I have the basis of a program that works as an inventory, it reads the contents of a file and displays them and their quantity in three places, listed below are the things that I need to add to the program below. I've included a photo of how the program currently runs.
Contents of the file:
Contents of Inventory.txt
Red delicious apples
1.00 25 6 8 10
Assorted bouquets
4.00 50 10 10 0
Camembert cheese
2.00 25 10 12 4
END
The Program:
//inventory menu gives placement, warning, and exit option
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
struct Record {
string name;
double cost;
int markup;
int count[3];
};
const string places[3] = {"counter", "shelf", "warehouse"};
bool read_file(vector <Record> &v);
void placement (vector <Record> &v);
void warning(vector <Record> &v);
int main()
{
vector <Record> invent;
char choice;
if (read_file(invent) == false) {
return 1;
}
cout << "You have " << invent.size() << endl;
while (true)
{
cout << "(P)lacement (W)Warning (E)xit: ";
cin >> choice;
switch(choice) {
case 'P':
case 'p':
placement(invent);
break;
case 'W':
case 'w':
warning(invent);
break;
case 'E':
case 'e':
return(0);
break;
default:
cout << choice << " is not a choice\n";
}
}
return 0;
}
void placement (vector <Record> &v)
{
int which = -1;
string input;
cout << "Which? ";
cin >> input;
for (int i = 0; i < 3; i++) {
if (input.substr(0,3) == places[i].substr(0,3)) which = i;
}
if (which == -1) {
cout << "No match\n";
return;
}
cout.setf(ios::left);
cout.unsetf (ios::right);
cout.width(30);
cout << "Item";
cout.setf(ios::right);
cout.width(6);
cout << places[which] << "\n";
int j;
for (j = 0; j < v.size(); j++) {
cout.setf(ios::left);
cout.unsetf (ios::right);
cout.width(30);
cout << v[j].name;
cout.setf(ios::right);
cout.width(6);
cout << v[j].count[which] << endl;
}
}
void warning(vector <Record> &v){
for(int i=0;i<v.size();i++){
for(int j=0;j<3;j++){
if(v[i].count[j]<10){
cout<<"Warning: "<<v[i].name<<" "<<places[j]<<" "<<v[i].count[j]<<endl;
}
}
}
}
bool read_file(vector <Record> &v)
{
Record r;
ifstream infile;
infile.open("inventory.txt");
if (infile.fail()) {
cout << "can't open file\n";
return (false);
}
while (true) {
getline (infile, r.name);
cout << "Read " << r.name << endl;
if (r.name == "END") {
infile.close();
return true;
}
else {
infile >> r.cost >> r.markup;
for (int i = 0; i < 3; i++) infile >> r.count[i];
infile.get();
v.push_back(r);
}
}
return true;
}

Step by step
Solved in 2 steps with 2 images

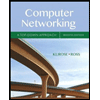
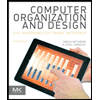
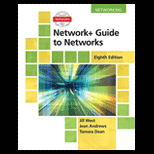
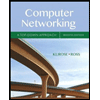
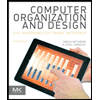
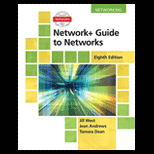
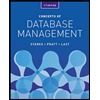
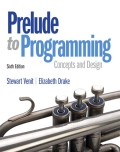
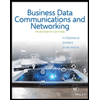