I need is a short summary of the steps taken when writing the code below. Nothing too complicated just explain the steps in order. package gradleproject2; import javafx.application.Application; import javafx.collections.FXCollections; import javafx.event.Event; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.ComboBox; import javafx.scene.control.Label; import javafx.scene.control.TextField; import javafx.scene.layout.Pane; import javafx.stage.Stage; /** * The application take in the amout in Omani Rails * And converts ut to an amount to the country of your choice; * Only 30 countries have been provide in this case and can be seen in the enum called * countries */ public class Main extends Application { @Override public void start(Stage appStage) throws Exception { //open stage Scene scene = new Scene(new Main().dataCollectionDialogue(),473,315); appStage.setScene(scene); appStage.setTitle("Currency converter"); appStage.show();//launch the application } public Pane dataCollectionDialogue(){ Pane pane = new Pane(); Label label = new Label("From Omani Rials Currency converter"); label.setLayoutY(22); label.setLayoutX(120); TextField amount = new TextField(); amount.setPromptText("Enter the amount to convert"); amount.setLayoutY(69); amount.setLayoutX(133); amount.setPrefWidth(100); Button btn = new Button("Convert"); btn.setLayoutY(270); btn.setLayoutX(195); ComboBox countriesComboBox = new ComboBox<>(); //Add countries to the combo box countriesComboBox.setItems(FXCollections.observableArrayList(countries.values())); countriesComboBox.setPromptText("Select a Country"); countriesComboBox.setLayoutY(165); countriesComboBox.setLayoutX(133); //create action event for the button btn.setOnAction(event -> { //Get selected country name String countryName= countriesComboBox.getValue().toString(); //Get amount to convert double m = Double.parseDouble(amount.getText()); double convert = currencyCalculator(countryName, m); String message = "The converted amount is : "+convert; Stage stage = new Stage(); Scene scene = new Scene(getConvertedAmountWindow(message)); stage.setScene(scene); stage.setTitle("results"); stage.show(); }); pane.getChildren().addAll(label, amount,btn,countriesComboBox); return pane; } /** * Create the converted amount display window */ private Pane getConvertedAmountWindow( String toDisplay){ Pane pane = new Pane(); pane.setPrefSize(473, 117); Label label = new Label(); label.setLayoutX(121); label.setLayoutY(50); label.setText(toDisplay); pane.getChildren().addAll(label); return pane; } /** * The method does our currency conversion * @return calculated amount */ private double currencyCalculator(String country, double amountTocConvert){ double amount = 0; //currency conversion selection process switch (country){ case "Kuwaiti_Dinar": amount = amountTocConvert/0.80; break; case "Kenyan_Shilling": amount = amountTocConvert/282.7; break; case "Lebanese_Pound": amount = amountTocConvert/3928.10; break; case "Liberian_Dollar": amount = amountTocConvert/513.96; break; case "Libya_Dinar": amount = amountTocConvert/3.57; break; case "Lisk": amount = amountTocConvert/2.33; break; case "Jamaican_Dollar": amount = amountTocConvert/367.85; break; case "Jersey_Pound": amount = amountTocConvert/2.01; break; case "japanese_Yen": amount = amountTocConvert/275.44; break; case "Korean_Won": amount = amountTocConvert/3021.29; break; case "Lao_Kip": amount = amountTocConvert/24040.13; break; case "Algerian_Dinar": amount = amountTocConvert/336.44; break; case "Argentina_Peso": amount = amountTocConvert/200.96; break; case "Ardor": amount = amountTocConvert/54.35; break; case "Augur": amount = amountTocConvert/0.19; break; case "Australian_Dollar": amount = amountTocConvert/3.65; break; case "Bahamian_Dollar": amount = amountTocConvert/2.60; break; case "Bahraini_Dinar": amount = amountTocConvert/0.98; break; case "Bangladeshi_Taka": amount = amountTocConvert/221.04; break; case "Belize_Dollar": amount = amountTocConvert/5.21; break; case "Prime_coin": amount = amountTocConvert/37.63; break; case "Qatari_Riyal": amount = amountTocConvert/9.49; break; case "Qtum": amount = amountTocConvert/1.15; break; case "Quark_coin": amount = amountTocConvert/92.21; break; case "Ripple": amount = amountTocConvert/10.46; break; case "Romanian_Leu": amount = amountTocConvert/10.8; break; case "Russian_Rubie": amount = amountTocConvert/203.46; break; case "Samoa_Tala": amount = amountTocConvert/6.95; break; case "Saudi_Arabian_Riyal": amount = amountTocConvert/9.77; } return amount; } //Hold the name of the countries enum countries{ Kuwaiti_Dinar, Kenyan_Shilling, Lebanese_Pound, Liberian_Dollar, Libya_Dinar, Lisk, Jamaican_Dollar, Jersey_Pound, japanese_Yen, Korean_Won, Lao_Kip, Algerian_Dinar, Argentina_Peso, Ardor, Augur, Australian_Dollar, Bahamian_Dollar, Bahraini_Dinar, Bangladeshi_Taka, Belize_Dollar, Prime_coin, Qatari_Riyal, Qtum, Quark_coin, Ripple, Romanian_Leu, Russian_Rubie, Samoa_Tala, Saudi_Arabian_Riyal } }
I need is a short summary of the steps taken when writing the code below. Nothing too complicated just explain the steps in order.
