I have a code that is supposed to output whether a mobile tree is balanced or unbalanced. The input format is id w id_l d_l id_r d_r. import java.util.*; import java.io.*; public class Solution { static class Node { int id; int weight; Node leftChild; Node rightChild; int leftDistance = -1; int rightDistance = -1; public Node(int id, int weight) { this.id = id; this.weight = weight; this.leftDistance = leftDistance; this.rightDistance = rightDistance; } } // Method to read input and create tree public static Node createTree() { Scanner sc = new Scanner(System.in); TreeMap map = new TreeMap<>(); //Map parentMap = new HashMap<>(); // inputs int id, w, id_l, d_l, id_r, d_r; Node node, leftNode, rightNode; // check all nodes while (sc.hasNextInt()) { id = sc.nextInt(); w = sc.nextInt(); node = map.get(id); if (node == null) { node = new Node(id, w); map.put(id, node); } else { node.weight = w; } id_l = sc.nextInt(); d_l = sc.nextInt(); id_r = sc.nextInt(); d_r = sc.nextInt(); // leftChild input doesn't equal -1 if (id_l != -1) { leftNode = map.get(id_l); if (leftNode == null) { leftNode = new Node(id_l, w); map.put(id_l, leftNode); } node.leftChild = leftNode; node.leftDistance = d_l; node.weight = w; //parentMap.put(leftNode, node); } else { node.leftChild = null; node.leftDistance = -1; } // rightChild input doesn't = -1 if (id_r != -1) { rightNode = map.get(id_r); if (rightNode == null) { rightNode = new Node(id_r, w); map.put(id_r, rightNode); } node.rightChild = rightNode; node.rightDistance = d_r; node.weight = w; //parentMap.put(rightNode, node); } else { node.rightChild = null; node.rightDistance = -1; } } for (Map.Entry entry : map.entrySet()) { System.out.println("Node " + entry.getKey() + ": " + entry.getValue().leftDistance + " " + entry.getValue().rightDistance + " " + entry.getValue().weight); } // return root of tree return map.firstEntry().getValue(); } // Recursive method to determine if tree is balanced public static int isBalanced(Node node) { if (node == null) { return 0; } int leftWeight = isBalanced(node.leftChild); int rightWeight = isBalanced(node.rightChild); int leftTorque = node.leftDistance * leftWeight ; int rightTorque = node.rightDistance * rightWeight; //int leftTorque = (node.leftChild != null) ? leftWeight * node.leftDistance : -1; //int rightTorque = (node.rightChild != null) ? rightWeight * node.rightDistance : -1; System.out.printf("Node %d: Left distance = %d, Right distance = %d\n", node.id, node.leftDistance, node.rightDistance); System.out.printf("Node %d: Left weight = %d, Right weight = %d\n", node.id, leftWeight, rightWeight); System.out.printf("Node %d: Left torque = %d, Right torque = %d\n", node.id, leftTorque, rightTorque); //if (leftTorque != rightTorque) if (Math.abs(leftTorque - rightTorque) > 1) { return -1; } int nodeWeight = Math.max(leftWeight, rightWeight) + node.weight; System.out.printf("Node %d weight = %d\n", node.id, nodeWeight); return nodeWeight; } public static void main(String[] args) { Node root = createTree(); Map parentMap = new HashMap<>(); int result = isBalanced(root); if (result == -1) { System.out.println("Unbalanced"); } else { System.out.println("Balanced"); } } } It's not working correctly, for example the input given below is supposed to output unbalanced meaning isBalanced needs to return -1. My code keeps saying that it is balanced with a weight of 3. I wanted ask why my code is incorrect and how to fix it to work correctly. The constraints is that I have to use a TreeMap or hashMap to consctruct the tree and use a recursive method that takes one pass through the tree to determine if it is balanced. 9 3 -1 -1 -1 -1 12 14 23 15 94 5 23 1 -1 -1 -1 -1 35 1 56 10 9 20 41 5 12 10 35 20 56 2 77 1 88 1 77 2 -1 -1 -1 -1 88 2 -1 -1 -1 -1 94 3 -1 -1 -1 -1
I have a code that is supposed to output whether a mobile tree is balanced or unbalanced. The input format is id w id_l d_l id_r d_r.
