I have to create a Java program that lets the user interact with it, so they can learn about exceptions. How do I let the user interact with a list of options and direct them to a catch statement? Here is my program so far: import java.util.Scanner; import java.io.*; import java.util.InputMismatchException; public class Exceptions{ public static void main(String[] args)throws IOException{ // declare variables char option = 'y'; //Arithhmetic & Array TEST int array[] = {10,20,30,40}; int num1 = 50; int num2 = 10; // Setting up scanner to get user data Scanner exc = new Scanner(System.in); // Main method (menu, try-catch block) // all within a loop try { } // I want to learn how an ArrayIndexOutOfBoundsException... catch(ArrayIndexOutOfBoundsException exception) { System.out.println("This exception is thrown when the array index that was entered is out of bounds."); } // I want to learn how an ArithhmeticException...(mathematics) catch(ArithmeticException exception) { System.out.println("This exception is thrown when an exceptional condition has occurred in an arithmetic operation 0"); } // I want to learn how an FileNotFoundException... catch(FileNotFoundException exception) { System.out.println("This exception is thrown when a file is not accessible or does not open. (ex. the file does not exist or is located in a folder the program doesn't have access to)."); } // I want to learn how an InputMismatchException... catch(InputMismatchException exception) { System.out.println("This exception is thrown when the input entered is not an integer, but written as the word and not the number (ex. two instead of the integer 2)."); } // I want to learn how an StringIndexOutOfBoundsException... catch(StringIndexOutOfBoundsException exception) { System.out.println("This exception is thrown when a the index entered is outside of the bounds the string was set to"); } // I want to learn how an NoSuchMethodException... catch(NoSuchMethodException exception) { System.out.println("This exception is thrown when a method does not exist or isn't recgonized from another class"); } // end of try-catch block // Display the menu and have the user try as many times as the want i++; System.out.println("Would you like to try another Unchecked Exception? (y/n)"); option = exc.next().charAt(0); // menu method while(option == 'y') { System.out.println("Enter 1 if you want to learn about ArrayIndexOutOfBoundsException"); System.out.println("Enter 2 if you want to learn about ArithmeticException"); System.out.println("Enter 3 if you want to learn about FileNotFoundException"); System.out.println("Enter 4 if you want to learn about InputMismatchException"); System.out.println("Enter 5 if you want to learn about StringIndexOutOfBoundsException"); System.out.println("Enter 6 if you want to learn about NoSuchMethodException"); } // end of main method } }
I have to create a Java program that lets the user interact with it, so they can learn about exceptions. How do I let the user interact with a list of options and direct them to a catch statement?
Here is my program so far:
import java.util.Scanner;
import java.io.*;
import java.util.InputMismatchException;
public class Exceptions{
public static void main(String[] args)throws IOException{
// declare variables
char option = 'y';
//Arithhmetic & Array TEST
int array[] = {10,20,30,40};
int num1 = 50;
int num2 = 10;
// Setting up scanner to get user data
Scanner exc = new Scanner(System.in);
// Main method (menu, try-catch block)
// all within a loop
try {
}
// I want to learn how an ArrayIndexOutOfBoundsException...
catch(ArrayIndexOutOfBoundsException exception) {
System.out.println("This exception is thrown when the array index that was entered is out of bounds.");
}
// I want to learn how an ArithhmeticException...(mathematics)
catch(ArithmeticException exception) {
System.out.println("This exception is thrown when an exceptional condition has occurred in an arithmetic operation 0");
}
// I want to learn how an FileNotFoundException...
catch(FileNotFoundException exception) {
System.out.println("This exception is thrown when a file is not accessible or does not open. (ex. the file does not exist or is located in a folder the program doesn't have access to).");
}
// I want to learn how an InputMismatchException...
catch(InputMismatchException exception) {
System.out.println("This exception is thrown when the input entered is not an integer, but written as the word and not the number (ex. two instead of the integer 2).");
}
// I want to learn how an StringIndexOutOfBoundsException...
catch(StringIndexOutOfBoundsException exception) {
System.out.println("This exception is thrown when a the index entered is outside of the bounds the string was set to");
}
// I want to learn how an NoSuchMethodException...
catch(NoSuchMethodException exception) {
System.out.println("This exception is thrown when a method does not exist or isn't recgonized from another class");
}
// end of try-catch block
// Display the menu and have the user try as many times as the want
i++;
System.out.println("Would you like to try another Unchecked Exception? (y/n)");
option = exc.next().charAt(0);
// menu method
while(option == 'y') {
System.out.println("Enter 1 if you want to learn about ArrayIndexOutOfBoundsException");
System.out.println("Enter 2 if you want to learn about ArithmeticException");
System.out.println("Enter 3 if you want to learn about FileNotFoundException");
System.out.println("Enter 4 if you want to learn about InputMismatchException");
System.out.println("Enter 5 if you want to learn about StringIndexOutOfBoundsException");
System.out.println("Enter 6 if you want to learn about NoSuchMethodException");
}
// end of main method
}
}

Step by step
Solved in 3 steps with 1 images

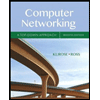
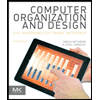
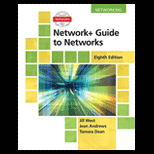
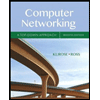
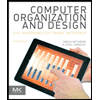
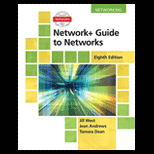
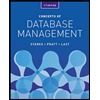
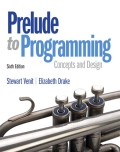
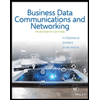