package gradleproject2;
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.event.Event;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ComboBox;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.Pane;
import javafx.stage.Stage;
/**
* The application take in the amout in Omani Rails
* And converts ut to an amount to the country of your choice;
* Only 30 countries have been provide in this case and can be seen in the enum called
* countries
*/
public class Main extends Application {
@Override
public void start(Stage appStage) throws Exception {
//open stage
Scene scene = new Scene(new Main().dataCollectionDialogue(),473,315);
appStage.setScene(scene);
appStage.setTitle("Currency converter");
appStage.show();//launch the application
}
public Pane dataCollectionDialogue(){
Pane pane = new Pane();
Label label = new Label("From Omani Rials Currency converter");
label.setLayoutY(22);
label.setLayoutX(120);
TextField amount = new TextField();
amount.setPromptText("Enter the amount to convert");
amount.setLayoutY(69);
amount.setLayoutX(133);
amount.setPrefWidth(100);
Button btn = new Button("Convert");
btn.setLayoutY(270);
btn.setLayoutX(195);
ComboBox<countries> countriesComboBox = new ComboBox<>();
//Add countries to the combo box
countriesComboBox.setItems(FXCollections.observableArrayList(countries.values()));
countriesComboBox.setPromptText("Select a Country");
countriesComboBox.setLayoutY(165);
countriesComboBox.setLayoutX(133);
//create action event for the button
btn.setOnAction(event -> {
//Get selected country name
String countryName= countriesComboBox.getValue().toString();
//Get amount to convert
double m = Double.parseDouble(amount.getText());
double convert = currencyCalculator(countryName, m);
String message = "The converted amount is : "+convert;
Stage stage = new Stage();
Scene scene = new Scene(getConvertedAmountWindow(message));
stage.setScene(scene);
stage.setTitle("results");
stage.show();
});
pane.getChildren().addAll(label, amount,btn,countriesComboBox);
return pane;
}
/**
* Create the converted amount display window
*/
private Pane getConvertedAmountWindow( String toDisplay){
Pane pane = new Pane();
pane.setPrefSize(473, 117);
Label label = new Label();
label.setLayoutX(121);
label.setLayoutY(50);
label.setText(toDisplay);
pane.getChildren().addAll(label);
return pane;
}
/**
* The method does our currency conversion
* @return calculated amount
*/
private double currencyCalculator(String country, double amountTocConvert){
double amount = 0;
//currency conversion selection process
switch (country){
case "Kuwaiti_Dinar":
amount = amountTocConvert/0.80;
break;
case "Kenyan_Shilling":
amount = amountTocConvert/282.7;
break;
case "Lebanese_Pound":
amount = amountTocConvert/3928.10;
break;
case "Liberian_Dollar":
amount = amountTocConvert/513.96;
break;
case "Libya_Dinar":
amount = amountTocConvert/3.57;
break;
case "Lisk":
amount = amountTocConvert/2.33;
break;
case "Jamaican_Dollar":
amount = amountTocConvert/367.85;
break;
case "Jersey_Pound":
amount = amountTocConvert/2.01;
break;
case "japanese_Yen":
amount = amountTocConvert/275.44;
break;
case "Korean_Won":
amount = amountTocConvert/3021.29;
break;
case "Lao_Kip":
amount = amountTocConvert/24040.13;
break;
case "Algerian_Dinar":
amount = amountTocConvert/336.44;
break;
case "Argentina_Peso":
amount = amountTocConvert/200.96;
break;
case "Ardor":
amount = amountTocConvert/54.35;
break;
case "Augur":
amount = amountTocConvert/0.19;
break;
case "Australian_Dollar":
amount = amountTocConvert/3.65;
break;
case "Bahamian_Dollar":
amount = amountTocConvert/2.60;
break;
case "Bahraini_Dinar":
amount = amountTocConvert/0.98;
break;
case "Bangladeshi_Taka":
amount = amountTocConvert/221.04;
break;
case "Belize_Dollar":
amount = amountTocConvert/5.21;
break;
case "Prime_coin":
amount = amountTocConvert/37.63;
break;
case "Qatari_Riyal":
amount = amountTocConvert/9.49;
break;
case "Qtum":
amount = amountTocConvert/1.15;
break;
case "Quark_coin":
amount = amountTocConvert/92.21;
break;
case "Ripple":
amount = amountTocConvert/10.46;
break;
case "Romanian_Leu":
amount = amountTocConvert/10.8;
break;
case "Russian_Rubie":
amount = amountTocConvert/203.46;
break;
case "Samoa_Tala":
amount = amountTocConvert/6.95;
break;
case "Saudi_Arabian_Riyal":
amount = amountTocConvert/9.77;
}
return amount;
}
//Hold the name of the countries
enum countries{
Kuwaiti_Dinar,
Kenyan_Shilling,
Lebanese_Pound,
Liberian_Dollar,
Libya_Dinar,
Lisk,
Jamaican_Dollar,
Jersey_Pound,
japanese_Yen,
Korean_Won,
Lao_Kip,
Algerian_Dinar,
Argentina_Peso,
Ardor,
Augur,
Australian_Dollar,
Bahamian_Dollar,
Bahraini_Dinar,
Bangladeshi_Taka,
Belize_Dollar,
Prime_coin,
Qatari_Riyal,
Qtum,
Quark_coin,
Ripple,
Romanian_Leu,
Russian_Rubie,
Samoa_Tala,
Saudi_Arabian_Riyal
}
}

Step by step
Solved in 2 steps

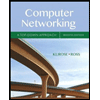
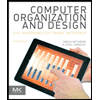
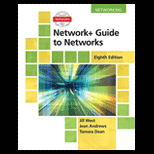
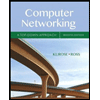
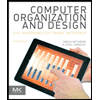
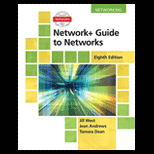
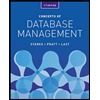
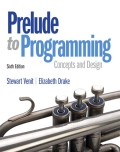
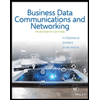