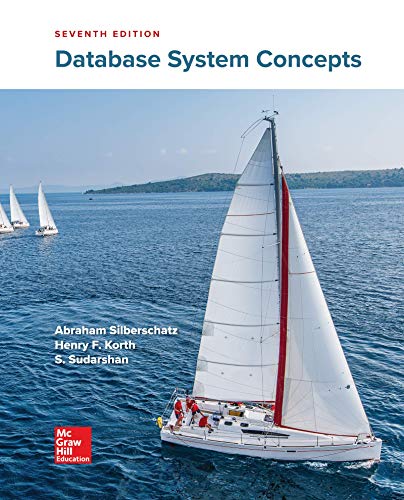
Java Problem
Create an object of type FitnessExperiment that stores an array of
StepsFitnessTracker, DistanceFitnessTracker, and
HeartRateFitnessTracker objects. In the FitnessExperiment class
Add to your FitnessExperiment
class a method called getTotalDistance() that calculates the total distance walked by all the
objects stored in your FitnessExperiment.
appropriately both values (total number of steps and total distance walked in that experiment) using
the printExperimentDetails() method.
FitnessExperiemnt.java
public class FitnessExperiment {
FitnessTracker[] fitnessTrackers;
public static void main(String[] args) {
FitnessTracker[] trackers =
{ new StepsFitnessTracker("steps", new Steps(230)),
new StepsFitnessTracker("steps2", new Steps(150)),
new StepsFitnessTracker("steps2", new Steps(150)),
new HeartRateFitnessTracker("hr", new HeartRate(80)),
new HeartRateFitnessTracker("hr", new HeartRate(80)),
new DistanceFitnessTracker("dist1", new Distance(1000)),
new DistanceFitnessTracker("dist2", new Distance(2430)),
new DistanceFitnessTracker("dist2", new Distance(2430))
};
FitnessExperiment fe = new FitnessExperiment(trackers);
fe.printExperimentDetails();
}
// Constructor
public FitnessExperiment(FitnessTracker[] fitnessTrackers) {
this.fitnessTrackers = fitnessTrackers;
}
// Methods to implement:
// Implementation hint: Should it use the corresponding toString() methods for
// each of the objects stored in the fitnessTrackers array?
public String toString() {
// TODO Implement
String s = "";
for (FitnessTracker ft : fitnessTrackers) {
s = s + ft.toString() + "\n";
}
return s;
}
// Method displays in console the details of this experiment which include:
// - Summary of the measurements of each individual fitness tracker
// (indicating if they are steps, distance or heart rate measurements)
// - The total number of fitness trackers that participated in this experiment
// - A summary of total number of steps and total distance walked in this experiment
// Implementation hint: Should it use the toString() method right above this method?
public void printExperimentDetails() {
// TODO Implement
System.out.print(this.toString());
System.out.println(fitnessTrackers.length + " number of fitness trackers are participated in this experiment");
System.out.println("Total number of steps : " + getTotalSteps());
System.out.println("Total number of steps : " + getTotalDistance());
}
// Method should iterate through all fitness tracker step measurements and returns a double
// with the total step count (see Task 2)
// Implementation hint: The instanceof operator and casting may become handy here
public int getTotalSteps() {
// TODO Implement
int totalSteps = 0;
for (FitnessTracker ft : fitnessTrackers) {
if (ft instanceof StepsFitnessTracker)
totalSteps = totalSteps + ((StepsFitnessTracker) ft).getTotalSteps().getValue();
}
return totalSteps;
}
// Method should iterate through all fitness tracker distance measurements and returns a double with the total distance
// Implementation hint: The instanceof operator and casting may become handy here
public double getTotalDistance() { double totalDistance=0;
for(FitnessTracker ft :fitnessTrackers){ if(ft instanceof
DistanceFitnessTracker) totalDistance = totalDistance +
((DistanceFitnessTracker)ft).getDistance(); } return totalDistance;
}
public FitnessTracker[] getTrackersEqualTo(FitnessTracker tracker) {
int count = 0;
for(FitnessTracker fitnessTracker:fitnessTrackers){
if(fitnessTracker.equals(tracker))
count++;
}
FitnessTracker[] resultfitnessTrackers = new FitnessTracker[count];
int index = 0;
for(FitnessTracker fitnessTracker:fitnessTrackers){
if(fitnessTracker.equals(tracker))
resultfitnessTrackers[index++] = fitnessTracker;
}
return resultfitnessTrackers;
}
}
Distancefitnesstrakcer.java
public class DistanceFitnessTracker extends FitnessTracker {
Distance distance;
public DistanceFitnessTracker(String modelName, Distance distance) {
super(modelName);
this.distance = distance;
};
}
I keep getting this error what to do?
