I have to implement a SubstringGenerator (class) that generates all substrings of a string recursively. For example, the substrings of the string “rum” are the seven strings “rum”, “ru”, “r”, “um”, “u”, “m”, “”
I have to implement a SubstringGenerator (class) that generates all substrings of a string recursively. For example, the substrings of the string “rum” are the seven strings
“rum”, “ru”, “r”, “um”, “u”, “m”, “”
Hint: First enumerate all substrings that start with the first character. There are n of them if the string has length n. Then enumerate the substrings of the string that you obtain by removing the first character.
Here is what your output should look like after your project is completed. (The order of your substrings is not important, if your generator produces all substrings correctly).
Substrings of "ab"
Actual: '' 'b' 'a' 'ab'
Expected: '' 'b' 'a' 'ab'
Substrings of "abc"
Actual: '' 'c' 'b' 'bc' 'a' 'ab' 'abc'
Expected: '' 'c' 'b' 'bc' 'a' 'ab' 'abc'
Substrings of "abc123"
Actual: '' '3' '2' '23' '1' '12' '123' 'c' 'c1' 'c12' 'c123' 'b' 'bc' 'bc1' 'bc12' 'bc123' 'a' 'ab' 'abc' 'abc1' 'abc12' 'abc123'
Expected: '' '3' '2' '23' '1' '12' '123' 'c' 'c1' 'c12' 'c123' 'b' 'bc' 'bc1' 'bc12' 'bc123' 'a' 'ab' 'abc' 'abc1' 'abc12' 'abc123'.
Recursion is really confusing to me and I'm not super sure where to start. I know I need a base case (which i assume would be something to do with the length of the string?), but I don't know where to go from here. Can you help?
The problem is from Big Java 6th edition Chapter 13. Excersice 13.14

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

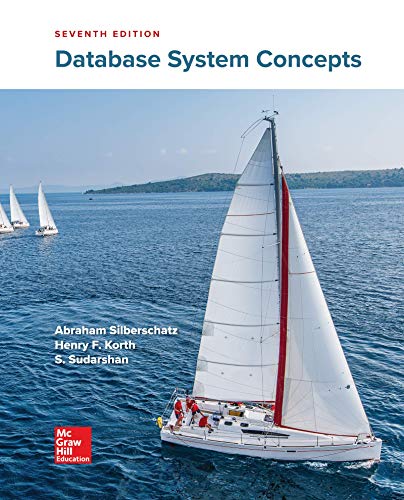
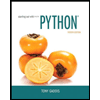
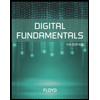
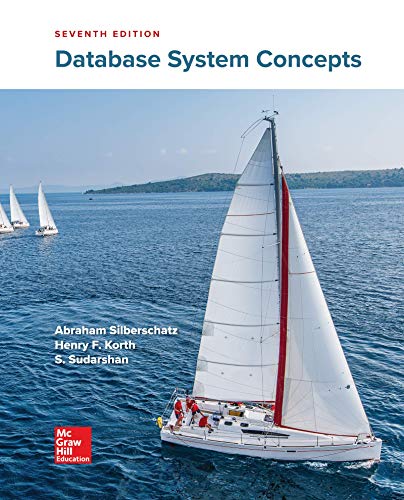
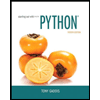
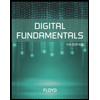
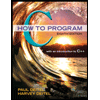
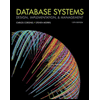
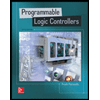