Question: Hello, for the last line in the code, could anyone show me how to perform it using f' strings, instead of the format() method and join()method. I can use f strings to format the first half of the code, print(f' {course:<8} {avg_grade:>4.1f}') In the code, gradebook is the dictionary, course is the key, and grades is the value(grades is in the format of a list) for course, grades in gradebook.items(): grades.sort() # sort the list of grades sum_grades = sum(grades) # sum all grades for the course count_grades = len(grades) # determine the number of grades in the list of grades avg_grade = sum_grades / count_grades # calculate the average for the course if avg_grade < lowest_avg[1]: # determine the course with the lowest average lowest_avg = (course, avg_grade) print("{:<8} {:>4.1f}% {:>25}".format(course, avg_grade, " ".join("{:>4}%".format(g) for g in grades)))
Question: Hello, for the last line in the code, could anyone show me how to perform it using f' strings, instead of the format() method and join()method. I can use f strings to format the first half of the code,
print(f' {course:<8} {avg_grade:>4.1f}')
In the code, gradebook is the dictionary, course is the key, and grades is the value(grades is in the format of a list)
for course, grades in gradebook.items():
grades.sort() # sort the list of grades
sum_grades = sum(grades) # sum all grades for the course
count_grades = len(grades) # determine the number of grades in the list of grades
avg_grade = sum_grades / count_grades # calculate the average for the course
if avg_grade < lowest_avg[1]: # determine the course with the lowest average
lowest_avg = (course, avg_grade)
print("{:<8} {:>4.1f}% {:>25}".format(course, avg_grade, " ".join("{:>4}%".format(g) for g in grades)))


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Thank you, I understand how the code is executed. The problem is that I'm only allowed to use either a for loop, or list comprehension to achieve the result. Is there any way to do so without implementing the "join" function or method, as I have not learned that in class yet.
Here is the exact requirements:
- use another for loop to print all grades in the grades list for the course. Use a format identifier to allow for printing of a right-justified grade with a width of 4 followed by a percent sign
Is there any way to only use f strings, for loops, and/or list comprehension to achieve the same result.
Thank you
Hello thank you for the solution. Is there also a way to format the list without using the .join method, and just using f' strings, perhaps in a list comprehension?
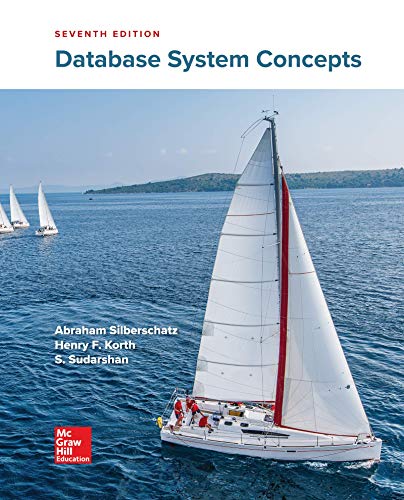
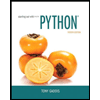
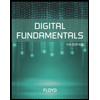
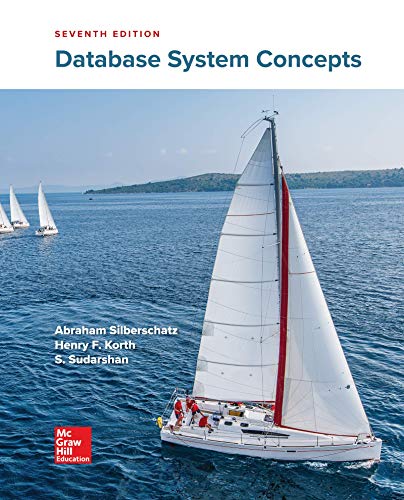
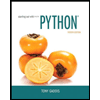
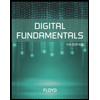
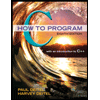
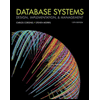
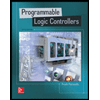