he following Python program solves Sudoku using backtracking. The method that starts the solution is "solve_sudoku(matrix)" and receives as input an n x n matrix where the empty inputs are represented by -1 from pprint import pprint def search_next_void(puzzle): for r in range(9): for c in range(9): if puzzle[r][c] == -1: return r, c return None, None def is_valid(puzzle, guess, row, col): row_vals = puzzle[row] if guess in row_vals: return False col_vars = [puzzle[i][col] for i in range(9)] if guess in col_vars: return False
The following Python program solves Sudoku using backtracking. The method that starts the solution is "solve_sudoku(matrix)" and receives as input an n x n matrix where the empty inputs are represented by -1
from pprint import pprint
def search_next_void(puzzle):
for r in range(9):
for c in range(9):
if puzzle[r][c] == -1:
return r, c
return None, None
def is_valid(puzzle, guess, row, col):
row_vals = puzzle[row]
if guess in row_vals:
return False
col_vars = [puzzle[i][col] for i in range(9)]
if guess in col_vars:
return False
row_start = (row // 3) * 3
col_start = (col // 3) * 3
for r in range(row_start, row_start + 3):
for c in range(col_start, col_start + 3):
if puzzle[r][c] == guess:
return False
return True
def solve_sudoku(puzzle):
row, col = search_next_void(puzzle)
if row is None:
return True
for guess in range(1, 10):
if is_valid(puzzle, guess, row, col):
print(board_example)
print("\n")
puzzle[row][col] = guess
if solve_sudoku(puzzle):
return True
puzzle[row][col] = -1
return False
what is the solution given by the program for the sudoku of the image?


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

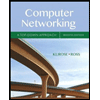
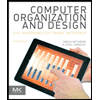
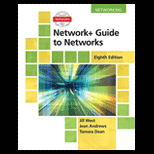
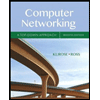
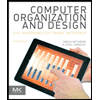
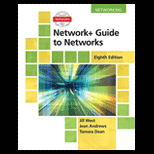
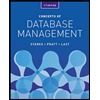
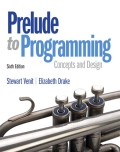
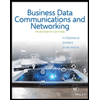