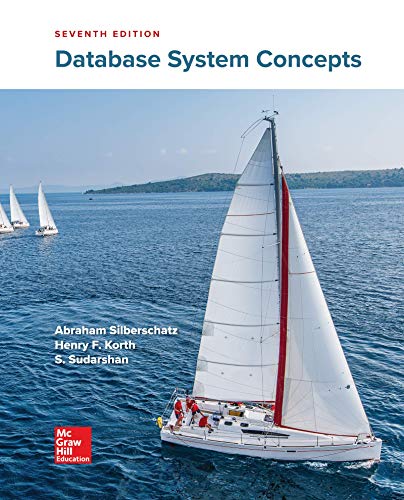
Implement the quadratic_formula() function. The function takes 3 arguments, a, b, and c, and computes the two results of the quadratic formula:
The quadratic_formula() function returns the tuple (x1, x2). Ex: When a = 1, b = -5, and c = 6, quadratic_formula() returns (3, 2).
Code provided in main.py reads a single input line containing values for a, b, and c, separated by spaces. Each input is converted to a float and passed to the quadratic_formula() function.
Ex: If the input is:
2 -3 -77
the output is:
Solutions to 2x^2 + -3x + -77 = 0 x1 = 7 x2 = -5.50
code:
# TODO: Import math module
def quadratic_formula(a, b, c):
# TODO: Compute the quadratic formula results in variables x1 and x2
return (x1, x2)
def print_number(number, prefix_str):
if float(int(number)) == number:
print("{}{:.0f}".format(prefix_str, number))
else:
print("{}{:.2f}".format(prefix_str, number))
if __name__ == "__main__":
input_line = input()
split_line = input_line.split(" ")
a = float(split_line[0])
b = float(split_line[1])
c = float(split_line[2])
solution = quadratic_formula(a, b, c)
print("Solutions to {:.0f}x^2 + {:.0f}x + {:.0f} = 0".format(a, b, c))
print_number(solution[0], "x1 = ")
print_number(solution[1], "x2 = ")

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- int p =5 , q =6; void foo ( int b , int c ) { b = 2 * c ; p = p + c ; c = 1 + p ; q = q * 2; print ( b + c ); } main () { foo (p , q ); print p , q ; } Explain and print the output of the above code when the parameters to the foo function are passed by value. Explain and print the output of the above code when the parameters to the foo function are passed by reference. Explain and print the output of the above code when the parameters to the foo function are passed by value result. Explain and print the output of the above code when the parameters to the foo function are passed by name.arrow_forwardWrite a fraction calculator program. Your program should check for the division by 0, have and use the following functions: abs - returns the absolute value of a given integer. min - returns the smallest of two positive integers. gcd - returns the greatest common divisor of two positive integers. reduce - reduces a given fraction. flip - reduces a given fraction and flips the sign if the denominator is negative. the program should run repeatedly until the user wants to quit.arrow_forwardpython codearrow_forward
- Function scale multiples its first argument (a real number) by 10 raised to the power indicated by its second argument (an integer). For example, the function call scale (2.5, 2) double scale (double x, int n) double scale_factor; scale factor - pow (10, n); return x*scale_factor; Returns the value 250.0 (2.5 x 102). The function call scale (2.5, -2) Return the value 0.025 (2.5 x 10²) Write only the main function, create and initialize a double array and then call the function scale for each elements of the array. At the end, the contents of the array must consist of scaled values. Create and use a separate integer array as the powers of each corresponding value. Eg. If double array has the following contents: 1.0 2.0 3.0 4.0 and the Integer array of powers have the following: 4 3. 2 -2, After function calls, the new contents of the double array must be updated as in seen in the following: 1.0x104 2.0x10³ 3.0x10² 4.0x10²arrow_forwardJava, I am not displaying my results correct. It should add up the digits in the string. input string |result for the sumIt Recursion functions and a findMax function that finds the largest number in a string "1d2d3d" | 6 total "55" |10 total "xx" | 0 total "12x8" |12 Max number "012x88" |88 Max Number "012x88ttttt9xe33ppp100" |100 Max Number public class Finder { //Write two recursive functions, both of which will parse any length string that consists of digits and numbers. Both functions //should be…arrow_forwardFinish the function below that takes in the length of the two parallel sides of a trapezoid a and b, which are separated by a distance h, and returns its area. In [ ]: defarea_of_trapezoid(a,b,h):# YOUR CODE HEREraiseNotImplementedError() In [ ]: """Check that area_of_triangle returns the correct output for several inputs""" assert abs(area_of_trapezoid(3,3, 5) - 15) <=.1 assert abs(area_of_trapezoid(3,4, 5) - 17.5)arrow_forward
