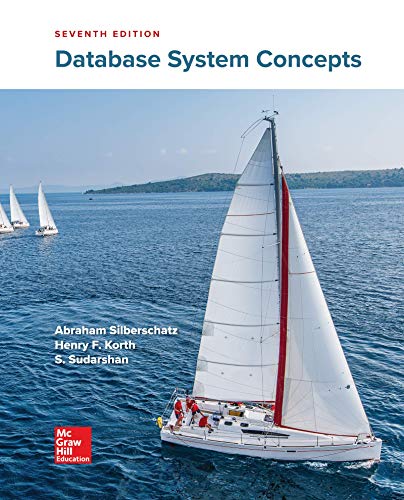
program5_1.py
Write a program that displays a table of ten distance equivalents in miles and kilometers. See Example output. You must generate the table by running a function inside a loop in main. Generate a random integer from 10 to 60, inclusive, in each loop cycle. Use this latter value as the miles argument to the function. Repeat: The function prints the table. Print the miles in a column 5 characters wide with 2 decimals and the kilometers in a column 13 characters wide with 5 decimals. Use the column formatting concepts at the end of Chapter 2, not tabs or other methods not in this course.
Example output

CODE IN PYTHON:
import random
def toKilometers(miles):
return miles * 1.60934
print("MILES KILOMETERS")
for i in range(10):
miles = random.randrange(10, 61) * 1.0
km = toKilometers(miles)
print("{0:.2f} {1:.5f}".format(miles, km))
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Create a function that takes a number as an argument and returns "Fizz", "Buzz" or "FizzBuzz" If the number is a multiple of 3 the output should be "Fizz" • If the number given is a multiple of 5, the output should be "Buzz" If the number given is a multiple of both 3 and 5, the output should be "FizzBuzz" ● • If the number is not a multiple of either 3 or 5, the number should be output on its own as shown in the examples below. • The output should always be a string even if it is not a multiple of 3 or 5. Examples fizz buzz (3) → "Fizz" fizz buzz (5) → "Buzz" fizz buzz (15) ➡ "FizzBuzz" fizz buzz (4) "4" (Ctrl)arrow_forwardCustomized step counter Learning Objectives In this lab, you will Create a function to match the specifications Use floating-point value division Instructions A pedometer treats walking 2,000 steps as walking 1 mile. It assumes that one step is a bit over 18 inches (1 mile = 36630 inches, so the pedometers assume that one step should be 18.315 inches). Let's customize this calculation to account for the size of our stride. Write a program whose input is the number of steps and the length of the step in inches, and whose output is the miles walked. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print(f'{your_value:.2f}') Ex: If the input is: 5345 18.315 the output is: You walked 5345 steps which are about 2.67 miles. Your program must define and call the following function. The function should return the number of miles walked.def steps_to_miles(user_steps, step_length) # Define your function here if __name__…arrow_forwardQ1: complete the function that will return the multipication of 3 given numbers - a,b,c. In [ ]: def multiply_numbers(a,b,c): II II || """insert the code that performs the operation. Use the variable 'number' to hold and return the value II || || # your code here return numberarrow_forward
- PYTHON PTGRAMMING ONLY NEED HELP MAKING A FLOWCHART TO MATCH MY CODE CODE IS CORRECT JUST NEED HELP AKING TO FLOWCHART QUESTION, CODE, FLOWCHART EXAMPLE PROVIDED QUESTION: Write a function named max that accepts two integer values as arguments and returns thevalue that is the greater of the two. For example, if 7 and 12 are passed as arguments tothe function, the function should return 12. Use the function in a program that prompts theuser to enter two integer values. The program should display the value that is the greaterof the two. MY CODE: # Function to find the maximum of two integersdef maximum(num1, num2):return max(num1, num2)# Function to get integer input from the user with input validationdef get_integer_input(prompt):while True:try:value = int(input(prompt)) # Prompt the user for inputreturn valueexcept ValueError:print("Please enter a valid integer.") # Handle input validation# Main program logicdef main():print("Enter