I have already written the program and have it right, but I need help write print statement for the testorder class so that the display result looks as shown in the images. I am having trouble getting that right. I will paste the program below so you can run it and attatch the image of display result. Program for Order Class: package homework; import java.util.Date; public class Order { //Declare final variables public static final int ON_ORDER = 0; public static final int CANCELLED = 1; public static final int SHIPPED = 2; //Declare local variables private int totalOrder; private String orderName; private Date date; private int status; private String shippingAddress; private String phoneNumber; private String billingAddress; //Constructor public Order(String name) { this.totalOrder += 1; this.orderName = name; this.status = ON_ORDER; this.date = new Date(); } //Cancel the placed order public void cancel() { this.status = CANCELLED; this.date = new Date(); } //Ship the placed order public void ship() { this.status = SHIPPED; this.date = new Date(); } //Set data feilds public void setShippingAddress(String a) { this.shippingAddress = a; } public void setPhoneNumber(String p) { this.phoneNumber = p; } public void setBillingAddress(String billingAddress) { this.billingAddress = billingAddress; } //Get data fields public int getTotalOrder() { return totalOrder; } public String getOrderName() { return orderName; } public Date getDate() { return date; } public int getStatus() { return status; } public String getShippingAddress() { return shippingAddress; } public String getPhoneNumber() { return phoneNumber; } public String getBillingAddress() { return billingAddress; } public String toString() { return this.orderName + "\t" + this.date + "\t" + this.status + "\t" + this.shippingAddress + "\t" + this.billingAddress + "\t" + this.phoneNumber; } }
I have already written the program and have it right, but I need help write print statement for the testorder class so that the display result looks as shown in the images. I am having trouble getting that right. I will paste the program below so you can run it and attatch the image of display result.
Program for Order Class:
package homework;
import java.util.Date;
public class Order {
//Declare final variables
public static final int ON_ORDER = 0;
public static final int CANCELLED = 1;
public static final int SHIPPED = 2;
//Declare local variables
private int totalOrder;
private String orderName;
private Date date;
private int status;
private String shippingAddress;
private String phoneNumber;
private String billingAddress;
//Constructor
public Order(String name) {
this.totalOrder += 1;
this.orderName = name;
this.status = ON_ORDER;
this.date = new Date();
}
//Cancel the placed order
public void cancel() {
this.status = CANCELLED;
this.date = new Date();
}
//Ship the placed order
public void ship() {
this.status = SHIPPED;
this.date = new Date();
}
//Set data feilds
public void setShippingAddress(String a) {
this.shippingAddress = a;
}
public void setPhoneNumber(String p) {
this.phoneNumber = p;
}
public void setBillingAddress(String billingAddress) {
this.billingAddress = billingAddress;
}
//Get data fields
public int getTotalOrder() {
return totalOrder;
}
public String getOrderName() {
return orderName;
}
public Date getDate() {
return date;
}
public int getStatus() {
return status;
}
public String getShippingAddress() {
return shippingAddress;
}
public String getPhoneNumber() {
return phoneNumber;
}
public String getBillingAddress() {
return billingAddress;
}
public String toString() {
return this.orderName + "\t" + this.date + "\t" + this.status + "\t" + this.shippingAddress + "\t"
+ this.billingAddress + "\t" + this.phoneNumber;
}
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

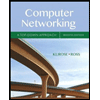
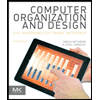
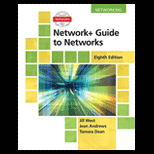
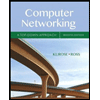
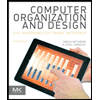
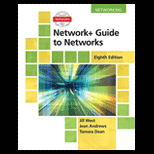
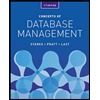
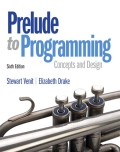
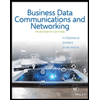