Implement a program that prompts the user to enter two positive numbers as formatted below Enter num1: Enter num2: Input validation MUST be completed input must be all digits input must be a positive number, Integer Overflow for the individual inputs and the sum of the inputs is now VALID and needs to be handled as described below with the ADDITION ALGORITHM. If NOT valid (non-digits or negative) then re-prompt as formatted below INVALID RE-Enter num 3 incorrect inputs in a row and stop program. This is the 3 strikes and you're out rule. PROGRAM ABORT ADDITION ALGORITH The input CANNOT be converted to a true int, unsigned int, long, unsigned long or long long data type The solution is to use an array to store each individual digit of the number For example, the inputted number: "1234" could be stored in the array nums backwards, so the ones position is at index 0 of the array. 4 = 10^0 (ones position) so that nums[0] = 4 3 = 10^1 (tens position) so that nums[1] = 3 2 = 10^2 (hundreds position) so that nums[2] = 2 1 = 10^3 (thousands position) so that nums[3] = 1 The program will perform the addition operation by implementing the usual paper-and-pencil addition algorithm (adding the ones digits and if greater than 10, then carry the 1 to the tens position, etc) Add starting with ones digits and carry the 1 if 10 or over. For the below example the following steps are completed sum[0] = 5+6+0(no carry over) =11 store the 1 and carry the 1 to the next index. sum[1] = 4+7+1(carry over)=12 store the 2 and carry the 1 to the next index. sum[2] = 3+8+1(carry over) = 12 store the 2 and carry the 1 to the next index. sum[3] = 2+9+1(carry over) = 12 store the 2 and carry the 1 to the next index. sum[4] = 1+0+1(carry over) = 2 store the 2 Reverse the sum array to get the answer. The ones place at index 0, should be written as the right most value. Answer: 22221 Outputs the sum of the two positive integers when input is valid as formatted below in the example test runs. I'm not getting the results needed for test #3-6 Test Run #3 Enter num1: -378 INVALID RE-Enter num1: abc INVALID RE-Enter num1: 18446744073709551616 Enter num2: 16abc INVALID RE-Enter num2: -16 INVALID RE-Enter num2: 18446744073709551616 18446744073709551616 + 18446744073709551616 ------------------------------------ 36893488147419103232 Test Run #4 Enter num1: -378 INVALID RE-Enter num1: abc INVALID RE-Enter num1: 18446744073709551614 Enter num2: 16abc INVALID RE-Enter num2: -16 INVALID RE-Enter num2: -2 PROGRAM ABORT Enter num1: -378 INVALID RE-Enter num1: abc INVALID RE-Enter num1: 18446744073709551619 Enter num2: 16abc INVALID RE-Enter num2: -16 INVALID RE-Enter num2: 1 18446744073709551619 + 1 ------------------------------------- 18446744073709551620 Test Run #6 Enter num1: 111213141516171819 Enter num2: 2132435465768798 111213141516171819 + 2132435465768798 -------------------------------- 113345576981940617
Implement a
Enter num1: Enter num2: Input validation MUST be completed input must be all digits input must be a positive number, Integer Overflow for the individual inputs and the sum of the inputs is now VALID and needs to be handled as described below with the ADDITION
The input CANNOT be converted to a true int, unsigned int, long, unsigned long or long long data type
The solution is to use an array to store each individual digit of the number
For example, the inputted number: "1234" could be stored in the array nums backwards, so the ones position is at index 0 of the array.
4 = 10^0 (ones position) so that nums[0] = 4 3 = 10^1 (tens position) so that nums[1] = 3 2 = 10^2 (hundreds position) so that nums[2] = 2 1 = 10^3 (thousands position) so that nums[3] = 1 The program will perform the addition operation by implementing the usual paper-and-pencil addition algorithm (adding the ones digits and if greater than 10, then carry the 1 to the tens position, etc)
Add starting with ones digits and carry the 1 if 10 or over. For the below example the following steps are completed sum[0] = 5+6+0(no carry over) =11 store the 1 and carry the 1 to the next index. sum[1] = 4+7+1(carry over)=12 store the 2 and carry the 1 to the next index. sum[2] = 3+8+1(carry over) = 12 store the 2 and carry the 1 to the next index. sum[3] = 2+9+1(carry over) = 12 store the 2 and carry the 1 to the next index. sum[4] = 1+0+1(carry over) = 2 store the 2 Reverse the sum array to get the answer. The ones place at index 0, should be written as the right most value. Answer: 22221 Outputs the sum of the two positive integers when input is valid as formatted below in the example test runs.
I'm not getting the results needed for test #3-6
Test Run #3
Test Run #4
Enter num1: -378
INVALID RE-Enter num1: abc
INVALID RE-Enter num1: 18446744073709551619
Enter num2: 16abc
INVALID RE-Enter num2: -16
INVALID RE-Enter num2: 1
18446744073709551619
+ 1
-------------------------------------
18446744073709551620
Test Run #6
Below is my C++ code already started
#include <iostream>
#include <bits/stdc++.h>
bool isNumber(std::string s) {
for (int i = 0; i < s.length(); i++)
if(isdigit(s[i]) == false)
return false;
return true;
}
std::string findSum(std::string str1, std::string str2) {
if(str1.length() > str2.length())
swap(str1, str2);
std::string str = "";
int n1 = str1.length(), n2 = str2.length();
reverse(str1.begin(), str1.end());
reverse(str2.begin(), str2.end());
int carry = 0;
for (int i = 0; i < n1; i++) {
int sum = ((str1[i] - '0') + (str2[i] - '0') + carry);
str.push_back(sum % 10 + '0');
carry = sum / 10;
}
for (int i = n1; i < n2; i++) {
int sum = ((str2[i] - '0') + carry);
str.push_back(sum % 10 + '0');
carry = sum / 10;
}
if (carry)
str.push_back(carry + '0');
reverse(str.begin(), str.end());
return str;
}

Step by step
Solved in 4 steps with 2 images

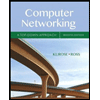
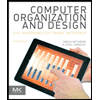
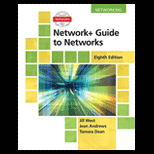
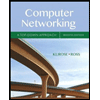
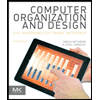
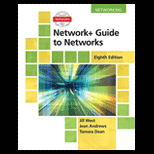
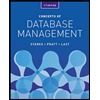
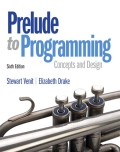
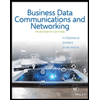