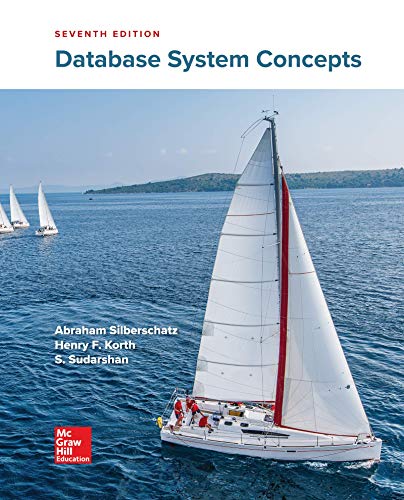
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
- Idiot’s Delight is a fairly simple game of solitaire, yet it is difficult to win. The goal is to draw all of the cards from the deck, and end up with no cards left in your hand. You will run through the deck of cards one time. Start by dealing 4 cards to your hand. You will always look at the last 4 cards in your hand. If the ranks of the “outer” pair (1st and 4th) are the same, discard all four cards. Otherwise, if the suits of the “inner” pair (2nd and 3rd) are the same, discard those 2 cards only. If you have less than 4 cards, draw enough to have 4 cards in your hand. If the deck is empty, the game is over. Your score will be the number of cards that remain in your hand. Like in golf, the lower the score the better.
- Create a new Python module in a file named “idiots_delight.py”. Add a function called deal_hand that creates a standard deck of cards, deals out a single hand of 4 cards and returns both the hand and the deck. Remember that for the last assignment, you created several functions to emulate cards. To help with testing, don’t shuffle the deck at this time. Deal out 4 cards.
- Add a function called discard that declares parameters for a hand and the number of cards to discard. If the number is not either 4 or 2, or there are less than 4 cards in the hand, simply return the hand. If the number is 4, discard the last 4 cards from the hand, otherwise if the number is 2, discard the 2 inner cards (the 2 before the last card). Return the hand.
- We’ll build up the function in this module that plays the main game. Add a function called play_round that declares parameters for a deck and a hand of cards (lists of card tuples). Start by ensuring that you have enough cards to play a round with. If you have less than 4 cards, draw from the deck until you have a hand of 4 cards. Print out the hand. At the end of the function, if there are still cards in the deck, draw one more card and print the hand. Return the deck and the hand. Hint: testing at this point should avoid either of the discard scenarios described in step 1 to ensure adding code for those scenarios later doesn’t impact your testing.
- The next step in the play_round function is immediately after ensuring that you have at least 4 cards. Loop until you have less than 4 cards, or you did not discard. Check if the 1st and 4th of the last four cards are the same rank. If they are, remove all 4 of those cards from the hand by calling your discard function.
- The next step in the play_round function is only done when the previous check of the 1st and 4th of the last 4 cards are not the same rank. In this case, check if the 2nd and 3rd cards of the last four are the same suit. If they are, remove only the 2nd and 3rd cards from the hand.
- Add a main function to your “idiots_delight.py” module. You need to deal a hand, and call play_round module until there are no cards left in the deck. When the game has ended, print a message telling them the game has ended as well as how many cards are left in their hand. If there are 0 cards left, also let them know that they are a winner, otherwise, give them encouragement to try again. Manually run the game twice. You should get the same score both times since we didn’t shuffle the deck. See the example snippets from two games below:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 6 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- This does not appear to be the answer to this question; Cheese's position is randomly generated in a 5x5 grid. The initial positions are x,y. The position of Cheese is not specified as (3, 4)arrow_forwardKnight's Tour: The Knight's Tour is a mathematical problem involving a knight on a chessboard. The knight is placed on the empty board and, moving according to the rules of chess, must visit each square exactly once. There are several billion solutions to the problem, of which about 122,000,000 have the knight finishing on the same square on which it begins. When this occurs the tour is said to be closed. Your assignment is to write a program that gives a solution to the Knight's Tour problem recursively. You must hand in a solution in C++ AND Java. The name of the C++ file should be "main.cc" and the name of the Java file should be "Main.java". Write C++ only with a file name of main.cc Please run in IDE and check to ensure that there are no errors occuring Output should look similar to: 1 34 3 18 49 32 13 16 4 19 56 33 14 17 50 31 57 2 35 48 55 52 15 12 20 5 60 53 36 47 30 51 41 58 37 46 61 54 11 26 6 21 42 59 38 27 64 29 43 40 23 8 45 62 25 10 22 7 44 39 24 9 28 63arrow_forwardJava Programming: Write a program that reads five integer values from the user, then analyzes them as if they were a hand of cards. When your program runs it might look like this (user input is in orange for clarity): Enter five numeric cards, no face cards. Use 2 - 9. Card 1: 8 Card 2: 7 Card 3: 8 Card 4: 2 Card 5: 9 Pair! (This is a pair, since there are two eights). You are only required to find the hands Pair and Straight.arrow_forward
