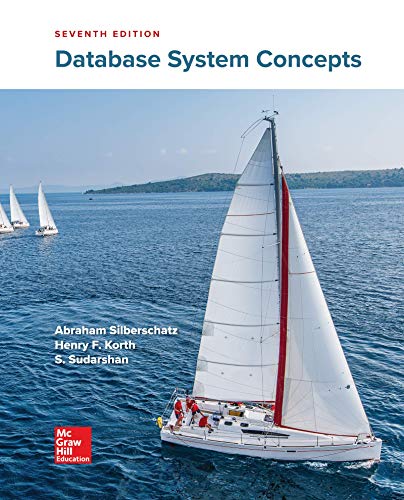
Concept explainers
//client.c
#include "csapp.h"
int main(int argc, char **argv)
{
int connfd;
rio_t rio;
connfd = Open_clientfd(argv[1], argv[2]);
Rio_readinitb(&rio, connfd);
char buffer[MAXLINE];
printf("Your balance is %s\n", buffer);
Close(connfd);
exit(0);
int choice;
float amount;
int acc2;
char buffer[MAXLINE];
rio_t rio;
Rio_readinitb(&rio, connfd);
while (1)
{
printf("\nHello welcome to the Bank Management System\n");
printf("----------------------\n");
printf("1. Check Balance\n");
printf("2. Deposit\n");
printf("3. Withdraw\n");
printf("4. Transfer\n");
printf("5. Quit\n");
printf("Enter your choice: ");
Rio_writen(connfd, &choice, sizeof(int));
if (choice == 1)
{
printf("Your balance is %s\n", buffer);
}
else if (choice == 2)
{
printf("Enter amount to deposit: ");
Rio_writen(connfd, &amount, sizeof(float));
printf("%s\n", buffer);
}
else if (choice == 3)
{
printf("Enter amount to withdraw: ");
Rio_writen(connfd, &amount, sizeof(float));
printf("%s\n", buffer);
}
else if (choice == 4)
{
printf("Enter amount to transfer: ");
printf("Enter recipient account number: ");
Rio_writen(connfd, &amount, sizeof(float));
Rio_writen(connfd, &acc2, sizeof(int));
printf("%s\n", buffer);
printf("Recipient's new balance is %s\n", buffer);
}
else if (choice == 5)
{
Close(connfd);
printf("Connection Closed!!\n");
break;
}
}
exit(0);
}
//server.c
#include "csapp.h"
void *thread(void *vargp);
int main(int argc, char **argv)
{
int listenfd, *connfdp;
socklen_t clientlen;
struct sockaddr_storage clientaddr;
pthread_t tid;
listenfd = Open_listenfd(argv[1]);
while (1)
{
clientlen = sizeof(struct sockaddr_storage);
connfdp = Malloc(sizeof(int));
*connfdp = Accept(listenfd, (SA *)&clientaddr, &clientlen);
Pthread_create(&tid, NULL, thread, connfdp);
}
}
void *thread(void *vargp)
{
int connfd = *((int *)vargp);
Pthread_detach(pthread_self());
Free(vargp);
FILE *fp = fopen("bank.txt", "r");
char buf[MAXLINE];
int account_number, balance;
char name[MAXLINE];
rio_t rio;
while (fgets(buf, MAXLINE, fp) != NULL)
{
sscanf(buf, "%d %s %d", &account_number, name, &balance);
}
fclose(fp);
sprintf(buf, "%d", balance);
Rio_writen(connfd, buf, strlen(buf));
while (1)
{
if (Rio_readlineb(&rio, buf, MAXLINE) <= 0)
{
break;
}
int choice = buf[0] - '0';
if (choice == 1)
{
sprintf(buf, "%d\n", balance);
Rio_writen(connfd, buf, strlen(buf));
}
else if (choice == 2)
{
if (Rio_readlineb(&rio, buf, MAXLINE) <= 0)
{
break;
}
int deposit_amount = atoi(buf);
balance += deposit_amount;
sprintf(buf, "Deposit successful. New balance: %d\n", balance);
Rio_writen(connfd, buf, strlen(buf));
}
else if (choice == 3)
{
if (Rio_readlineb(&rio, buf, MAXLINE) <= 0)
{
break;
}
int withdrawal_amount = atoi(buf);
if (balance >= withdrawal_amount)
{
balance -= withdrawal_amount;
sprintf(buf, "Withdrawal successful. New balance: %d\n", balance);
Rio_writen(connfd, buf, strlen(buf));
}
}
else if (choice == 4)
{
if (Rio_readlineb(&rio, buf, MAXLINE) <= 0)
{
break;
}
int transfer_amount = atoi(buf);
if (Rio_readlineb(&rio, buf, MAXLINE) <= 0)
{
break;
}
int destination_account = atoi(buf);
if (balance >= transfer_amount)
{
balance -= transfer_amount;
sprintf(buf, "Transfer successful. New balance: %d\n", balance);
Rio_writen(connfd, buf, strlen(buf));
}
}
else if (choice == 5)
{
Close(connfd);
break;
}
}
return NULL;
}
//bank.txt
100 John Smith 500.00
200 Jane Doe 1000.00
300 Luke Skylar 1500.00
Can you help me make a connection with the client and server and be able to read the text file?

