. (There are perfectly valid solutions to the project where the constructor does absolutely nothing, but there are also solutions where some preprocessing work done in the constructor allows the solve method to be much faster.)

Step by stepSolved in 6 steps with 4 images

- Implement the Solver class. In doing so, you are allowed to define other classes to help you (as well as use “built-in” Java classes or the book’s classes). The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of booleans and returns a char array containing a minimal sequence of moves that will lead to the solved board (all the cells around the edges being filled). The board configuration is passed in as a 5-by-5 boolean array of Booleans with exactly 16 true cells (filled) and 9 false cells (empty). The solve method then returns an array of characters representing a minimal sequence of moves that solves the puzzle. In other words, if the characters from the returned array are used in order as input to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. Furthermore, the solution must be minimal in the sense that there are no solutions…arrow_forwardBased on the information in the screenshots, please answer the following question below. The language used is Java and please provide the code for it with the explanation. Create a testing class named “PlanTest”. Under this class, there needs to be another static method besides the main method: You will need to create a static method named “prerequisiteGenerator” under “PlanTest”, which return a random 2D array containing prerequisite pairs (e.g., {{1, 3}, {2, 3}, {4, 2}}). The value range of a random integer is [0, 10] (inclusive). This method will accept an integer parameter, which specifies the length of the returned array: int[][] pre = prerequisiteGenerator(3); // possibly {{1, 3}, {2, 3}, {4, 2}} Under the main method, you will need to use prerequisiteGenerator to generate random prerequisite pair lists, and perform tests on plan method at least three examples by printing proper messages. Given 4 courses and prerequisites as [[1,0], [2,0], [3,1]] It is possible to take all…arrow_forwardThis is in Java. The assignement is to create a class. I am confused on how to write de code for an array that is up to 5. Also I am confused regarding how to meet the requirements of the constructor. This is what I have. public class InventoryOnShelf { //fields private int[] itemOnShelfList []; private int size; public InventoryOnShelf() { }arrow_forward
- Java Problem Create an object of type FitnessExperiment that stores an array ofStepsFitnessTracker, DistanceFitnessTracker, andHeartRateFitnessTracker objects. In the FitnessExperiment class Add to your FitnessExperimentclass a method called getTotalDistance() that calculates the total distance walked by all theobjects stored in your FitnessExperiment. appropriately both values (total number of steps and total distance walked in that experiment) usingthe printExperimentDetails() method. FitnessExperiemnt.java public class FitnessExperiment {FitnessTracker[] fitnessTrackers;public static void main(String[] args) {FitnessTracker[] trackers ={ new StepsFitnessTracker("steps", new Steps(230)),new StepsFitnessTracker("steps2", new Steps(150)),new StepsFitnessTracker("steps2", new Steps(150)),new HeartRateFitnessTracker("hr", new HeartRate(80)),new HeartRateFitnessTracker("hr", new HeartRate(80)),new DistanceFitnessTracker("dist1", new Distance(1000)),new DistanceFitnessTracker("dist2",…arrow_forwardJava with screen shot pleasearrow_forwardHi, can i please get assistance with the following Java class below, where there is "TO DO:" I would like the task to be done please. Add anything relevant if needed only. The author class and the book class description is given. public class Book {private String title;private double averageRating;private String ISBN;private int numPages;// TO DO: insert an appropriate collection for associating Authors and initialise within constructors public Book(){} public Book(String title, double averageRating, String isbn, int numPages){ this.title = title;this.averageRating = averageRating;this.ISBN = isbn;this.numPages = numPages;}//accessorspublic String getTitle(){return title;}public double getAverageRating(){return averageRating;}public String getISBN(){return ISBN;}public int getNumPages(){return numPages;}// TO DO: provide an accessor for the collection on line 9 public String getAuthorList(){String authorList ="";// TO DO: insert code to return all author names separated by commas if…arrow_forward