Steps Tracker steps; Total Steps: 230
Steps Tracker steps2; Total Steps: 150
Steps Tracker steps2; Total Steps: 150
Heart Rate Tracker hr; Average Heart Rate: 80.0, for 1 measurements
Heart Rate Tracker hr; Average Heart Rate: 80.0, for 1 measurements
uk.ac.sheffield.com1003.problemsheet2.DistanceFitnessTracker@470e2030
uk.ac.sheffield.com1003.problemsheet2.DistanceFitnessTracker@3fb4f649
uk.ac.sheffield.com1003.problemsheet2.DistanceFitnessTracker@33833882
Exception in thread "main" 8 number of fitness trackers are participated in this experiment
Total number of steps : 530
java.lang.Error: Unresolved compilation problem:
The method getDistance() is undefined for the type DistanceFitnessTracker
at uk.ac.sheffield.com1003.problemsheet2.FitnessExperiment.getTotalDistance(FitnessExperiment.java:91)
at uk.ac.sheffield.com1003.problemsheet2.FitnessExperiment.printExperimentDetails(FitnessExperiment.java:69)
at uk.ac.sheffield.com1003.problemsheet2.FitnessExperiment.main(FitnessExperiment.java:34)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- An incomplete program named w_pl java, which must include three methods, is provided. You must implement two methods within the program as described below. The third method, which is a main method, is already written in the program and you can use it to test your program. The two methods you mast inplement are: • find method • Signature: public statie vold find(int[] a, int x) • Behavior: • Reccives an array of integers a and an integer x • Performs a linear search on a for x. • If x is in a, print the indexes of the aray slots where x is stored (there may be multiple x's in a) on the screen. • Ifx is not in a, print the message "x does not exist" on the screen. Note that you should not use Java's built-in method. Your program must scan and search the array. • isPrifie method • Signature: public statie boolean isPrefix(String s1, String s2) • Behavior: • Receives two strings sl and s2. Assume that length of sl length of s2. Returns true if sl is a prefix of s2 and returns false…arrow_forwardBased on the information in the screenshots, please answer the following question below. The language used is Java and please provide the code for it with the explanation. Create a testing class named “PlanTest”. Under this class, there needs to be another static method besides the main method: You will need to create a static method named “prerequisiteGenerator” under “PlanTest”, which return a random 2D array containing prerequisite pairs (e.g., {{1, 3}, {2, 3}, {4, 2}}). The value range of a random integer is [0, 10] (inclusive). This method will accept an integer parameter, which specifies the length of the returned array: int[][] pre = prerequisiteGenerator(3); // possibly {{1, 3}, {2, 3}, {4, 2}} Under the main method, you will need to use prerequisiteGenerator to generate random prerequisite pair lists, and perform tests on plan method at least three examples by printing proper messages. Given 4 courses and prerequisites as [[1,0], [2,0], [3,1]] It is possible to take all…arrow_forwardFirst, implement a python class called Student. This class should store 2 variables, name and grade. Later, implement a class called Classroom. The constructor of this class should input the list of students in this classrom. This class should also contain 3 methods, add_student, remove_student and calculate_stats. As the names suggest, add_student method should input a Student object and add that to its' already existing student list, remove_student must input a full name(string) and delete the student with the provided name(you can assume no 2 students have the same full name). Finally, calculate_stats method must calculate and print the following statistics of the grades: 1. The name of the student with highest grade and the grade itself. 2. The name of the student with lowest grade and the grade itself. 3. The average gradearrow_forward
- A class Date has methods intgetMonth() and intgetDay(). Write a method public boolean has3OnSameDay(Date[] birthdays) that returns true if at least three birthdays in the array fall on the same date. Your method should work in O(n) time, where n = birthdays.length.arrow_forwardIn Java.arrow_forwardPlease complete the task by yourself only in JAVA with explanation. Don't copy. Thank you. Using quicksort to sort an array of car objects by various criteria. Define a class Car as follows: class Car { public String make; public String model; public int mpg; // Miles per gallon } b) Implement a comparator called CompareCarsByDescendingMPG that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByDescendingMPG should return a value that will cause quicksort to sort an array of cars in descending order (from largest to smallest) by mpg.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
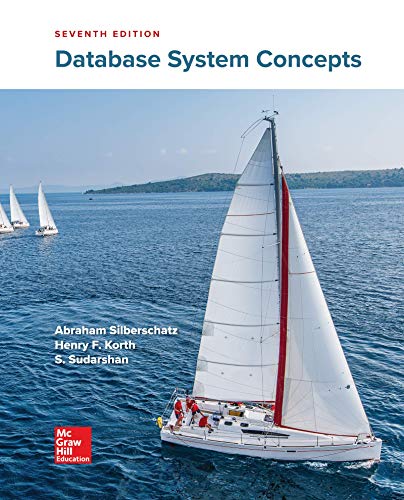
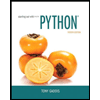
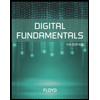
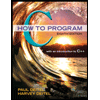
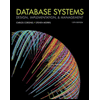
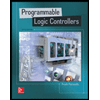