For the following code which I have, I cannot discern why the output is 0 when the expected output is meant to be 30. I would greatly appreciate it if you could walk me through it so that we reach a final consensus of what the code should be. It would refine my knowledge regarding stacks. Thanks: def evaluate_expression_iter(expr_list): if not isinstance(expr_list, list): return expr_list stack = [] for token in expr_list: if isinstance(token, (int, float)): stack.append(token) elif token in ['+', '-', '*', '/']: if len(stack) < 2: return 0.0 # Insufficient operands operand2 = stack.pop() operand1 = stack.pop() if token == '+': result = operand1 + operand2 elif token == '-': result = operand1 - operand2 elif token == '*': result = operand1 * operand2 elif token == '/': if operand2 == 0: return 0.0 # Division by zero result = operand1 / operand2 stack.append(result) else: return 0.0 # Invalid token if len(stack) == 1: return stack[0] else: return 0.0 # Invalid expression # Test the function with the provided expressions expr_lists = [ ['*', ['/', 4, 2], ['+', 8, 7]], ['-', ['+', 3, 4], ['', ['+', 2, 5], ['', 3, 3]]], ['-', ['+', ['*', 3, 3], 4], ['', ['+', ['+', 8, 7], 5], ['*', 3, 3]]], ['+', 12, ['+', ['*', 4, 6], ['/', 12, 2]] ]] for i, expr in enumerate(expr_lists): result = evaluate_expression_iter(expr) print(f"Result for expression {i}: {result}")
For the following code which I have, I cannot discern why the output is 0 when the expected output is meant to be 30. I would greatly appreciate it if you could walk me through it so that we reach a final consensus of what the code should be. It would refine my knowledge regarding stacks. Thanks:
def evaluate_expression_iter(expr_list): if not isinstance(expr_list, list): return expr_list stack = [] for token in expr_list: if isinstance(token, (int, float)): stack.append(token) elif token in ['+', '-', '*', '/']: if len(stack) < 2: return 0.0 # Insufficient operands operand2 = stack.pop() operand1 = stack.pop() if token == '+': result = operand1 + operand2 elif token == '-': result = operand1 - operand2 elif token == '*': result = operand1 * operand2 elif token == '/': if operand2 == 0: return 0.0 # Division by zero result = operand1 / operand2 stack.append(result) else: return 0.0 # Invalid token if len(stack) == 1: return stack[0] else: return 0.0 # Invalid expression # Test the function with the provided expressions expr_lists = [ ['*', ['/', 4, 2], ['+', 8, 7]], ['-', ['+', 3, 4], ['', ['+', 2, 5], ['', 3, 3]]], ['-', ['+', ['*', 3, 3], 4], ['', ['+', ['+', 8, 7], 5], ['*', 3, 3]]], ['+', 12, ['+', ['*', 4, 6], ['/', 12, 2]] ]] for i, expr in enumerate(expr_lists): result = evaluate_expression_iter(expr) print(f"Result for expression {i}: {result}")

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

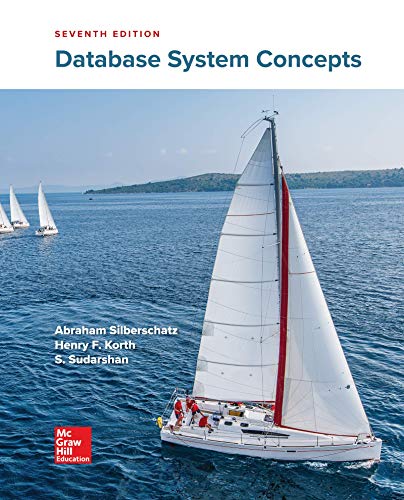
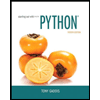
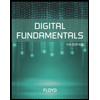
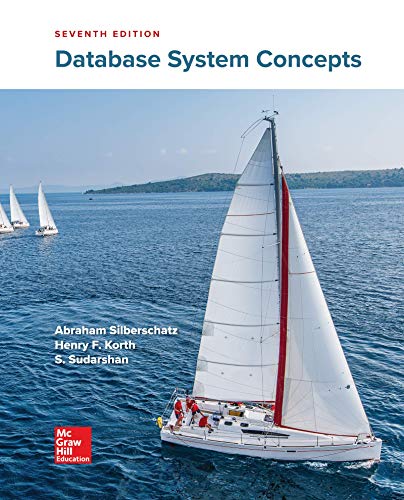
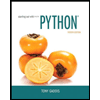
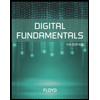
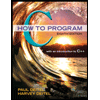
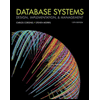
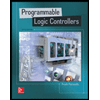