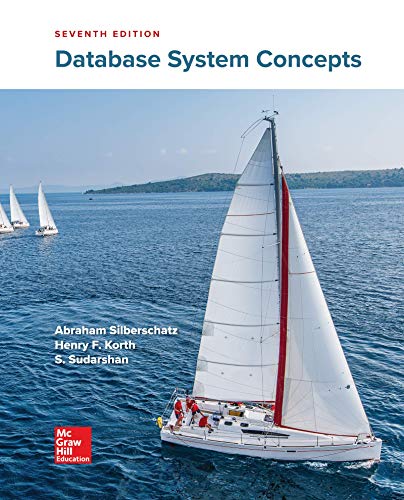
For our homework this week we have two problem. The first one reinforces the concepts in chapter 2. The second one deals with concepts from chapter 3. 1. Your solution to this question must conform to the coding rules restrictions shown at the top of page 129. These are as follows. Assumptions Integers are represented in two’s complement form Right shifts of signed data are performed arithmetically Data type int is w bits long. For some problems you will be given a specific value for w, but otherwise your code should work as long as w is a multiple of 8. You can use the expression sizeof(int)<<3 to compute w. Forbidden Conditionals (if or ?:), loops, switch statements, function calls, and macro invocations. Division, modulus, and multiplication. Relative comparison operators ( >, <, <=, and >=) Allowed operations All bit level and logic operations Left and right shifts, but only with shift amounts between 0 and w - 1 Addition and subtraction Equality (==) and inequality (!=) tests Integer constants INT_MIN and INT_MAX Casting between data types int and unsigned, either explicitly or implicitly Write C expressions that evaluate to 1 when the following conditions are true and to 0 when they are false. Assume x is of type int A. Any bit of x equals 1 B. Any bit of x equals 0 C. Any bit in the least significant byte of x equals 1 D. Any bit in the most significant byte of x equals 0 2. The coding restrictions for the previous question do not apply to this question. For the function skeleton long decode2(long x, long y, long z) { long t1 = ? ; long t2 = ? ; long t3 = ? ; long t4 = ? ; return t4; } The parameters x, y, and z are passed in registers %rdi, %rsi, and %rdx. The code stores the return value in register %rax. Suppose the gcc compiler generates the following code subq %rdx, %rsi movq %rsi, %rax imulq %rdi, %rsi salq $63, %rax sarq $63, %rax xorq %rsi, %rax ret Copy the function skeleton to your solutions file and fill in the C code that would have been where the question marks are shown.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Consider a 16-bit binary floating point number representation system: + SE E EEEE m m m m The first bit of the exponent is dedicated to its sign. Assume that the mantissa must start with a '1'. Use this system to answer the following questions: 1. What is the smallest (magnitude) number that can be represented with this system? 2. Calculate the relative round-off error introduced when this system tries to represent e = 2.718281828459045235360287 .. using rounding.arrow_forwardThe assignment is to create a MIPS program that demonstrates that the associative law fails in addition for floating point numbers (single or double precision). You only need to demonstrate it for single precision. Remember the associative law is a + (b + c) = (a + b) + c. The key is to have two of the number large (one positively and one negatively but equal in magnitude) floating point numbers and the third floating point number very small in comparison. ***Please make sure you include comments in every field*** Note: make sure the program operats and doesnt run with errors like: operand is of incorrect type or not formattedarrow_forwardAssume we are using the simple model for floating-point representation discussed in the class (the representation uses a 14-bit format, 5 bits for the exponent with an Excess-M, a significand of 8 bits, and a single sign bit for the number): Convert -43.0735 to the floating-point binary representation. (Remember we learned "implied one" format in the lecture)arrow_forward