- Implement the following function: Code should be written in python.arrow_forwardin the C++ version please suppose to have a score corresponding with probabilities at the end and do not use the count[] function. Please explain the detail when coding. DO NOT USE ARRAY. The game of Pig The game of Pig is a dice game with simple rules: Two players race to reach 100 points. Each turn, a player repeatedly rolls a die until either a 1 ("pig") is rolled or the player holds and scores the sum of the rolls (i.e. the turn total). At any time during a player's turn, the player is faced with two decisions: roll - if the player rolls 1: the player scores nothing and it becomes the opponents turn. 2 - 6: the number is added to the player's turn total and the player's turn continues. hold - The turn total is added to the player's score and it becomes the opponent's turn. This game is a game of probability. Players can use their knowledge of probabilities to make an educated game decision. Assignment specifications Hold-at-20 means that the player will choose to roll…arrow_forwardComplete the rotate_text() function that takes 2 parameters, a string data and an integer n. If n is positive, then the function will shift all the characters in data forward by n positions, with characters at the end of the string being moved to the start of the string. If n is 0 then the text remains the same. For example: rotate_text('abcde', rotate_text('abcde', rotate_text('abcde', 1) would return the string 'eabcd' 3) would return the string 'cdeab' 5) would return the string 'abcde' rotate_text('abcde', 6) would return the string 'eabcd' ... and so on. If n is negative, then the function will shift the characters in data backward by n positions, with characters at the start of the string being moved to the end of the string. For example: rotate text('abcde', -1) would return the string 'bcdea'arrow_forward
- def square(x): return x * X def halve(x): return x // 2 def twice(f,x): """Apply f to the result of applying f to x >>> twice (square,3) 81 >>> twice (square, 4) 256 >>> twice (halve, 32) 8 >>> twice (halve, 80) 20 *** YOUR CODE HERE ***"arrow_forwardWrite a program that will:• Select a random number between 1 and 100.• Test if the number is prime• Call a function named isPrime(). Pass the number to be tested. isPrime() should test whetherthe number has any factors other than 1 and itself. isPrime() returns either True or False.• isPrime() should use a function isDivisible(x,y) to test if y divides evenly into x. That is,isDivisible() will identify whether y is a factor of x.• Print the resultarrow_forwardWrite a recursive function that takes as a parameter a nonnegative integer and generates the following pattern of stars. If the nonnegative integer is 4, the pattern generated is as follows: **** *** ** * * ** *** **** Also, write a program that prompts the user to enter the number of lines in the pattern and uses the recursive function to generate the pattern. For example, specifying 4 as the number of lines generates the preceding pattern.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
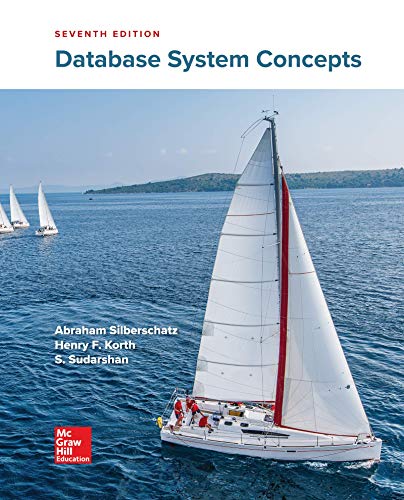
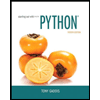
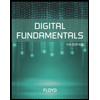
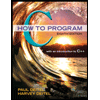
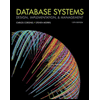
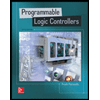