I need help with a Java question so that it can output them described in the image below: import java.util.Scanner; public class LabProgram { public static void sortArray(int[] myArr, int arrSize) { for (int i = 0; i < arrSize - 1; i++) { for (int j = 0; j < arrSize - i - 1; j++) { if (myArr[j] < myArr[j + 1]) { // Swap elements if they are in the wrong order int temp = myArr[j]; myArr[j] = myArr[j + 1]; myArr[j + 1] = temp; } } } } public static void main(String[] args) { Scanner scanner = new Scanner(System.in); // Read the number of elements in the array int arrSize = scanner.nextInt(); int[] myArr = new int[arrSize]; // Read and populate the array with elements for (int i = 0; i < arrSize; i++) { myArr[i] = scanner.nextInt(); } // Call the sorting method sortArray(myArr, arrSize); // Output the sorted array for (int i = 0; i < arrSize; i++) { System.out.print(myArr[i]); if (i < arrSize - 1) { System.out.print(","); } } System.out.println(); } }
I need help with a Java question so that it can output them described in the image below:
import java.util.Scanner;
public class LabProgram {
public static void sortArray(int[] myArr, int arrSize) {
for (int i = 0; i < arrSize - 1; i++) {
for (int j = 0; j < arrSize - i - 1; j++) {
if (myArr[j] < myArr[j + 1]) {
// Swap elements if they are in the wrong order
int temp = myArr[j];
myArr[j] = myArr[j + 1];
myArr[j + 1] = temp;
}
}
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Read the number of elements in the array
int arrSize = scanner.nextInt();
int[] myArr = new int[arrSize];
// Read and populate the array with elements
for (int i = 0; i < arrSize; i++) {
myArr[i] = scanner.nextInt();
}
// Call the sorting method
sortArray(myArr, arrSize);
// Output the sorted array
for (int i = 0; i < arrSize; i++) {
System.out.print(myArr[i]);
if (i < arrSize - 1) {
System.out.print(",");
}
}
System.out.println();
}
}
![### Test Case Analysis: Sorting Function
This page provides an analysis of test cases for a sorting function, focusing on discrepancies between the actual output and expected results.
#### Test Case 1
- **Input**: `[5, 10, 4, 39, 12, 2]`
- **Your output**: `[39, 12, 10, 4, 2]`
- **Expected output**: `[39, 12, 10, 4, 2, ]`
**Issue**: The output is missing a trailing comma.
---
#### Test Case 2
- **Input**: `[3, 99, 3, 27]`
- **Your output**: `[99, 27, 3]`
- **Expected output**: `[99, 27, 3, ]`
**Issue**: The output is missing a trailing comma.
---
#### Test Case 3
- **Input**: `[1, 25]`
- **Your output**: `[25]`
- **Expected output**: `[25, ]`
**Issue**: The output is missing a trailing comma.
---
### Unit Test Analysis
- **Function call**: `sortArray([61, 92, 0, 22])`
- **Test feedback**:
- `sortArray([61, 92, 0, 22]) correctly returned [92, 61, 22, 0, ]`
The function executed successfully for this input, producing the correct result with the trailing comma included.
---
### Conclusion
The discrepancies highlighted indicate a consistent issue with missing trailing commas in the function's output. Adjustments are necessary to ensure that the output format matches the expected results consistently across tests.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4e135d5c-f867-4afd-9f0d-b505f4f19664%2F2379047b-6adc-4ac5-8904-cf2ab0555fa2%2F6c132r9_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

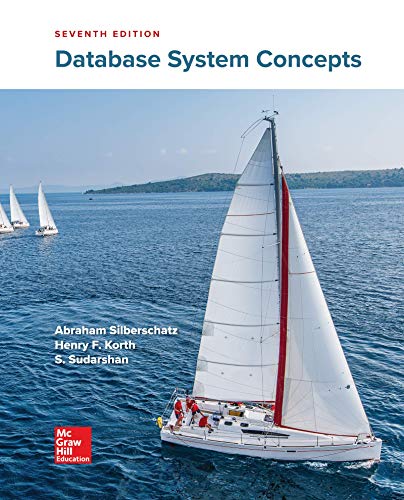
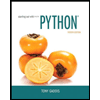
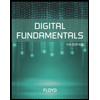
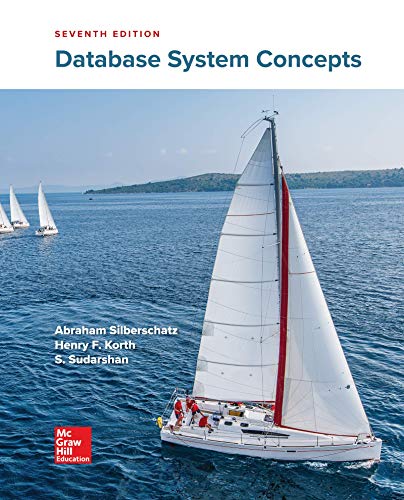
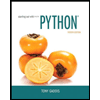
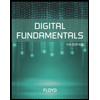
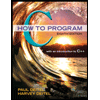
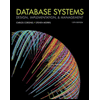
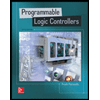