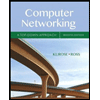
How do I complete the code for the void initMemo() {} section and the unsigned long long mfib(int n)} section where it says ADD CODE?
Fibonacci Sequence Coding using Memoization C PROGRAM HELP Details:
- Create the mfib() function to calculate Fibonacci using memoization
- Also create the initMemo() function to clear out memoization table(s) at the beginning of each mfib() run
The output of the program will record the relative amount of time required from the start of each function calculation to the end.
Note the speed difference between fib and mfib!
Turn in your commented program and a screen shot of the execution run of the program.
Do not be alarmed as the non-memoization Fibonacci calls take a long time to calculate, especially the higher values.
Be patient. It should finish.
Given Code:
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
#include <time.h>
// max number of inputs
#define MAX_FIB 200
// result of call to fib function
unsigned long long result;
//loop variable
int i;
// ========================== MEMO DEFINITIONS ==========================
unsigned long long memo[MAX_FIB]; // array to hold computed data values
bool valid[MAX_FIB]; // array to hold validity of data
// make all entries in the memoization table invalid
void initMemo() {
// ***** ADD YOUR INITIALIZATION CODE HERE *****
// ***** ADD YOUR INITIALIZATION CODE HERE *****
// ***** ADD YOUR INITIALIZATION CODE HERE *****
// ***** ADD YOUR INITIALIZATION CODE HERE *****
// ***** ADD YOUR INITIALIZATION CODE HERE *****
return;
}
// ===================== TIME DEFINITIONS ===============
// timer functions found in time.h
// time_t is time type
time_t startTime;
time_t stopTime;
// get current time in seconds from some magic date with
// t = time(NULL);
// or
// time(&t);
// where t is of type time_t
//
// get difference in secs between times (t1-t2) with
// d = difftime(t1, t2);
// where d is of type double
// ========================== NAIVE FIB =========
unsigned long long fib(int n) {
if (n < 1) {
return 0;
} else if (n == 1) {
return 1;
} else {
return fib(n-2) + fib(n-1);
}
}
// ========================== MEMOIZED FIB ==========================
unsigned long long mfib(int n) {
// ***** ADD YOUR MFIB CODE HERE *****
// ***** ADD YOUR MFIB CODE HERE *****
// ***** ADD YOUR MFIB CODE HERE *****
// ***** ADD YOUR MFIB CODE HERE *****
// ***** ADD YOUR MFIB CODE HERE *****
return 0; // replace this return with real computation
}
// ========================== MAIN PROGRAM ==========================
int main() {
for (i = 0; i < 55; i+=5) {
// get start time
time(&startTime);
// call fib
result = fib(i);
// get stop time
time(&stopTime);
printf("fib of %d = %llu\n", i, result);
printf("time taken (sec) = %lf\n\n", difftime(stopTime, startTime));
}
printf("\n\n\n");
for (i = 0; i < 90; i+=5) {
// get start time
time(&startTime);
printf("fib of %d = %llu\n", i, result);
printf("time taken (sec) = %lf\n\n", difftime(stopTime, startTime));
}
printf("\n\n\n");
for (i = 0; i < 90; i+=5) {
// get start time
time(&startTime);
// call mfib
initMemo();
result = mfib(i);
// get stop time
time(&stopTime);
printf("mfib of %d = %llu\n", i, result);
printf("time taken (sec) = %lf\n\n", difftime(stopTime, startTime));
}
return 0;
}
Sample output:
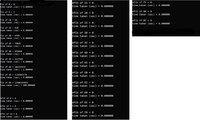

Step by stepSolved in 3 steps

- 1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forwardQuestion 5 Write the code for the function printRoster, it should print out the contacts in a format similar to the example. You can use a range-based for-loop and auto to simplify the solution, if you wish. #include #include #include #include #include using namespace std; void printRoster(map>>& roster) { // Fill code here int main() { map>> roster; roster["ELCO"]["CS-30"]. emplace_back("Anthony Davis"); roster["ElCo"]"cS-30"j. emplace_back("Talen Horton-Tucker"); roster["ElCo"Ï"BUS-101"].emplace_back("LeBron James"); roster["SMC"]["CHEM-101"].emplace_back("Russell Westbrook"); printRoster(roster); return 0; Here is the sample output: ElCo BUS-101: LeBron James CS-30: Anthony Davis Talen Horton-Tucker SMC CHEM-101: Russell Westbrookarrow_forwardWrite a function strlen that takes one char str[] as an argument, and return the number of characters in the str[] array. (c++)arrow_forward
- Note: It`s C++arrow_forwardA1: File Handling with struct C++ This program is to read a given file and display the information about employees according to the type of employee. Specifically, the requirements are as follows. Read the given file of the information of employees. Store the information in an array or arrays. Display all the salaried employees first and then the hourly employees Prompt the user to enter an SSN and find the corresponding employee and display the information of that employee. NOTE : S- salaryemployee , H- Hourly employee S 135-25-1234 Smith Sophia DevOps Developer 70000H 135-67-5462 Johnaon Jacob SecOps Pentester 30 60.50S 252-34-6728 William Emma DevOps DBExpert 100000S 237-12-1289 Miller Mason DevOps CloudArchitect 80000S 581-23-4536 Jones Jayden SecOps Pentester 250000S 501-56-9724 Rogers Mia DevOps Auditor 90000H 408-67-8234 Cook Chloe DevOps QAEngineer 40 45.10S 516-34-6524 Morris Daniel DevOps ProductOwner 300000H 526-47-2435 Smith Natalie DevOps…arrow_forwardC++ PLEASE HELP MUST MATCH OUTPUT IN PICS In this lab, you're going to be working with partially filled arrays that are parallel with each other. That means that the row index in multiple arrays identifies different pieces of data for the same person. This is a simple payroll system that just calculates gross pay given a set of employees, hours worked for the week and hourly rate. Parallel Arrays First, you have to define several arrays in your main program employee names for each employee hourly pay rate for each employee total hours worked for each employee gross pay for each employee You can use a global SIZE of 50 to initialize these arrays Second, you will need a two dimension (2-D) array to record the hours worked each day for an employee. The number of rows for the 2-D array should be the same as the arrays above since each row corresponds to an employee. The number of columns represents days of the week (7 last I looked) Functions Needed In this lab, you must read in the…arrow_forward
- Complete the C++ function given below:arrow_forwardhelp me solve this in c++ please Write a program that asks the user to enter a list of numbers from 1 to 9 in random order, creates and displays the corresponding 3 by 3 square, and determines whether the resulting square is a Lo Shu Magic Square. Notes Create the square by filling the numbers entered from left to right, top to bottom. Input validation - Do not accept numbers outside the range. Do not accept repeats. Must use two-dimensional arrays in the implementation. Functional decomposition — Program should rely on functions that are consistent with the algorithm.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
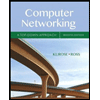
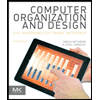
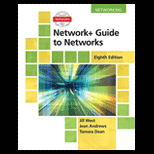
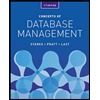
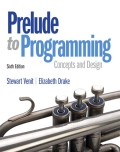
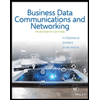