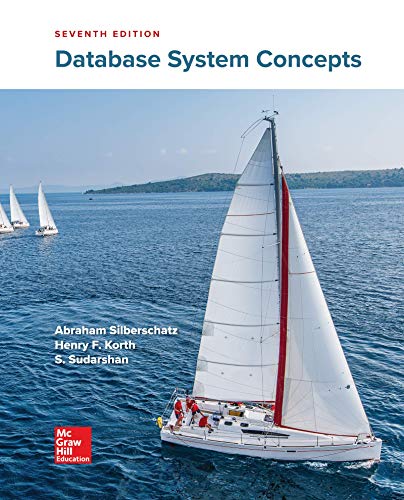
How would I create this code in Java? The coding prompt is attached below.
SAMPLE RUN BELOW (user entry in bold)
Make sure that for the search feature it can search between author, title, or isbn depending on what the user enters.
Welcome to the book program!
Would you like to create a book object? (yes/no): yEs (yEs, Yes,..., No, NO,nO...etc all acceptable entries)
Enter the author, title and the isbn of the book separated by /: Ericka Jones/Java made Easy/458792132
Got it!
Now, tell me if it is a bookstore book or a library book (enter BB for bookstore book or LB for library book): BSB (Lb, bB...etc all acceptable entries)
Oops! That’s not a valid entry. Please try again: Bookstore
Oops! That’s not a valid entry. Please try again: bB
Got it!
Enter the list price of JAVA MADE EASY by ERICKA JONES: 14.99
Is it on sale? (yes/no): yes (yEs, Yes,..., No, NO,nO...etc all acceptable entries)
Deduction percentage: 15% (Enter this as a percentage)
Got it!
Here is your bookstore book information
[458792132-JAVA MADE EASY by ERICKA JONES- $14.99 listed for $12.74] (this output is what the toString() returns)
If Java Made Easy wasn’t on sale, your code should print: [458792132-JAVA MADE EASY by ERICKA JONES, $14.99]
Would you like to create a book object? (yes/no): yeah
Oops! That’s not a valid entry. Please try again: yes
Enter the author, title and the isbn of the book separated by /: Eryc Jones/Java as a healing language/95879213m
Got it!
Now, tell me if it is a bookstore book or a library book (enter BB for bookstore book or LB for library book): LB
What’s the subject: Medicine (MeDiciNE, MEDICINE.... are acceptable entries . See list of subjects at the end of this document.)
Got it!
Here is your library book information
[958792130-JAVA AS A HEALING LANGUAGE by ERYC JONES-R.09.ERI.M]
Would you like to create a book object? (yes/no): yes
Enter the author, title and the isbn of the book separated by /: Erica Jone/The Art of Java /958792139
Got it!
Now, tell me if it is a bookstore book or a library book (enter BB for bookstore book or LB for library book): LB
What’s the subject: Fine art
Oops! That’s not a valid entry. Please try again: Fine arts
Got it!
Here is your library book information
[958792139-THE ART OF JAVA by ERICA JONE-N.01.ERI.9]
Would you like to create a book object? (yes/no): no
Sure!
Here are all the books you entered...
Library Books (2)
[958792130-JAVA AS A HEALING LANGUAGE by ERYC JONES-R.09.ERI.M]
[958792139-THE ART OF JAVA by ERICA JONE-N.01.ERI.9]
_ _ _ _
Bookstore Books (1)
[458792132-JAVA MADE EASY by ERYCKA JONES, $14.99 listed for $12.74]
_ _ _ _
Would like to search for a book? (yes/no): yes
Search by isbn, author or title? Author (IsbN, aUthor, tiTle ...etc are acceptable entries. Here assume that the user keys in correct entries)
Enter the first three letters of the author: Eri
We found 1 Library Book (s) and 1 Book Store Book(s):
[458792132-JAVA MADE EASY by ERYCKA JONES, $14.99 listed for $12.74]
[958792139-THE ART OF JAVA by ERICA JONE-N.01.ERI.9]
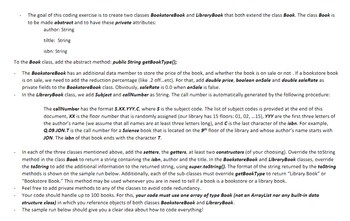

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 9 images

- (PLEASE USE JAVA AND JFRAME GUI) Game rules:The game consists of a two-dimensional field of size m × n (game field). Each element ofthe game field is a button with a number assigned to it.Initialization of the game:• The numbers on the buttons of the game field are set to a random digit between 0and 9• The target value is displayed above the game field• The current sum of the numbers displayed on the buttons of the game field is printedbelow the game field• The number of moves to completion is displayed in the upper right corner above thegame field.Playing:The first move is determined by the player by selecting any button (button A) on thegame field.1. The player is allowed to choose a second button (button B) located in the columnor row of the previously selected button (A).2. Upon selecting the second button (B), the value of button (A) is updated accordingto the following formula: A = (AoperationB)mod10.3. The operation for the basic game will be +.4. Decrement the number of moves…arrow_forward1.In your game console class, add a start function which should prompt the user for an email address. -Use a regular expression to validate that it is a valid email address and prompt them again until they enter a valid one. -Next, prompt the user to enter a credit card number and use a regular expression to validate the credit card. -Prompt them again until they enter a valid one and once done, display the game menu. Existing Code