I am having trouble with these 2 review questions for my C++ course. Ex 9: Storing an array into a file. a) Implement the integer array {1,2,3,4,5}. b) Implement a save function which stores the array into a file using comma delimited format (1,2,3,4,5). Example File 1,2,3,4,5,
I am having trouble with these 2 review questions for my C++ course.
Ex 9: Storing an array into a file.
a) Implement the integer array {1,2,3,4,5}.
b) Implement a save function which stores the array into a file using comma delimited format (1,2,3,4,5).
Example File
1,2,3,4,5,
Ex 10: Reading an array into a file.
a) Implement a partially filled integer array of size 100.
b) Implement a load function which:
1) Reads the input file (from problem 9) one line at a time.
2) Parses each line using stringstream into tokens (comma delimiter).
3) Converts each token into an integer then stores each token into the array.
c) Implement an output function that outputs the array to the console.
Example Output (from file to the array, to console)
1 2 3 4 5

Step by step
Solved in 2 steps

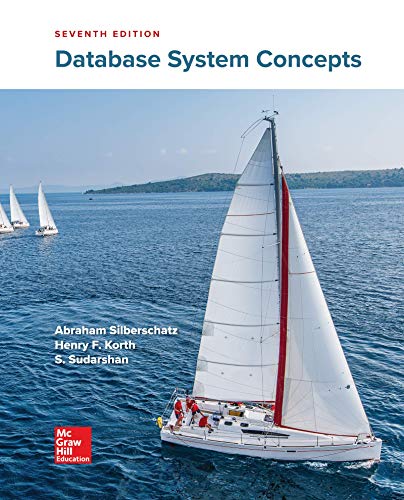
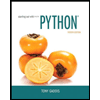
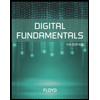
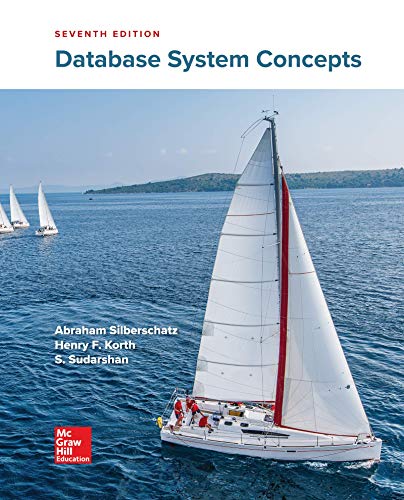
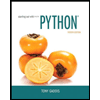
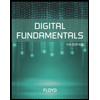
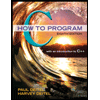
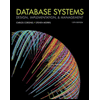
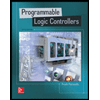