I need help with this Java program over a Cell class program shown below detailed in the given image: This is the Java code (Cell class)that need fixed with help of both the given image and the interface guide - package classPackage; import java.util.ArrayList; import interfaces.CellInterface; import interfaces.RatInterface; /** * @author Owner * */ public class Cell implements CellInterface { privatefinalint [] location; private ArrayList ratHouse = new ArrayList (); public Cell(int[] pLocation) { location = pLocation.clone(); } @Override publicchar getCellType() { // TODO Auto-generated method stub return 0; } @Override publicint[] receiveRat(RatInterface pRat) { pRat.wearDown(); if (Math.random()<.2) pRat.refresh(); ratHouse.add(pRat); returnlocation.clone(); } @Override public RatInterface retrieveRat() { /** * The storeTheDead(Rat pRat) method stores the argument Rat in a list of dead rats. * precondition: pRat must reference a Rat object, a list structure of some kind that stores rats, must exist. * postcondition: pRat's reference is added to a list of dead rats. * @param pRat */ returnratHouse; } @Override publicvoid storeTheDead(RatInterface pRat) { /** * The returnTheDead() method retrieves a list of the rats that died in the cell. * @precondition The dead rat ArrayList must exist though it need not have any rats in it. * @postcondition none * @return * ArrayList<> of Rat objects. */ } @Override public ArrayList returnTheDead() { // TODO Auto-generated method stub returnnull; } } The interface code that has directions that needs to be inputted in the cell class code - package interfaces; import java.util.ArrayList; /** * @author Owner * */ public interface CellInterface { int[] location = newint[2]; /** * The getCellType() returns the cell type * @Precondition The cell type must be initialized as a final variable during the construction of a cell object. * It should indicate one of the four cell types Cell, Hole, Waste, or Shelter * @Postcondition no change * @return a character for the cell type */ publicchar getCellType(); /** * The receiveRat() method receives a rat and stores the rat * @param RatInterface holds reference to Rat object * @return * Returns {row,col} * cell location if rat is accepted and stored * -1, -1 if there is no space to hold the rat * */ publicint[] receiveRat(RatInterface pRat); /** * The retrieveRat(String pRatID) method receives a rat ID and returns the reference to that rat if the rat is stored in the cell * @param RatID holds String ID of Rat object * @return Returns the reference to a Rat object if there is one in the cell */ public RatInterface retrieveRat(); /** * The storeTheDead(Rat pRat) method stores the argument Rat in a list of dead rats. * precondition: pRat must reference a Rat object, a list structure of some kind that stores rats, must exist. * postcondition: pRat's reference is added to a list of dead rats. * @param pRat */ publicvoid storeTheDead(RatInterface pRat); /** * The returnTheDead() method retrieves a list of the rats that died in the cell. * @precondition The dead rat ArrayList must exist though it need not have any rats in it. * @postcondition none * @return * ArrayList<> of Rat objects. */ public ArrayList returnTheDead(); }
I need help with this Java program over a Cell class program shown below detailed in the given image:
This is the Java code (Cell class)that need fixed with help of both the given image and the interface guide -
package classPackage;
import java.util.ArrayList;
import interfaces.CellInterface;
import interfaces.RatInterface;
/**
* @author Owner
*
*/
public class Cell implements CellInterface {
privatefinalint [] location;
private ArrayList <RatInterface> ratHouse = new ArrayList <RatInterface>();
public Cell(int[] pLocation) {
location = pLocation.clone();
}
@Override
publicchar getCellType() {
// TODO Auto-generated method stub
return 0;
}
@Override
publicint[] receiveRat(RatInterface pRat) {
pRat.wearDown();
if (Math.random()<.2)
pRat.refresh();
ratHouse.add(pRat);
returnlocation.clone();
}
@Override
public RatInterface retrieveRat() {
/**
* The storeTheDead(Rat pRat) method stores the argument Rat in a list of dead rats.
* precondition: pRat must reference a Rat object, a list structure of some kind that stores rats, must exist.