below: class Game {constructor(name){this.name = name;}} class GameConsole{constructor(){this.games = [];} load(){var gameNames = ["Zelda", "Halo", "Mario", "The God Among Us\n"];for (var i = 0; i < gameNames.length; i++) {let game = new Game(gameNames[i]);this.games.push(game);}} log(){console.log("Games Loaded:");for (var i = 0; i < this.games.length; i++) {var name = this.games[i].name;console.log(name);}}} let gamecon = new GameConsole();gamecon.load();gamecon.log(); const prompt = require('prompt-sync')(); var adventurersName = ["Captain…arrow_forwardJava code File IO ApplicationA shop collects the following information from its customers:1. Full name (first name, last name, and optional middle initial) 2. Address (street name and number, city, zip code, and state)3. Phone number 4. Email 5. First visit/service date6. Most recent visit/service date7. Total amount charged8. Current balance and description of the last service You are to write a program that allow the shop to manage their customers. The shop needs the following functionalities:1. Search for a customer by:a. Nameb. Phone numberc. Email (The result of the search would have similar option as in the “List all customers” option below)2. List all customersa. Select and edit a customer b. Delete a customer3. Add a new customera. Prompt for appropriate data fields4. Get account consolidation sheet that shows total charges and total balance of all customers5. Get the records of the biggest 5 spenders (spend the most)6. Exit and save all changes (all changes to the customers…arrow_forward
- Create a simple Java application to manage student information. The application should have a text-based interface. The application should prompt the user for the following information: Full Name, Degree, and Points. The user should be able to continuously enter student data until an exit key is pressed (se the algorithm below). After the student has entered the student data the application should display all student data entered. Algorithm 1 Ask if the user if wants to stop entering data 2 Do the following until the student wants to stop entering data 2.1 Prompt the user to enter data for a new student 2.2 Save the new student data 2.3 Ask if the user wants to stop entering data 2 Show all student data entered 3 Exit program Example input: Text in bold is entered by the user Do you want to continue (y/n) ? y Enter the student's name: John Smith Enter the student's degree: Computer Science Enter the student's points: 125 Do you want to continue (y/n) ? y Enter the student's name:…arrow_forwardC++ program Write a method that displays information about a movie. The method accepts the movie title, running time in minutes and the release year as arguments. Provide a default value for the running time so that if you call the method without minutes, it defaults to 90. Write a main() method that demonstrates you can call the method with two or three arguments. Save the file as Movie.cpparrow_forwardTrue or False: Object privileges allow you to add and drop usersarrow_forward
- true of false: a) The first step in any programming task is to start coding. b) The only places we would ever want to put a comment in our code are above a method and above a class.arrow_forwardWhat form of object should be created if you want to read data from a text file?arrow_forwardFor beginning Java, need help with this: " Purpose: Define class using OO approach Understand how to create and manipulate list of objects Design and create a well-structure program using basic programming constructs and classes Description: Write a program to help you to manage courses you are taking this semester. Your program provides the following options: List all courses Add a course Search word: show all the courses that has the word in the course title (ask the user for the word) List >= input-unit: show all the courses that has unit >= input-unit (ask the user for input) Exit Explanation of options: The program prints your course list. Initially, it should say “Your list is empty” The program asks the user input for a course including: course ID, number of units and title. The program asks the user to input the search word. Then, display all courses that has that word in the title. The search must be case-insensitive. For example, “computer” and…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
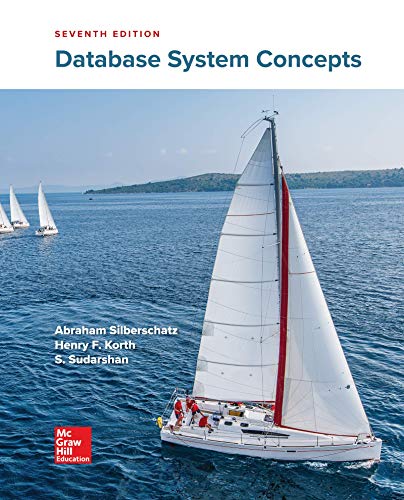
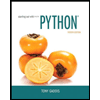
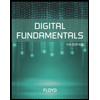
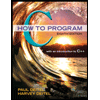
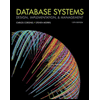
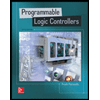