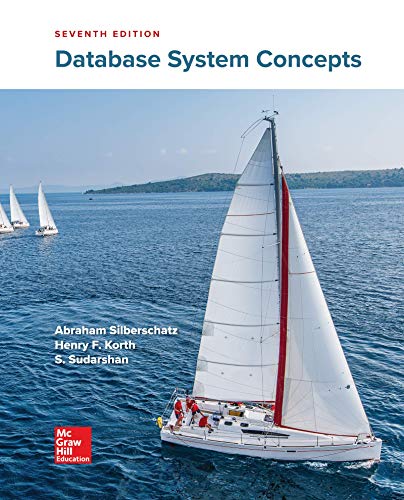
I would like to just plot the data, that is the result from the mean (see code in bold).
import numpy as np
import matplotlib.pyplot as plt
import glob
from astropy.io import fits
from astropy.wcs import WCS
%matplotlib inline
%matplotlib widget
fig, ax = plt.subplots(figsize = (10,10)) # figsize changes the size of the plot)
data_dir = glob.glob('/Users/xxxxxxxxx/Desktop/harpn_sun_release_package_ccf_2018/2018-01-18/*.fits')
lams = []
fluxs = []
for file_path in data_dir:
hdul = fits.open(file_path)
data = hdul[1].data
h1 = hdul[1].header
flux = data[1]
w = WCS(h1, naxis=1, relax=False, fix=False)
lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0]
lams.append(lam)
fluxs.append(flux)
ax.plot(lam,flux)
mean_flux = np.mean(fluxs, axis = 0)
ax.plot(lams[0], mean_flux, color = 'k', linewidth = 2)
max_rv = lams[0][np.argmin(mean_flux)]
ax.vlines(max_rv, np.min(fluxs), np.max(fluxs), color = 'r', linestyle='--')
ax.set_xlabel('RV [km/s]')
ax.set_ylabel('Normalized CCF')
plt.title('CCF')
plt.show()
print(mean_flux)
![40000
30000
0
10
20
RV [km/s]
30
40
93492.52
[104930.09 103464.03 101587.89 99710.74 98333.64
97645.76 98206.375 99064.03 100027.09 100870.03
101821.414 101427.24 100071.93 97552.41
79864.69 70415.9 60115.39 50119.555
34768.734 37060.95 43239.555 52353.89
81910.02
41815.21
36503.027
62804.
73144.07
95144.336 96109.86
96252.39
96249.18
97033.84
98058.13
99442.02
88459.586 92786.42
96053.164 96014.38
101030.03 102540.664 103737.98 104716.67 105623.34 106510.61
107199.984]
97626.96
101466.84
87540.8
50](https://content.bartleby.com/qna-images/question/c1c1ced3-1875-44c4-84f9-6bbf6b04c124/2d8bade6-d343-4a21-adf4-27366d63e3c6/o8iho9i_thumbnail.png)

Step by stepSolved in 3 steps

- import pandas as pd import statsmodels.formula.api as sm import matplotlib.pyplot as plt X1 = np.random.normal (0, 1, 100) X2 = X1 + 3 Y = X1 + X2 + np.random.normal(0, 1, 100) df = pd.DataFrame ({"Y": Y, "X1": X1, "X2": X2}) linmodel = sm.ols(formula "Y X1 + X2", data df).fit() linmodel.summary() Output: Intercept -0.0272 X1 1.1074 X2 1.0257 Warnings: [1] Standard Errors assume that the covariance matrix of the errors is correctly specified. [2] The smallest eigenvalue is 3.27e-31. This might indicate that there are strong multicollinearity problems or that the design matrix is singular. Which of the following statements are correct? a) Perfectly correlated regressors X1 and x2 are used. b) Either X1 or X2 should be excluded, as the second regressor does not add any information to the model. 6. c) The second warning indicates that the model fit to the data is perfect.arrow_forwardThe second picture is the code. I want the output of the code to look like picture one. What part do I have to fix? THX!arrow_forwardHello! I need some help with my Java homework. Please use Eclipse Please add comments to the to program so I can understand what the code is doing and learn Create a new Eclipse project named so as to include your name (eg smith15 or jones15). In this project, create a new package with the same name as the project. In this package, write a solution to the exercise noted below. Implement the following method that returns the maximum element in an array: public static <E extends Comparable<E>> E max(E[] list) Write a test program that generates 10 random integers, invokes this method to find the max, and then displays the random integers sorted smallest to largest and then prints the value returned from the method. Max sure the the last sorted and returned value as the same!arrow_forward