Step by stepSolved in 5 steps

- #ifndef LLCP_INT_H#define LLCP_INT_H #include <iostream> struct Node{ int data; Node *link;};void DelOddCopEven(Node*& headPtr);int FindListLength(Node* headPtr);bool IsSortedUp(Node* headPtr);void InsertAsHead(Node*& headPtr, int value);void InsertAsTail(Node*& headPtr, int value);void InsertSortedUp(Node*& headPtr, int value);bool DelFirstTargetNode(Node*& headPtr, int target);bool DelNodeBefore1stMatch(Node*& headPtr, int target);void ShowAll(std::ostream& outs, Node* headPtr);void FindMinMax(Node* headPtr, int& minValue, int& maxValue);double FindAverage(Node* headPtr);void ListClear(Node*& headPtr, int noMsg = 0); // prototype of DelOddCopEven of Assignment 5 Part 1 #endifarrow_forwardWhat are the values of the variable number and the vector review after the following code segment is executed? vector<int> review(10,-1); int number; number = review[0]; review[1] = 150; review[2] = 350; review[3] = 450; review.push_back(550); review.push_back(650); number += review[review.size()-1]; review.pop_back();arrow_forwardWhat are the pid values?arrow_forward
- #ifndef INT_SET_H#define INT_SET_H #include <iostream> class IntSet{public: static const int DEFAULT_CAPACITY = 1; IntSet(int initial_capacity = DEFAULT_CAPACITY); IntSet(const IntSet& src); ~IntSet(); IntSet& operator=(const IntSet& rhs); int size() const; bool isEmpty() const; bool contains(int anInt) const; bool isSubsetOf(const IntSet& otherIntSet) const; void DumpData(std::ostream& out) const; IntSet unionWith(const IntSet& otherIntSet) const; IntSet intersect(const IntSet& otherIntSet) const; IntSet subtract(const IntSet& otherIntSet) const; void reset(); bool add(int anInt); bool remove(int anInt); private: int* data; int capacity; int used; void resize(int new_capacity);}; bool operator==(const IntSet& is1, const IntSet& is2); #endifarrow_forwardProgramarrow_forwardGiventhe following C program that contains struct student, that has name and age, and a pointer to struct student *ptr, but does not assign a value to it: 1. What gdb commands would you use to find the error it contains? #include <stdio.h> /*write program Then, print ptr->age*/ //make struct student struct student { charname[25]; int age; }; int main() { //declare pointer to struct student struct student *ptr; //print ptr -> age printf("%d", ptr->age); return0; }arrow_forward
- C++arrow_forwardstruct Info { int id; float cost; }; Given the struct above, the following code segment will correctly declare and initialize an instance of that struct. Info myInfo = {10,4.99}; Group of answer choices True False ============== Consider the following code fragment. int a[] = {3, -5, 7, 12, 9}; int *ptr = a; *ptr = (*ptr) + 1; cout << *ptr; Group of answer choices 4 -5 3 Address of -5 =============== What will happen if I use new without delete? Group of answer choices The program will never compile The program will never run The program will run fine without memory leak None of thesearrow_forwardGiven the following declaration : char msg[100] = "Department of Computer Science"; What is printed by: strcpy_s(msg, "University"); int len = strlen(msg); for (int i = len-3; i >0; i--) { msg[i] = 'x'; } cout << msg;arrow_forward
- Game of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forwardC++ Programming Requirments: Please submit just one file for the classes and main to test the classes. Just create the classes before main and submit only one cpp file. Do not create separate header files. Please note: the deleteNode needs to initialize the *nodePtr and the *previousNode. The code to do this can be copied from here:ListNode *nodePtr, *previousNode = nullptr; Part 1: Your own Linked ListDesign your own linked list class to hold a series of integers. The class should have member functions for appending, inserting, and deleting nodes. Don't forget to add a destructor that destroys the list. Demonstrate the class with a driver program.Part 2: List PrintModify the linked list class you created in part 1 to add a print member function. The function should display all the values in the linked list. Test the class by starting with an empty list, adding some elements, and then printing the resulting list out.arrow_forwardvoid getVectorSize(int& size); void readData(vector<Highscore>& scores); void sortData(vector<Highscore>& scores); vector<Highscore>::iterator findLocationOfLargest( const vector<Highscore>::iterator startingLocation, const vector<Highscore>::iterator endingLocation); void displayData(const vector<Highscore>& scores); The size parameter from the given code won't be needed now, since a vector knows its own size. Notice that the findLocationOfLargest() function does not need the vector itself as a parameter, since you can access the vector using the provided iterator parameters. The name field in the struct must still be a c-string The focus of this assignment is to use iterators. You must use iterators wherever possible to access the vector. As a result, you must not use square brackets, the push_back() function, the at() function, etc. Also, the word "index" shouldn't appear in your code anywhere. You won't get full credit if…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
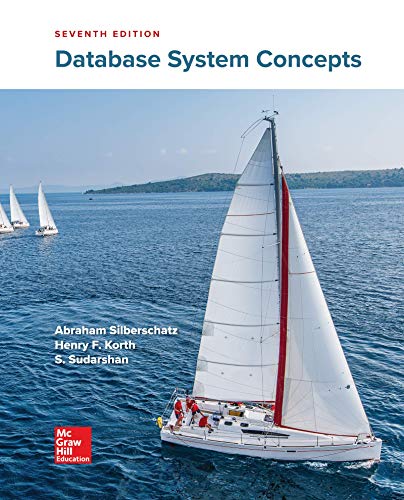
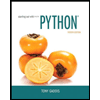
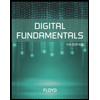
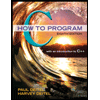
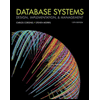
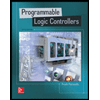