Hello! I am having trouble on how to write out the Java code based on the instructions (//***) that I am given. As a note, this specific Java code is a code that is going to setup the basic framework for a game of Yahtzee. In other words, you will need to understand the rules of Yahtzee and implement the rules into coding. I appreciate the help. Thanks! Also, I am using the IntelliJ IDEA application when writing out this program. I also included some code above the instructions so that you know what to help with here. Here is what I need help with (in bold): do { System.out.println(); System.out.println(REROLL_MESSAGE_1); System.out.println(); System.out.println(REROLL_MESSAGE_2); System.out.println(REROLL_MESSAGE_3); System.out.println(REROLL_MESSAGE_4); System.out.println(); System.out.printf(REROLL_MESSAGE_5, (MAX_NUMBER_ROLLS - numberOfRolls)); System.out.println(); System.out.println(); System.out.print(REROLL_MESSAGE_6); dice2Reroll = input.nextLine().trim(); switch (dice2Reroll.toUpperCase()) { case 'X': turnOver = true; gameExit = true; break; case 'S': displayScoreSheet(); break; case 'D': displayDice(); break; case '0': turnOver = true; break; case "": displayErrorMessage(); break; default: dice2Reroll = dice2Reroll.replace(target:" ", replacement:""); String checkDice2Reroll = dice2Reroll; checkDice2Reroll = checkDice2Reroll.replaceFirst(regex:"1", replacement:" "); checkDice2Reroll = checkDice2Reroll.replaceFirst(regex:"2", replacement:" "); checkDice2Reroll = checkDice2Reroll.replaceFirst(regex:"3", replacement:" "); checkDice2Reroll = checkDice2Reroll.replaceFirst(regex:"4", replacement:" "); checkDice2Reroll = checkDice2Reroll.replaceFirst(regex:"5", replacement:" "); if (checkDice2Reroll.isBlank()) { for (int i = 0; i < dice2Reroll.length(); i++) { die2Reroll = dice2Reroll.charAt(i); switch (die2Reroll) { case '1': die1 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1; break; case '2': die2 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1; break; case '3': die3 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1; break; case '4': die4 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1; break; case '5': die5 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1; break; } } numberOfRolls++; displayDice(); if (numberOfRolls == MAX_NUMBER_ROLLS) turnOver = true; } else { displayErrorMessage(); } } } while (!turnOver); //*** //*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE //*** //*** 1) Write the if-statement-expression for the if-statement; where it says if () { is where it begins. //*** A) The expression must use the correct Java syntax for "not gameExit". //*** B) "not" must be translated to the correct Java Boolean Operator. //*** C) "gameExit" is a boolean variable. //*** 2) Code block for if-statement in Step 1: //*** A) Call method "displayScoreSheet" passing no arguments. //*** B) Define three variables of boolean datatype named: //*** a) "isValidEntry" //*** b) "categoryPicked" //*** c) "continuePrompting" //*** NOTE: These variables do not need to be initialized here, just declared. //*** C) Code block for do-while loop starting on do {: //*** a) Write a statement that assigns the value of true to the variable "isValidEntry". //*** b) Write a statement that assigns the value of false to the variable "categoryPicked". //*** //*** Insert the if-statement-expression from Step 1 into this if-statement. //*** if () { //*** //*** Insert code for Step 2A here. //*** //*** //*** Insert code for Step 2B here. //*** do { //*** //*** Insert code for Step 2C here. //***
Hello! I am having trouble on how to write out the Java code based on the instructions (//***) that I am given. As a note, this specific Java code is a code that is going to setup the basic framework for a game of Yahtzee. In other words, you will need to understand the rules of Yahtzee and implement the rules into coding. I appreciate the help. Thanks! Also, I am using the IntelliJ IDEA application when writing out this program. I also included some code above the instructions so that you know what to help with here. Here is what I need help with (in bold):
do {
System.out.println();
System.out.println(REROLL_MESSAGE_1);
System.out.println();
System.out.println(REROLL_MESSAGE_2);
System.out.println(REROLL_MESSAGE_3);
System.out.println(REROLL_MESSAGE_4);
System.out.println();
System.out.printf(REROLL_MESSAGE_5, (MAX_NUMBER_ROLLS - numberOfRolls));
System.out.println();
System.out.println();
System.out.print(REROLL_MESSAGE_6);
dice2Reroll = input.nextLine().trim();
switch (dice2Reroll.toUpperCase()) {
case 'X':
turnOver = true;
gameExit = true;
break;
case 'S':
displayScoreSheet();
break;
case 'D':
displayDice();
break;
case '0':
turnOver = true;
break;
case "":
displayErrorMessage();
break;
default:
dice2Reroll = dice2Reroll.replace(target:" ", replacement:"");
String checkDice2Reroll = dice2Reroll;
checkDice2Reroll = checkDice2Reroll.replaceFirst(regex:"1", replacement:" ");
checkDice2Reroll = checkDice2Reroll.replaceFirst(regex:"2", replacement:" ");
checkDice2Reroll = checkDice2Reroll.replaceFirst(regex:"3", replacement:" ");
checkDice2Reroll = checkDice2Reroll.replaceFirst(regex:"4", replacement:" ");
checkDice2Reroll = checkDice2Reroll.replaceFirst(regex:"5", replacement:" ");
if (checkDice2Reroll.isBlank()) {
for (int i = 0; i < dice2Reroll.length(); i++) {
die2Reroll = dice2Reroll.charAt(i);
switch (die2Reroll) {
case '1':
die1 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1;
break;
case '2':
die2 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1;
break;
case '3':
die3 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1;
break;
case '4':
die4 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1;
break;
case '5':
die5 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1;
break;
}
}
numberOfRolls++;
displayDice();
if (numberOfRolls == MAX_NUMBER_ROLLS)
turnOver = true;
}
else {
displayErrorMessage();
}
}
} while (!turnOver);
//***
//*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE
//***
//*** 1) Write the if-statement-expression for the if-statement; where it says if () { is where it begins.
//*** A) The expression must use the correct Java syntax for "not gameExit".
//*** B) "not" must be translated to the correct Java Boolean Operator.
//*** C) "gameExit" is a boolean variable.
//*** 2) Code block for if-statement in Step 1:
//*** A) Call method "displayScoreSheet" passing no arguments.
//*** B) Define three variables of boolean datatype named:
//*** a) "isValidEntry"
//*** b) "categoryPicked"
//*** c) "continuePrompting"
//*** NOTE: These variables do not need to be initialized here, just declared.
//*** C) Code block for do-while loop starting on do {:
//*** a) Write a statement that assigns the value of true to the variable "isValidEntry".
//*** b) Write a statement that assigns the value of false to the variable "categoryPicked".
//***
//*** Insert the if-statement-expression from Step 1 into this if-statement.
//***
if () {
//***
//*** Insert code for Step 2A here.
//***
//***
//*** Insert code for Step 2B here.
//***
do {
//***
//*** Insert code for Step 2C here.
//***

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

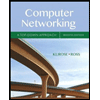
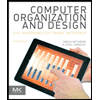
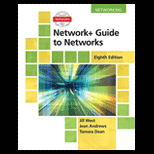
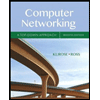
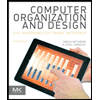
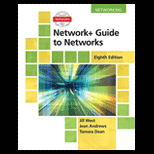
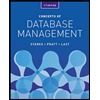
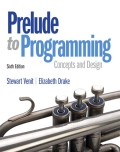
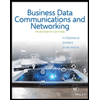