two integer values to find the greater of the…arrow_forwardCreating a function table, python code from the following pictures:arrow_forwardprogram5_1.py Part 1Write a program that displays a table of ten distance equivalents in miles and kilometers. See Example output. You must generate the table by running a function inside a loop in main. Generate a random integer from 10 to 60, inclusive, in each loop cycle. Use this latter value as the miles argument to the function. Repeat: The function prints the table. Print the miles in a column 5 characters wide with 2 decimals and the kilometers in a column 13 characters wide with 5 decimals. Use the column formatting concepts at the end of Chapter 2, not tabs or other methods not in this course. Please include your psudocode to explain your code. Example output MILES KILOMETERS 52.00 83.68568 11.00 17.70274 40.00 64.37360 21.00 33.79614 14.00 22.53076 23.00 37.01482 48.00 77.24832 22.00 35.40548 15.00 24.14010 16.00 25.74944 program5_2.py Part 2Write another program that generates another table with the same columns and decimals…arrow_forward
- Complete the Funnyville High School registration program where user is prompted for her full name and the program generates email id and temporary password. Sample run: generate_EmailID: This function takes two arguments: the user’s first name and last name and creates and returns the email id as a string by using these rules: The email id is all lower case. email id is of the form “last.first@fhs.edu”. e.g. For "John Doe" it will be "doe.john@fhs.edu". See sample runs above. generate_Password: This function takes two arguments: the user’s first name and last name and generates and returns a temporary password as a string by using these rules. Assume that user's first and last names have at least 2 letters. The temporary password starts with the first 2 letters of the first name, made lower case. followed by a number which is the sum of the lengths of the first and last name (For example this number will be 7 for "John Doe" since length of "John" is 4 and length of "Doe" is…arrow_forwardWhat are the steps for making a list?arrow_forwardWrite a flowchart and C code for a program that does the following: Declare an array that will store 9 values Use a for() statement to store user entered scores for all 9- holes Create a function and pass the array into the definition of the function to compute the total Use an accumulating total statement to compute the total score Display the overall total to the output screen so that the golfer can see if he won.arrow_forward
- Use C++arrow_forwardComplete the code that returns the value of f(x), which has the value 1 inside the range −1 ≤ x ≤ 1 otherwise it is the value 0. f(x) [ ] # complete the function to return the value of 1, x = [-1, 1] 0, otherwise #f(x) given x. # return as the value called variable def function_f(x): # YOUR CODE HERE return fval "fval" ## Check your code below using print command ← → +0 0+arrow_forwardJS You are writing a number guessing program where the player must try to guess the secret number 17. This function will take a player's guess and tell them if they are either right or whether they should guess higher or lower on their next turn Write a function named "higher_lower" that takes an int as a parameter and returns "higher" if 17 is greater than the input and "lower" if 17 is less than the input, and "correct" if 17 is equal to the input.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
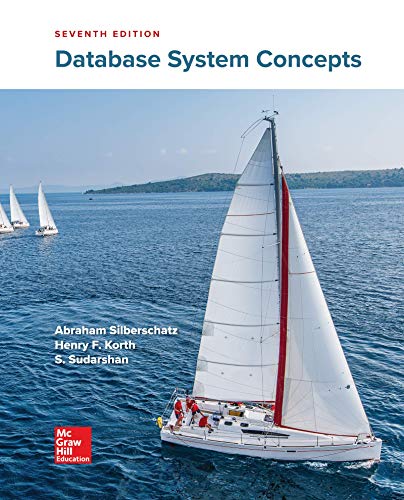
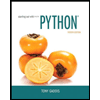
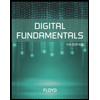
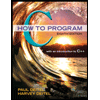
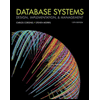
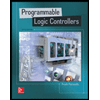