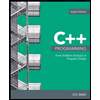
Concept explainers
Given a HashMap pre-filled with student names as keys and grades as values, complete main() by reading in the name of a student, outputting their original grade, and then reading in and outputting their new grade.
Ex: If the input is:
Quincy Wraight 73.1
the output is:
Quincy Wraight's original grade: 65.4 Quincy Wraight's new grade: 73.1
import java.util.Scanner;
import java.util.HashMap;
public class StudentGrades {
public static void main (String[] args) {
Scanner scnr = new Scanner(System.in);
String studentName;
double studentGrade;
HashMap<String, Double> studentGrades = new HashMap<String, Double>();
// Students's grades (pre-entered)
studentGrades.put("Harry Rawlins", 84.3);
studentGrades.put("Stephanie Kong", 91.0);
studentGrades.put("Shailen Tennyson", 78.6);
studentGrades.put("Quincy Wraight", 65.4);
studentGrades.put("Janine Antinori", 98.2);
// TODO: Read in new grade for a student, output initial
// grade, replace with new grade in HashMap,
// output new grade
}
}
java please

Step by stepSolved in 2 steps with 1 images

- The implementation of a queue in an array, as given in this chapter, uses the variable count to determine whether the queue is empty or full. You can also use the variable count to return the number of elements in the queue. On the other hand, class linkedQueueType does not use such a variable to keep track of the number of elements in the queue. Redefine the class linkedQueueType by adding the variable count to keep track of the number of elements in the queue. Modify the definitions of the functions addQueue and deleteQueue as necessary. Add the function queueCount to return the number of elements in the queue. Also, write a program to test various operations of the class you defined.arrow_forwarda. Write a FractionDemo program that instantiates several Fraction objects and demonstrates that their methods work correctly. Create a Fraction class with fields that hold a whole number, a numerator, and a denominator. In addition: Create properties for each field. The set access or for the denominator should not allow a 0 value; the value defaults to 1. Add three constructors. One takes three parameters for a whole number, numerator, and denominator. Another accepts two parameters for the numerator and denominator; when this constructor is used, the whole number value is 0. The last constructor is parameterless; it sets the whole number and numerator to 0 and the denominator to 1. (After construction, Fractions do not have to be reduced to proper form. For example, even though 3/9 could be reduced to 1/3, your constructors do not have to perform this task.) Add a Reduce() method that reduces a Fraction if it is in improper form. For example, 2/4 should be reduced to 1/2. Add an operator+() method that adds two Fractions. To add two fractions, first eliminate any whole number part of the value. For example, 2 1/4 becomes 9/4 and 1 3/5 becomes 8/5. Find a common denominator and convert the fractions to it. For example, when adding 9/4 and 8/5, you can convert them to 45/20 and 32/20. Then you can add the numerators, giving 77/20. Finally, call the Reduce() method to reduce the result, restoring any whole number value so the fractional part of the number is less than 1. For example, 77/20 becomes 3 17/20. Include a function that returns a string that contains a Fraction in the usual display format—the whole number, a space, the numerator, a slash (D, and a denominator. When the whole number is 0, just the Fraction part of the value should be displayed (for example, 1/2 instead of 0 1/2). If the numerator is 0, just the whole number should be displayed (for example, 2 instead of 2 0/3). b. Add an operator*() method to the Fraction class created in Exercise 11a so that it correctly multiplies two Fractions. The result should be in proper, reduced format. Demonstrate that the method works correctly in a program named FractionDemo2. c. Write a program named FractionDem03 that includes an array of four Fractions. Prompt the user for values for each. Display every possible combination of addition results and every possible combination of multiplication results for each Fraction pair (that is, each type will have 16 results).arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
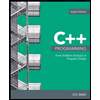
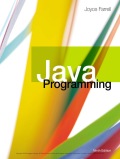
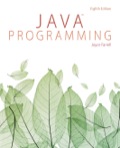
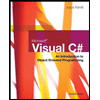