- You have a pack of 5 randomly numbered cards, which can range from 0-9. You can win if you can produce a higher two-digit number from your cards than your opponent. Return true if your cards win that round. Examples winRound ([2, 5, 2, 6, 9], [3, 7, 3, 1, 2]) → true // Your cards can make the number 96 // Your opponent can make the number 73 // You win the round since 96 > 73 winRound ([2, 5, 2, 6, 9], [3, 7, 3, 1, 2]) → true winRound ([1, 2, 3, 4, 5], [9, 8, 7, 6, 5]) → false winRound ([4, 3, 4, 4, 5], [3, 2, 5, 4, 1]) → falsearrow_forwardFaceUp card game In this assignment we will implement a made-up card game we'll call FaceUp. When the game starts, you deal five cards face down. Your goal is to achieve as high a score as possible. Your score only includes cards that are face up. Red cards (hearts and diamonds) award positive points, while black cards (clubs and spades) award negative points. Cards 2-10 have points worth their face value. Cards Jack, Queen, and King have value 10, and Ace is 11. The game is played by flipping over cards, either from face-down to face-up or from face-up to face-down. As you play, you are told your total score (ie, total of the face-up cards) and the total score of the cards that are face down. The challenge is that you only get up to a fixed number of flips and then the game is over. Here is an example of the output from playing the game: FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 0 Face down total: -5 Number of flips left: 5 Pick a card to flip between 1…arrow_forwardYou will build a simplified, one-player version of the classic board game Battleship! In this version of the game, there will be a single ship hidden in a random location on a 5 × 5 grid. The player will have 4 guesses at most to try to sink the ship. At each guess, the player names an attacking coordinate, that is (“guess row”, “guess col”). The game ends in two conditions: (1) the player is out of guesses; (2) the player hits the ship. Examples are given in Figure 1. The 5 × 5 board is shown every time the player inputs a guess entry. The ship takes only one entry of the board and it is randomly given before the player’s guesses. The player inputs guessing entries in the Python console. Entries missed the ship are replaced by “X” in the board. You must use loop in your code. Please submit your code and console screenshots to bartleby. Code contain- ing syntax error will be graded zero. Hints: 1 Create a variable board and set it equal to an empty list…arrow_forward
- : You will build a simplified, one-player version of the classic board game Battleship! In this version of the game, there will be a single ship hidden in a random location on a 5 °ø 5 grid. The player will have 4 guesses at most to try to sink the ship. At each guess, the player names an attacking coordinate, that is (“guessrow”, “guess col”). The game ends in two conditions: (1) the player is out of guesses; (2) the player hits the ship. Examples are given in Figure 1. § The 5 X 5 board is shown every time the player inputs a guess entry. § The ship takes only one entry of the board, and it is randomly given before the player’s guesses. § The player inputs guessing entries in the Python console. § Entries that missed the ship are replaced by “X” on the board. § You must use a loop in your code. § Please submit your code and console screenshots to Blackboard. Code containing syntax error will be graded zero. Hints: 1. Create a variable board and set it equal to an empty list and…arrow_forwardcanMove(int x, int y, int destX, int destY, Side s): This method returns true if the player of color s can move the piece at coordinates (x,y) can move to coordinates (destX, destY) on the board in its current state. This means that here you do need to consider this piece’s interaction with other pieces on the board. Conditions for this method to return false are given in the code. public boolean canMove(int x, int y, int destX, int destY, Side s){ /* TODO write a method that checks if a piece at coordinates x,y can move to coordinates destX,destY Conditions for false: - Origin or destination coordinates are outside the board - Piece at origin is null - If source and destination coordinates are the same - Piece at origin is not of the same side as s - You can check this using piece.getSide() - Piece cannot move to the destination by piece movement rules - You should check this using Piece.canMove(destX,…arrow_forwardImplement the "Add player" menu option. Prompt the user for a new player's jersey number and rating. Append the values to the two vectors. Ex: Enter a new player's jersey number: 49 Enter the player's rating: 8arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
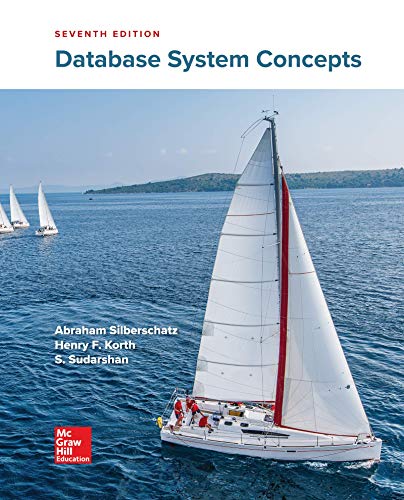
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
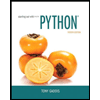
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
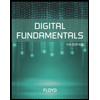
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
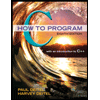
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
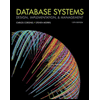
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
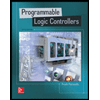
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education