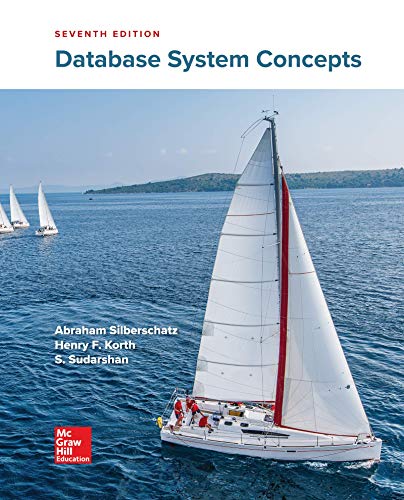
I need help with this C assignment, I've been stuck on!!
I have attached the assignment.
It declares that the files merge.c, mergesort.h, and wrt.c CANNOT be changed
Please follow the assignment to further complete all else thats required!
Merge.c [Don't make changes]
#include "mergesort.h"
void merge(int a[], int b[], int c[], int m, int n)
{
int i = 0, j = 0, k = 0;
/* Loop through both arrays and compare their elements to eachother */
while (i < m && j < n)
if (a[i] < b[j])
c[k++] = a[i++];
else
c[k++] = b[j++];
/* If there are any remaining elements in array b[]. copy them to array c[]. */
while (i < m) /* pick up any remainder */
c[k++] = a[i++];
/* If there are any remaining elements in array a[], copy them to array c[]. */
while (j < n)
c[k++] = b[j++];
}
Mergesort.h[Don't make changes]
#include <assert.h>
#include <stdio.h>
#include <stdlib.h>
// This function merges two sorted arrys a and b into a sorted array c.
// paterameters: a - c sorts its array. m: is the elements in a n: is the number of elements in b
void merge(int a[], int b[], int c[], int m, int n);
//This function sorts an array of integers using the merge sort
//key: the array of integers to be sorted
//n: the number of elements in the array
void mergesort(int key[], int n);
//This function writes an array of integers
//key: the array of integers to be written
//sz: the number of elements in the array
void wrt(int key [], int sz);
Wrt.c [Don't make changes]
#include "mergesort.h"
/* Print out the elements of an array of integers.
key: the array to be printed.
sz: the number of elements in the array. */
void wrt(int key[], int sz)
{
int i;
for (i=0; i < sz; ++i)
printf("%4d%s", key[i], ((i < sz - 1)? "" : "\n"));
}
[KINDLY, PLEASE Check the makefile, sort158a.c and merge.c if it needs change, please do so, make sure that the file will execute the "make" command and NO ERRORS DISPLAY]
Ex.
sort158a.c
#include "mergesort.h"
int main(void)
{
int sz, key[] = { 4, 3, 1, 67, 55, 8, 0, 4,
-5, 37, 7, 4, 2, 9, 1, -1 };
sz = sizeof(key) / sizeof(int); /* the size of key[] */
wrt(key, sz); /* Print the contents of the orginal arry */
mergesort(key, sz); /* Sort the array using mergesort */
printf("After mergesort:"\n); /*prints "After mergesort:"*/
wrt(key, sz); // Print the contents of the sorted array
return 0;
}
MERGE.C
#include "mergesort.h"
void merge(int a[], int b[], int c[], int m, int n)
{
int i = 0, j = 0, k = 0;
/* Loop through both arrays and compare their elements to eachother */
while (i < m && j < n)
if (a[i] < b[j])
c[k++] = a[i++];
else
c[k++] = b[j++];
/* If there are any remaining elements in array b[]. copy them to array c[]. */
while (i < m) /* pick up any remainder */
c[k++] = a[i++];
/* If there are any remaining elements in array a[], copy them to array c[]. */
while (i < m) /* pick up any remainder */
c[k++] = a[i++];
while (j < n)
c[k++] = b[j++];
}
MAKEFILE
sort158: main.o merge.o mergesort.o wrt.o
gcc -o sort158 main.o merge.o mergesort.o wrt.o -lm
main.o: main.c mergesort.h
gcc -c main.c
merge.o: merge.c mergesort.h
gcc -c merge.c
mergesort.o: mergesort.c mergesort.h
gcc -c mergesort.c
wrt.o: wrt.c
gcc -c wrt.c
clean:
rm *.o sort158

Step by stepSolved in 5 steps with 2 images

- //inserts e at index ind publicvoidadd(intind,Te){ //if index is invalid display a message and return //valid index are 0 to size //push all elements up by one all the down to index ind (stop at index ind) //insert e at index ind //increase the size }arrow_forwardGDB and Getopt Class Activity activity [-r] -b bval value Required Modify the activity program from last week with the usage shown. The value for bval is required to be an integer as is the value at the end. In addition, there should only be one value. Since everything on the command line is read in as a string, these now need to be converted to numbers. You can use the function atoi() to do that conversion. You can do the conversion in the switch or you can do it at the end of the program. The number coming in as bval and the value should be added together to get a total. That should be the only value printed out at the end. Total = x should be the only output from the program upon success. Remove all the other print statements after testing is complete. Take a screenshot of the output to paste into a Word document and submit. Practice Compile the program with the -g option to load the symbol table. Run the program using gdb and use watch on the result so the program stops when the…arrow_forwardC++ You will write the code to resize a hash table. Modify the code below. Increase the hash table size from 10 to 50. Write a function to calculate the greatest number of key-value pairs in any bucket in the hash table. Write a function to resize the hash table. Calculate the size of the resized hash table by selecting the next prime number that is greater than or equal to the maximum number of buckets in the original hash table multiplied by 2. (next prime number ≥ (N * 2)). Resize when any list in any bucket contains more than four key-value pairs. Update the control function to randomly generate 200 key-value pairs and add them to the hash table. If any bucket contains more than four key-value pairs, resize the hash table. Print the hash table before it is resized and after it is resized. using namespace std; class HashTable{ private: static int const BUCKET_CNT = 10; list<pair<int, string>> bucket[BUCKET_CNT]; public: bool isEmpty(); int hashFunction(int key); void…arrow_forward
