Help me create the following function constraints in w04c_ans.py the test case file provided in one of the image is named w04c_test_public.py copy and paste it in notepad ++ for it to look exactly the same. w04c_test_public.py import io import textwrap import unittest from unittest.mock import patch # TODO: Replace w04c_ans with the name of your Python file. # Make sure that this file and that file are in the same # folder. from w04c_ans import print_frame, is_valid_num class TestIsValidNum(unittest.TestCase): def test_pt01(self): a_test = is_valid_num('2') self.assertTrue(a_test) def test_pt02(self): a_test = is_valid_num('-5') self.assertFalse(a_test) def test_pt03(self): a_test = is_valid_num('512abc32') self.assertFalse(a_test) class TestPrintFrame(unittest.TestCase): @patch('sys.stdout', new_callable=io.StringIO) def test_pt01(self, mocked_print): print_frame() self.assertEqual( mocked_print.getvalue(), textwrap.dedent( """\ ┌───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┐ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ └───┤ └───┤ └───┤ └───┤ └───┤ └───┤ └───┤ └───┤ └───┤ └───┴───┤ │ │ │ │ │ │ │ │ │ │ │ └───────┴───────┴───────┴───────┴───────┴───────┴───────┴───────┴───────┴───────────┘ """ ) ) if __name__ == '__main__': unittest.main() w04c_ans.py def print_frame(): pass def is_valid_num(): return False def main():
Help me create the following function constraints in w04c_ans.py
the test case file provided in one of the image is named w04c_test_public.py copy and paste it in notepad ++ for it to look exactly the same.
w04c_test_public.py
import io
import textwrap
import unittest
from unittest.mock import patch
# TODO: Replace w04c_ans with the name of your Python file.
# Make sure that this file and that file are in the same
# folder.
from w04c_ans import print_frame, is_valid_num
class TestIsValidNum(unittest.TestCase):
def test_pt01(self):
a_test = is_valid_num('2')
self.assertTrue(a_test)
def test_pt02(self):
a_test = is_valid_num('-5')
self.assertFalse(a_test)
def test_pt03(self):
a_test = is_valid_num('512abc32')
self.assertFalse(a_test)
class TestPrintFrame(unittest.TestCase):
@patch('sys.stdout', new_callable=io.StringIO)
def test_pt01(self, mocked_print):
print_frame()
self.assertEqual(
mocked_print.getvalue(),
textwrap.dedent(
"""\
┌───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┐
│ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │ │
│ └───┤ └───┤ └───┤ └───┤ └───┤ └───┤ └───┤ └───┤ └───┤ └───┴───┤
│ │ │ │ │ │ │ │ │ │ │
└───────┴───────┴───────┴───────┴───────┴───────┴───────┴───────┴───────┴───────────┘
"""
)
)
if __name__ == '__main__':
unittest.main()
w04c_ans.py
def print_frame():
pass
def is_valid_num():
return False
def main():
while True:
num_frames = input('Enter number of frames from 2 to 10 inclusive: ')
if not is_valid_num(num_frames):
print('Entered input is either not a number or is not between 2 and 10 inclusive. Please try again.')
continue
num_frames = int(num_frames)
print_frame(num_frames)
break
if __name__ == '__main__':
main()



Step by step
Solved in 3 steps with 1 images

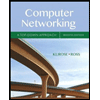
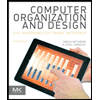
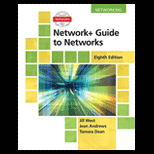
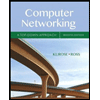
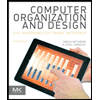
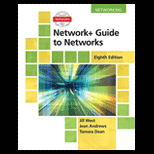
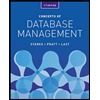
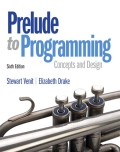
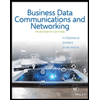