- In Java, please. Complete the Course class by implementing the findStudentHighestGpa() method, which returns the Student object with the highest GPA in the course. Assume that no two students have the same highest GPA. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. (Hint: getGPA() returns a student's GPA.) Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Nguyen 3.5 Brenda Stern 2.0 Lynda Robison 3.2 Sonya King 3.9 the output is: Top student: Sonya King (GPA: 3.9) LabProgram.java import java.util.Scanner; public class LabProgram { public static void main(String args[]) { Scanner scnr = new Scanner(System.in); Course course = new Course(); int…arrow_forwardCan you implement an interface in your class? A solution is placed in the "solution" section to help you, but we would suggest you try to solve it on your own first. Problem Statement# You are given an interface Addition which contains a method signature int add(int num1, int num2). You need to write a class called Calculator which implements the Addition interface. The add(int, int) method takes two integers and returns their sum. Input# Calls the add(int, int) method by passing num1 and num2. Output# Returns the addition of num1 and num2.arrow_forwardIN JAVA. Any help is appreciated! Thank you! PART 1 : Automobiles Create a data class named Automobile that implements the Comparable interface. Give the class data fields for make, model, year, and price. Then add a constructor, all getters, a toString method that shows all attribute values, and implement Comparable by using the year as the criterion for comparing instances. Write a program named TestAutos that creates an ArrayList of five or six Automobiles. Use a for loop to display the elements in the ArrayList. Sort the Arraylist of autos by year with Collections.sort(). Finally, use a foreach loop to display the ArrayList sorted by year.arrow_forward
- Can you please help me with this, its in java. Thank you. Write classes in an inheritance hierarchy Implement a polymorphic method Create an ArrayList object Use ArrayList methods For this, please design and write a Java program to keep track of various menu items. Your program will store, display and modify salads, sandwiches and frozen yogurts. Present the user with the following menu options: Actions: 1) Add a salad2) Add a sandwich3) Add a frozen yogurt4) Display an item5) Display all items6) Add a topping to an item9) QuitIf the user does not enter one of these options, then display:Sorry, <NUMBER> is not a valid option.Where <NUMBER> is the user's input. All menu items have the following: Name (String) Price (double) Topping (StringBuilder or StringBuffer of comma separated values) In addition to everything that a menu item has, a main dish (salad or sandwich) also has: Side (String) A salad also has: Dressing (String) A sandwich also has: Bread type…arrow_forwardImplement the Solver class. The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of bytes and returns a byte array containing a minimal sequence of moves that will lead to the solved board. The 2D array of bytes is “triangular” and represents a valid board configuration. Namely, the 2D array has 5 rows (0 – 4) and the size of every row is 1 more than its index.The array contains one 0, five 1s, four 2s and five 3s. The solve method then returns an array of bytes representing a minimal sequence of moves that solves the puzzle. In other words, if the numbers from the returned array are used in order as inputs to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. import java.util.Arrays;…arrow_forwardAre there obvious improvements that could be made here with respect the software design for Squares and Circles? What programming constructs were you familiar with, and which did you need to look up? Assume we used a separate array for Squares and for Circles rather than one unifying Object array. How would this complicate the task of adding a new Shape (say, a Triangle) to our ObjectList class?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
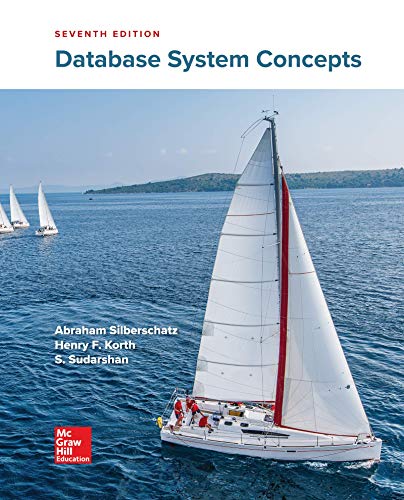
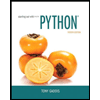
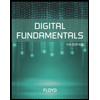
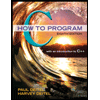
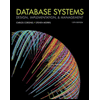
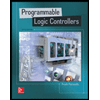