Given- struct tag { char ch; int i; }; struct bTag{ float f; double d; }; struct cTag{ struct aTag a; struct bTagb; int A[5]; }; typedef struct aTag aType; typedef struct bTag bType; typedef struct cTag cType; aType a, A[5]; bType b, B[5]; cType c, C[5]; aType *pa; bType *pb; cType *pc; 1a). Implement a function void InputFunc1(cType *ptr) that will input via scanf() the members of the structure pointed to by ptr. Assume that the memory space already exist. b). Implement a function cType InputFunc2(void) that will declare a local variable as cType temp. The function will then input the values of the members of temp using scanf(). Finally, the function will return temp. c) Implement a function void InputFunc3(cType C[], int n) that will input via scanf() the elements of the array of structure C. Parameter n represents the number of elements in the array. d) Implement a function void OutputFunc1(cType *ptr) that will output the members of the structure pointed to by ptr. Assume that the memory space already exist and that the values of the structure are valid. Use dereference operator and structure member operator only. DO NOT use the structure pointer operator ->. e). Implement a function void OutputFunc2(cType*ptr) similar to the previous problem. The difference here is that it is required to use the structure pointer operator ->. f). Implement a function void OutputFunc 3(c Type C[ ], int n) that will output the elements of array C using array indexing.
I need help in solving the question highlighted in bold for C language.
Given-
struct tag {
char ch;
int i;
};
struct bTag{
float f;
double d;
};
struct cTag{
struct aTag a;
struct bTagb;
int A[5];
};
typedef struct aTag aType;
typedef struct bTag bType;
typedef struct cTag cType;
aType a, A[5];
bType b, B[5];
cType c, C[5];
aType *pa;
bType *pb;
cType *pc;
1a). Implement a function void InputFunc1(cType *ptr) that will input via scanf() the members of the structure pointed to by ptr. Assume that the memory space already exist.
b). Implement a function cType InputFunc2(void) that will declare a local variable as cType temp. The function will then input the values of the members of temp using scanf(). Finally, the function will return temp.
c) Implement a function void InputFunc3(cType C[], int n) that will input via scanf() the elements of the array of structure C. Parameter n represents the number of elements in the array.
d) Implement a function void OutputFunc1(cType *ptr) that will output the members of the structure pointed to by ptr. Assume that the memory space already exist and that the values of the structure are valid. Use dereference operator and structure member operator only. DO NOT use the structure pointer operator ->.
e). Implement a function void OutputFunc2(cType*ptr) similar to the previous problem. The difference here is that it is required to use the structure pointer operator ->.
f). Implement a function void OutputFunc 3(c Type C[ ], int n) that will output the elements of array C using array indexing.

Step by step
Solved in 3 steps

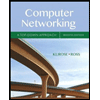
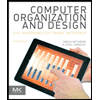
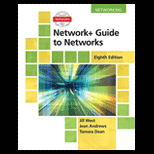
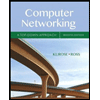
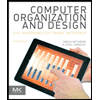
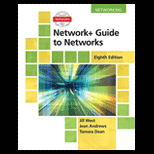
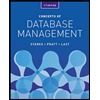
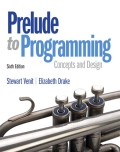
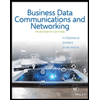