- Pls code use c++ and provide explanation about the hash function usedarrow_forwardCampground reservations, part 3: Available campsites Excellent job! This next one's a little more challenging. Which campsites are available to reserve? We want to be able to quickly find which campsites are available. Can you help us? Given a campgrounds array, return an array with the campsite numbers of all currently unreserved (isReserved === false) campsites. Call the function availableCampsites. Dataset As a reminder, our data looks like this. It's an array with a bunch of campsite objects in it. Here is a small subset of the data to give you an idea: const campgrounds = [ { number: 1, view: "ocean", partySize: 8, isReserved: false }, { number: 5, view: "ocean", partySize: 4, isReserved: false }, { number: 12, view: "ocean", partySize: 4, isReserved: true }, { number: 18, view: "forest", partySize: 4, isReserved: false }, { number: 23, view: "forest", partySize: 4, isReserved: true }, ];arrow_forwardWrite a program that opens a specified text file then displays a list of all the unique words found in the file. Hint: Store each word as an element of a set. 5. Word Frequency Write a program that reads the contents of a text file. The program should create a dictio- nary in which the keys are the individual words found in the file and the values are the number of times each word appears. For example, if the word "the" appears 128 times, the dictionary would contain an element with 'the' as the key and 128 as the value. Thearrow_forward
- Please help write the functions (context for the problem in the images): write_X_to_map(filename, row, col): Takes as inputs a string corresponding to a filename, and two non-negative integers representing a row and column index. Reads in the map at the given filename, inserts an X into the given row and column position, then saves the map to a new file with 'new_' prepended to the given filename. You can assume the filename given to the function as argument refers to a file that exists.arrow_forwardtionary.java Overview You and a partner will create a program that will compete against another program created by a pair of your fellow classmates in a game that generally follows the rules of Ghost. The game will consist of two programs that will take turns adding letters to a growing word fragment, trying not to be the one to complete a valid word. Each fragment must be the beginning of an actual word. The program that completes a word that has more than four characters loses. The program that creates a word fragment that has no possible chance of creating a word loses. Programmatic Rules Your program will compete against another program, the programs will communicate with each other by reading and writing from a shared file on the hard drive. Each program will take turns adding a letter to an ever-growing word fragment in the shared file. Before adding a letter to the file your program should figure out what the next best letter to play is. It should maintain the current word…arrow_forwardC++ Complete the program that will allow the user to enter data on 10 Halloween costumes. The program will create Costume objects and then store the Costume objects in a Hash Table. The Costume ID will be the key and a pointer to the Costume object will be the value. You are given Lab7.cpp, HashEntry.h, and Costume.h and you should not have to make any changes to these files at all. You will need to write HashTable.h, which implements the HashTable class. This should NOT be a template class. Your HashTable class should resolve collisions by linear probing and should use the HashEntry class for the linkedlist nodes. Because we’re using probing, there is no removal function. This is okay for this application, since we want to keep a record of costumes that are no longer being kept in stock. ---GIVEN--- Lab.cpp #include <iostream> #include "HashTable.h" #include "Costume.h" using namespace std; int main() { int size; int key; Costume *newCostume; string name; float price;…arrow_forward
- Create a file called toArray.js that reads n strings from the command line, adds them to an array, and returns them in order that they were entered. You must use a forEach loop for this file. The output will contain the size of the array and the array itself.arrow_forward- Write a line of code to look at the first few rows of the DataFrame in Python import csv # take a look at the datasurvey = pd.read_csv('https://raw.githubusercontent.com/fivethirtyeight/data/master/steak-survey/steak-risk-survey.csv') # YOUR CODE HEREarrow_forwardplease provide code and explanation with output (list student)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
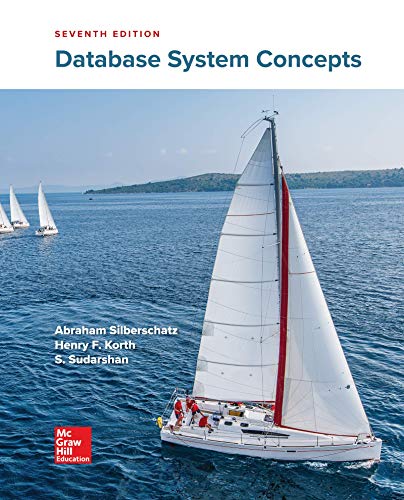
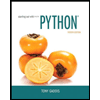
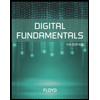
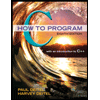
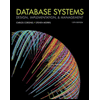
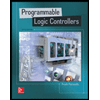