import java.util.*;
import java.io.*;
public class Solution {
static class Node {
int id;
int weight;
Node leftChild;
Node rightChild;
int leftDistance = -1;
int rightDistance = -1;
public Node(int id, int weight) {
this.id = id;
this.weight = weight;
this.leftDistance = leftDistance;
this.rightDistance = rightDistance;
}
}
// Method to read input and create tree
public static Node createTree() {
Scanner sc = new Scanner(System.in);
TreeMap<Integer, Node> map = new TreeMap<>();
//Map<Node, Node> parentMap = new HashMap<>();
// inputs
int id, w, id_l, d_l, id_r, d_r;
Node node, leftNode, rightNode;
// check all nodes
while (sc.hasNextInt()) {
id = sc.nextInt();
w = sc.nextInt();
node = map.get(id);
if (node == null) {
node = new Node(id, w);
map.put(id, node);
} else {
node.weight = w;
}
id_l = sc.nextInt();
d_l = sc.nextInt();
id_r = sc.nextInt();
d_r = sc.nextInt();
// leftChild input doesn't equal -1
if (id_l != -1) {
leftNode = map.get(id_l);
if (leftNode == null) {
leftNode = new Node(id_l, w);
map.put(id_l, leftNode);
}
node.leftChild = leftNode;
node.leftDistance = d_l;
node.weight = w;
//parentMap.put(leftNode, node);
}
else {
node.leftChild = null;
node.leftDistance = -1;
}
// rightChild input doesn't = -1
if (id_r != -1) {
rightNode = map.get(id_r);
if (rightNode == null) {
rightNode = new Node(id_r, w);
map.put(id_r, rightNode);
}
node.rightChild = rightNode;
node.rightDistance = d_r;
node.weight = w;
//parentMap.put(rightNode, node);
}
else {
node.rightChild = null;
node.rightDistance = -1;
}
}
for (Map.Entry<Integer, Node> entry : map.entrySet()) {
System.out.println("Node " + entry.getKey() + ": " + entry.getValue().leftDistance + " " + entry.getValue().rightDistance + " " + entry.getValue().weight);
}
// return root of tree
return map.firstEntry().getValue();
}
// Recursive method to determine if tree is balanced
public static int isBalanced(Node node) {
if (node == null) {
return 0;
}
int leftWeight = isBalanced(node.leftChild);
int rightWeight = isBalanced(node.rightChild);
int leftTorque = node.leftDistance * leftWeight ;
int rightTorque = node.rightDistance * rightWeight;
//int leftTorque = (node.leftChild != null) ? leftWeight * node.leftDistance : -1;
//int rightTorque = (node.rightChild != null) ? rightWeight * node.rightDistance : -1;
System.out.printf("Node %d: Left distance = %d, Right distance = %d\n", node.id, node.leftDistance, node.rightDistance);
System.out.printf("Node %d: Left weight = %d, Right weight = %d\n", node.id, leftWeight, rightWeight);
System.out.printf("Node %d: Left torque = %d, Right torque = %d\n", node.id, leftTorque, rightTorque);
//if (leftTorque != rightTorque)
if (Math.abs(leftTorque - rightTorque) > 1) {
return -1;
}
int nodeWeight = Math.max(leftWeight, rightWeight) + node.weight;
System.out.printf("Node %d weight = %d\n", node.id, nodeWeight);
return nodeWeight;
}
public static void main(String[] args) {
Node root = createTree();
Map<Node, Node> parentMap = new HashMap<>();
int result = isBalanced(root);
if (result == -1) {
System.out.println("Unbalanced");
} else {
System.out.println("Balanced");
}
}
}
It's not working correctly, for example the input given below is supposed to output unbalanced meaning isBalanced needs to return -1. My code keeps saying that it is balanced with a weight of 3. I wanted ask why my code is incorrect and how to fix it to work correctly. The constraints is that I have to use a TreeMap or hashMap to consctruct the tree and use a recursive method that takes one pass through the tree to determine if it is balanced.
9 3 -1 -1 -1 -1
12 14 23 15 94 5
23 1 -1 -1 -1 -1
35 1 56 10 9 20
41 5 12 10 35 20
56 2 77 1 88 1
77 2 -1 -1 -1 -1
88 2 -1 -1 -1 -1
94 3 -1 -1 -1 -1

Step by step
Solved in 2 steps

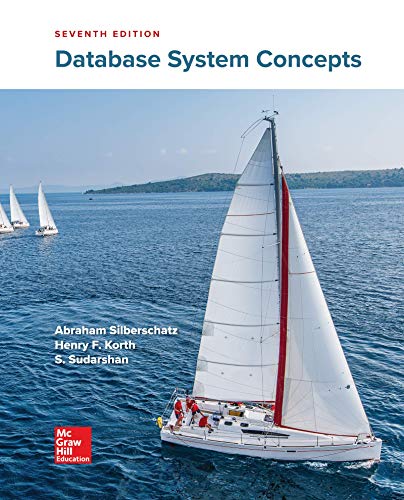
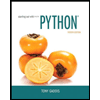
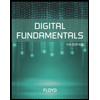
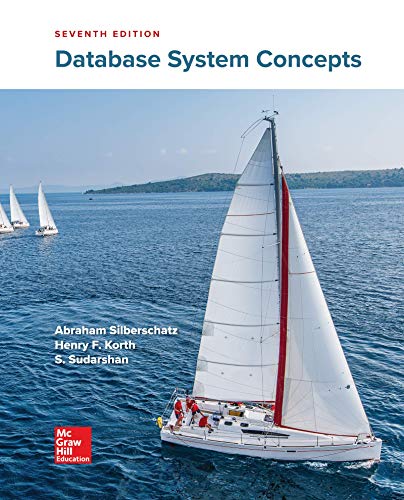
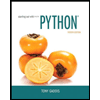
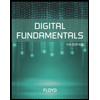
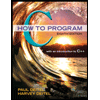
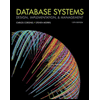
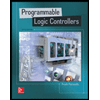