- Answer the given question with a proper explanation and step-by-step solution. Write a method that takes in an array of Point2D objects, and then analyzes the dataset to find points that are close together. Be sure to review the Point2D API. In your method, if the distance between any pair of points is less than 10, display the distance and the (x,y)s of each point. For example, "The distance between (3,5) and (8,9) is 6.40312." The complete API for the Point2D ADT may be viewed at http://www.ime.usp.br/~pf/sedgewick-wayne/algs4/documentation/Point2D.htmlLinks to an external site.. Do not compare a point to itself! Try to write your program directly from the API - do not review the ADT's source code. (Don't worry too much about your answer compiling - focus on using an API from its documentation.) Your answer should include only the method you wrote, do not write a complete program.arrow_forwardWhen I run this code it says there is an error. Can anyone help point out why I am getting an error for this code? from Artist import *from Artwork import *if __name__ == "__main__":mylist = input().split(" ")user_artist_name = str(mylist[0]+" "+mylist[1])user_birth_year = int(mylist[2])user_death_year = int(mylist[3])#mylist1 = mylist(4:-1)user_title = ""for i in mylist[4:-1]:user_title+=i+" "#user_title = str(mylist[4:-1])user_year_created = int(mylist[-1])user_artist = Artist(user_artist_name,user_birth_year,user_death_year)new_artwork = Artwork(user_title, user_year_created, user_artist)new_artwork.print_info() Here is the error message: Traceback (most recent call last): File "main.py", line 7, in <module> user_birth_year = int(mylist[2]) IndexError: list index out of rangearrow_forwardCreate an ArrayList of strings to store the names of celebrities or athletes. Add five names to the list. Process the list with a for loop and the get() method to display the names, one name per line. Pass the list to a void method. Inside the method, Insert another name at index 2 and remove the name at index 4. Use a foreach loop to display the arraylist again, all names on one line separated by asterisks. After the method call in main, create an iterator for the arraylist and use it to display the list one more time.arrow_forward
- mport tkinter as tkfrom tkinter import ttkroot = tk.Tk()root.title("Escape from the Maze")root.geometry("600x300")frame = ttk.Frame(root, padding="200 100 200 100")frame.pack(fill=tk.BOTH, expand=True)def click_button1(): root.title("Wrong way!")def click_button2(): root.destroy()button1 = ttk.Button(frame, text="Go Left", command=click_button1)button2 = ttk.Button(frame, text="Go Right", command=click_button2)button1.pack()button2.pack()root.mainloop() Refer to Code Example 18-1: How big is the window that this code displays? a. 600 pixels wide by 300 pixels high b. 600 characters wide by 300 characters high c. 200 pixels wide by 100 pixels high d. 200 characters wide by 100 characters higharrow_forwardI am attempting to write a code that will allow me to loop through a series of FITS fies in a folder on my Desktop. This is what I have so far, but no plot as yet. I have included a picture of what the plot should look like after the looping process. import numpy as npimport matplotlib.pyplot as pltimport globfrom astropy.io import fitsfrom astropy.wcs import WCSfrom pathlib import Path%matplotlib inline%matplotlib widget plt.figure(figsize=(5,5))legends = [] def plot_fits_file(file_path): # used this data to test ---------- # lam = np.random.random(100) # flux = np.random.random(100) # -------------------- # below code will work when you have file # all the plot will be on single chart hdul = fits.open(file_path) data = hdul[1].data h1 = hdul[1].header flux = data[1] w = WCS(h1, naxis=1, relax=False, fix=False) lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0] plt.plot(lam, flux) plt.ylim(0, ) plt.xlabel('RV[km/s]')…arrow_forwardIn JAVA, use a HashMap to count and store the frequency counts for all the words from a large text document. Using file util, please. Then, display the contents of this HashMap with words and frequency count. Next, please create a set view of the Map and store the contents in an array. Sort this array based on key value and display it. Finally, sort the array in decreasing order by frequency and display it as well. Please label your explanation in the code Thank youarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
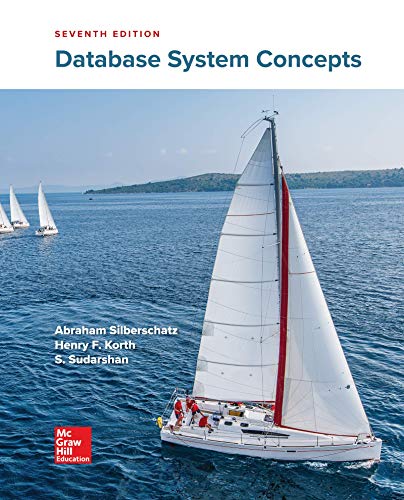
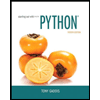
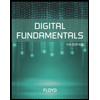
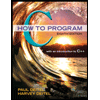
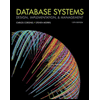
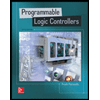