* postcondition: pRat's reference is added to a list of dead rats.
* @param pRat
*/
returnratHouse;
}
@Override
publicvoid storeTheDead(RatInterface pRat) {
/**
* The returnTheDead() method retrieves a list of the rats that died in the cell.
* @precondition The dead rat ArrayList must exist though it need not have any rats in it.
* @postcondition none
* @return
* ArrayList<> of Rat objects.
*/
}
@Override
public ArrayList<RatInterface> returnTheDead() {
// TODO Auto-generated method stub
returnnull;
}
}
The interface code that has directions that needs to be inputted in the cell class code -
package interfaces;
import java.util.ArrayList;
/**
* @author Owner
*
*/
public interface CellInterface
{
int[] location = newint[2];
/**
* The getCellType() returns the cell type
* @Precondition The cell type must be initialized as a final variable during the construction of a cell object.<br>
* It should indicate one of the four cell types Cell, Hole, Waste, or Shelter
* @Postcondition no change
* @return a character for the cell type
*/
publicchar getCellType();
/**
* The receiveRat() method receives a rat and stores the rat
* @param RatInterface holds reference to Rat object
* @return
* Returns {row,col}<br>
* cell location if rat is accepted and stored<br>
* -1, -1 if there is no space to hold the rat
*
*/
publicint[] receiveRat(RatInterface pRat);
/**
* The retrieveRat(String pRatID) method receives a rat ID and returns the reference to that rat if the rat is stored in the cell
* @param RatID holds String ID of Rat object
* @return Returns the reference to a Rat object if there is one in the cell
*/
public RatInterface retrieveRat();
/**
* The storeTheDead(Rat pRat) method stores the argument Rat in a list of dead rats.
* precondition: pRat must reference a Rat object, a list structure of some kind that stores rats, must exist.
* postcondition: pRat's reference is added to a list of dead rats.
* @param pRat
*/
publicvoid storeTheDead(RatInterface pRat);
/**
* The returnTheDead() method retrieves a list of the rats that died in the cell.
* @precondition The dead rat ArrayList must exist though it need not have any rats in it.
* @postcondition none
* @return
* ArrayList<> of Rat objects.
*/
public ArrayList<RatInterface> returnTheDead();
}


Step by step
Solved in 4 steps with 1 images

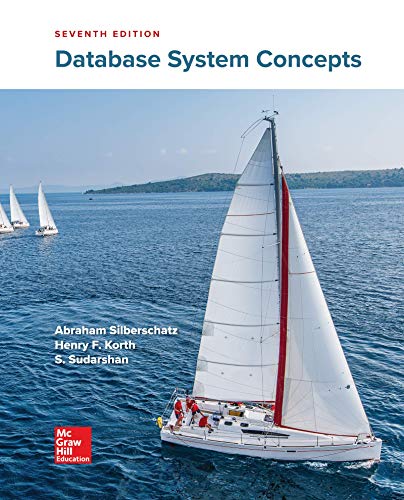
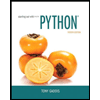
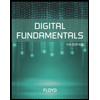
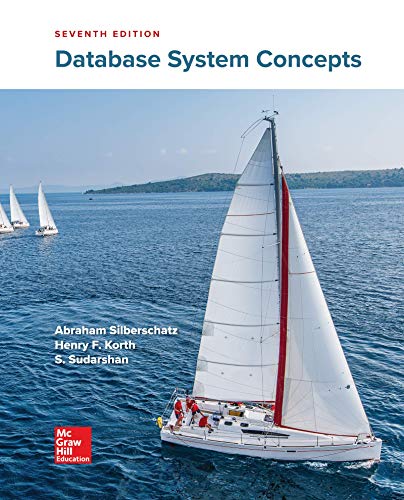
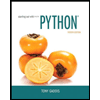
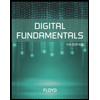
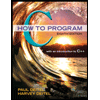
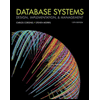
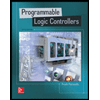