- Consider an 8-bit floating point format. In this representation 4 bits are reserved for the mantissa and 3 bits are reserved for the exponent and 1 bit is reserved for the sign of the number. The exponent of the number is stored with a bias = 3. Express the decimal number 4.75 in this 8 bit floating point format. Write down the next bigger representable number in decimal base. B If you would be conducting the following operation using this 8 bit floating point format what would the result of the following calculation be ? 4.75 + 0.05 - 4.75 Express the decimal number 4.75 in this 8 bit floating point format. [10] b) Write down the next bigger representable number in decimal base. [7.5] c) If you would be conducting the following operation using this 8 bit floating point format what would the result of the following calculation be ? 4.75 + 0.05 - 4.75arrow_forwardPlease help me answer the question 3a and 3b in the image with details on how to do it. Thank youarrow_forwardSelect the correct statement(s) regarding the difference between analog and digital signals. a. analog signals are used more extensively in modern computer systems compared to digital signals b. digital signals are represented by logical and discrete values (i.e., “0” and “1”) and therefore logical bits must be grouped together to represent symbols c. digital signals are more accurate than analog signals; this is why digital music sounds much better d. analog networks easily fit into the OSI Reference Modelarrow_forward
- Answer the given question with a proper explanation and step-by-step solution. Consider a Hamming (7.4) code in which the parity bits are calculated according to the following equations: r0 = a0 + a1 + a2 r1 = a1 + a2 + a3 r2 = a0 + a1 + a3 The following dataword is to be coded with this scheme: format: a3 a2 a1 a0 0010 What is the parity code for the above dataword? Show it in decimal format for (r2,r1,r0); e.g. if the resulting bits are r2=1, r1=0, r0=0 (100), type the decimal equivalent of 100, which is 4.arrow_forward4, in the fixed-point machine, the following statement is wrong ( ).A. In addition to the complement, the original code and the inverted code cannot indicate that the original code of −1 B. +0 is not equal to −0C. the inverse of +0 is not equal to the negative of −0 D. For the same machine word length, the complement code can represent a negative number more than the original code and the inverted code.arrow_forwardVI. Floating point representation Consider a 12-bit variant of the IEEE floating point format as follows: • Sign bit 5-bit exponent with a bias of 15. • 6-bit significand All of the rules for IEEE 754 Standard apply. Fill in the numeric value represented by the following bit patterns. You must write your number in decimal form (e.g. 0.0146485375, -0.0146485375). Bit Pattern 010011101110 111011101011 100101001111 001010111010 Numerical Valuearrow_forward
- Use C++ coding language and provide a code that works properly input.txt 4 51 0 1 0 01 0 1 1 11 1 1 1 11 0 0 1 05 71 0 1 0 0 1 11 0 1 1 1 1 11 1 1 1 1 0 11 0 1 1 1 0 11 0 1 0 1 0 10arrow_forwardConvert the decimal number -43.68 to a floating-point number expressed in the 14-bit simple model given in your textbook. Note: in the 14-bit simple model, the left-most bit is the sign, followed by 5 bits for the exponent, followed by 8 bits for the mantissa (There are no implied bits). The exponent is in Excess 15 notation. 101011.1010arrow_forwardAn interesting application of a PLA is conversion from the old, obsolete punched cards character codes to ASCII codes. The standard punched cards that were so pop- ular with computers in the past had 12 rows and 80 columns where holes could be punched. Each column corresponded to one character. so each character had a 12-bit code. However, only 96 characters were actually used. Consider an application that reads punched cards and converts the character codes to ASCII. a. Describe a PLA implementation of this application. b. Can this problem be solved with a ROM? Explain.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
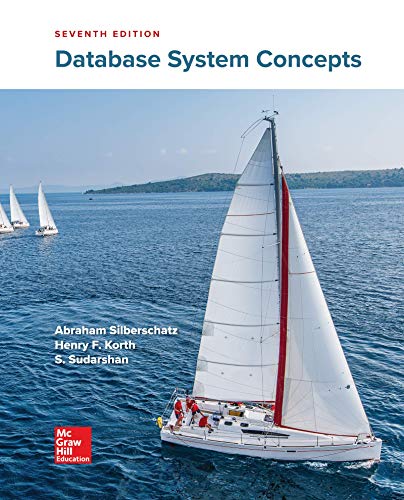
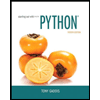
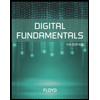
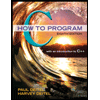
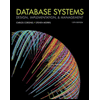
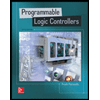