Given the following parallel arrays: int[] arrProdNumber = new int[] { 100, 115, 125, 142, 163, 199, 205, 388 }; string[] arrProdDesc = new string[] { "Kitten", "Cat", "Bird", "Chicken", "Dog", "Puppy", "Aardvark", "Zebra" }; Write a C# program that includes these arrays. Your program should then read a product number from the user and search the product number array for that number. If it is found, then your program should report the product number they entered and the corresponding product description. If the product number they enter is not in the first array, then your program should indicate that item number was not found. Here’s a sample run: //first run Enter product number: 115 Item #115's product description is Cat //second run Enter product number: 1963 Item #1963 does not exist in your inventory
. Given the following parallel arrays:
int[] arrProdNumber = new int[] { 100, 115, 125, 142, 163, 199, 205, 388 };
string[] arrProdDesc = new string[] { "Kitten", "Cat", "Bird", "Chicken", "Dog", "Puppy", "Aardvark", "Zebra" };
Write a C#
the product number array for that number. If it is found, then your program should report the product number they
entered and the corresponding product description. If the product number they enter is not in the first array, then your
program should indicate that item number was not found. Here’s a sample run:
//first run
Enter product number: 115
Item #115's product description is Cat
//second run
Enter product number: 1963
Item #1963 does not exist in your inventory
Some Random Number Generation Hints
Random rndNumber = new Random();
Console.WriteLine(rndNumber.Next()); //random integer
Console.WriteLine(rndNumber.Next(101)); //random integer between 0 and 100
Console.WriteLine(rndNumber.Next(10, 43)); //random integer between 10 and 42
Console.WriteLine(rndNumber.NextDouble()); //random double greater than 0, but less than 1
Console.WriteLine(rndNumber.NextDouble()*10); //random double between 0 and 10

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

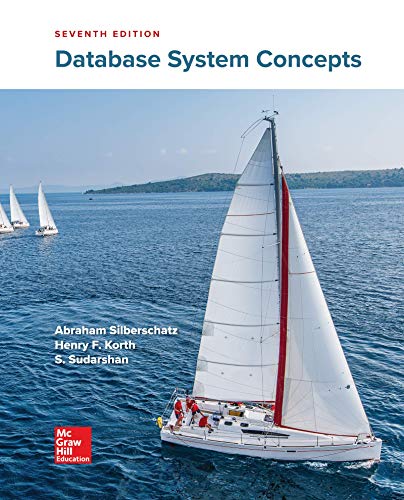
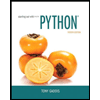
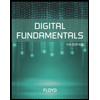
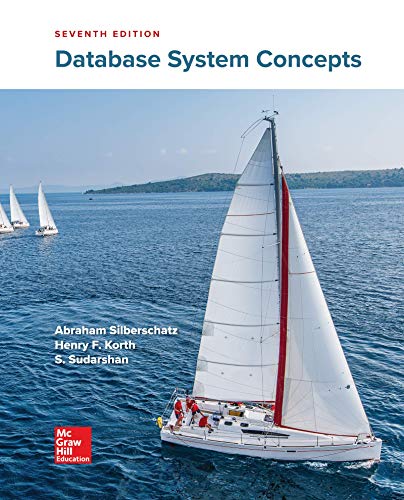
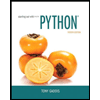
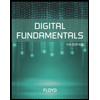
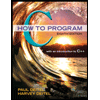
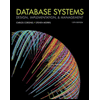
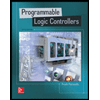