Functions for operating on strings are provided with the standard C library; however, we would like to be able to treat strings in our programs as if they were a built-in type. Thus, in this exercise you are to develop a software package in C that implements the STRING Abstract Data Type (ADT). This ADT should at the minimum support the following operations: Retrieve (i, s). Returns element I of string s. Print(s). Prints the contents of string s. Concatenate (s1, s2). Concatenates strings s1and s2and returns the resulting string. Copy (s1, s2). Copies the contents of string s1to string s2. Compare (s1, s2). Compares string s1 to string s2, returning a result indicating whether s1 is lexicographically greater than s2. Length (s). Returns the number of characters in the string s. Capacity (s). Returns the maximum numbers of characters that can be stored in the string s. Proper data encapsulation requires that a structure be created that holds the string representation, as well as information about the string. The following declaration and structure should be placed in a header file called String.h along with the function prototypes for the operations listed above: typedef struct { char *element; int length; int capacity; } string_type; /* function prototypes */ string_type Create(char *ary, int capacity); void Destroy(string_type str); void Print(string_type str); string_type Concatenate(string_type str1, string_type str2); ...... A code fragment that demonstrates how the string package will be used in given below: #include “String.h” char a[] = “hello”; char b[] = “hi!”;main() { string_type str1, str2; str1 = Create(a, 15); str2 = Create(b, 15); Print(Concatenate(str1, str2)); ...... } Use your string package to perform the following task: Sort the following strings: defunct, abeyance, irrelevant, acquiesce, western, zealous, electric, trihedral, unimodal, vanquish, ambient, abbreviate, edifice, genre, ferrous, breve, brocade.
Functions for operating on strings are provided with the standard C library; however, we would like to be able to treat strings in our programs as if they were a built-in type. Thus, in this exercise you are to develop a software package in C that implements the STRING Abstract Data Type (ADT). This ADT should at the minimum support the following operations:
- Retrieve (i, s). Returns element I of string s.
- Print(s). Prints the contents of string s.
- Concatenate (s1, s2). Concatenates strings s1and s2and returns the resulting string.
- Copy (s1, s2). Copies the contents of string s1to string s2.
- Compare (s1, s2). Compares string s1 to string s2, returning a result indicating whether s1 is lexicographically greater than s2.
- Length (s). Returns the number of characters in the string s.
- Capacity (s). Returns the maximum numbers of characters that can be stored in the string s.
Proper data encapsulation requires that a structure be created that holds the string representation, as well as information about the string. The following declaration and structure should be placed in a header file called String.h along with the function prototypes for the operations listed above:
typedef struct {
char *element;
int length;
int capacity;
} string_type;
/* function prototypes */
string_type Create(char *ary, int capacity);
void Destroy(string_type str);
void Print(string_type str);
string_type Concatenate(string_type str1, string_type str2);
......
A code fragment that demonstrates how the string package will be used in given below:
#include “String.h”
char a[] = “hello”;
char b[] = “hi!”;main()
{
string_type str1, str2;
str1 = Create(a, 15);
str2 = Create(b, 15);
Print(Concatenate(str1, str2));
...... }
Use your string package to perform the following task:
Sort the following strings: defunct, abeyance, irrelevant, acquiesce, western, zealous, electric, trihedral, unimodal, vanquish, ambient, abbreviate, edifice, genre, ferrous, breve, brocade.

Step by step
Solved in 3 steps with 3 images

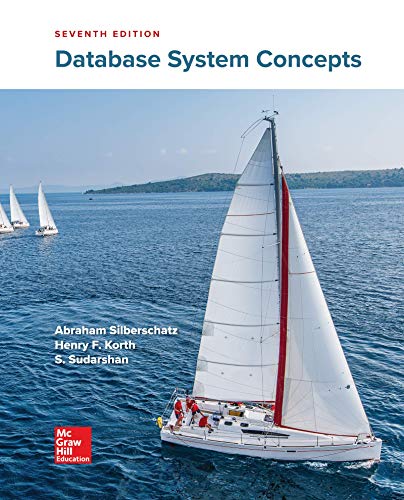
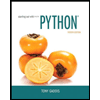
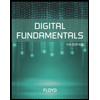
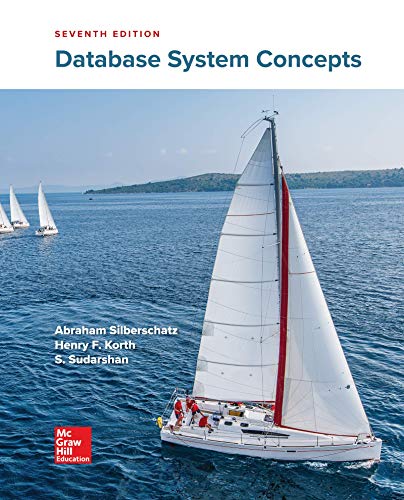
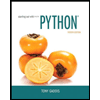
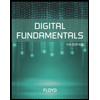
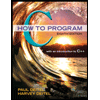
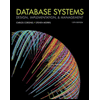
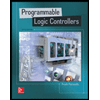