follows: Mark all bit positions that are powers of two as
Assignment for Computer Architecture! this is about hamming codes
write the code IN MIPS ASSEMBLY LANGUAGE
calculating hamming codes;
The key to the Hamming Code is the use of extra parity bits to allow the identification of a single error. Create the code word as follows:
-
Mark all bit positions that are powers of two as parity bits. (positions 1, 2, 4, 8, 16, 32, 64, etc.)
-
All other bit positions are for the data to be encoded. (positions 3, 5, 6, 7, 9, 10, 11, 12, 13, 14, 15, 17, etc.)
-
Each parity bit calculates the parity for some of the bits in the code word. The position of the parity bit determines the sequence of bits that it alternately checks and skips.
Position 1: check 1 bit, skip 1 bit, check 1 bit, skip 1 bit, etc. (1,3,5,7,9,11,13,15,...)
Position 2: check 2 bits, skip 2 bits, check 2 bits, skip 2 bits, etc. (2,3,6,7,10,11,14,15,...)
Position 4: check 4 bits, skip 4 bits, check 4 bits, skip 4 bits, etc. (4,5,6,7,12,13,14,15,20,21,22,23,...)
Position 8: check 8 bits, skip 8 bits, check 8 bits, skip 8 bits, etc. (8-15,24-31,40-47,...)
Position 16: check 16 bits, skip 16 bits, check 16 bits, skip 16 bits, etc. (16-31,48-63,80-95,...)
Position 32: check 32 bits, skip 32 bits, check 32 bits, skip 32 bits, etc. (32-63,96-127,160-191,...)
etc. -
Set a parity bit to 1 if the total number of ones in the positions it checks is odd. Set a parity bit to 0 if the total number of ones in the positions it checks is even.
The assignment is to create a MIPS programs that the determines what the ECC code should be for a given number (an 8-bit byte). ECC Hamming codes are explained on pages 420-424 in your text. The codes you create are to work for 8-bit positive numbers as these are simpler to work with than larger numbers.
The program is to request the user to enter a byte of data (a positive integer in the range of 0 to 255 in decimal) and then create the 12-bit Hamming code as described in your text (see above). The program is to then output this (with an appropriate label) in hex.
Make certain that you have lots of comments in your code as this is in MIPS. Also make the code neat: line up the instruction columns, the register columns, and the comment fields (see page 134 in your text for a nice example).
For this assignment, turn in your code, a screenshot showing a working for a test case

Step by step
Solved in 3 steps

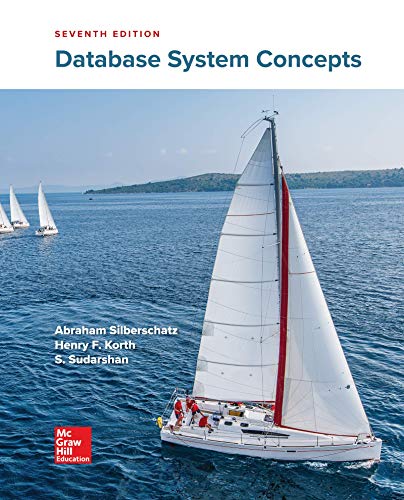
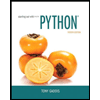
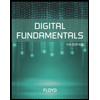
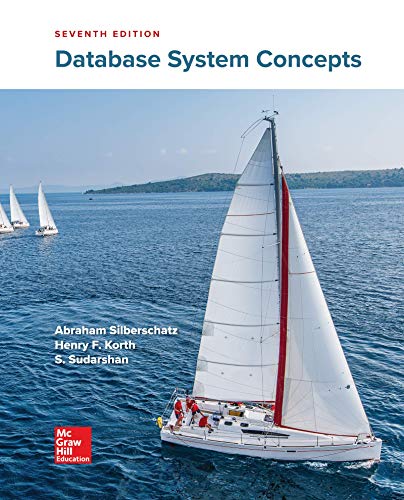
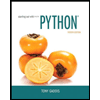
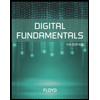
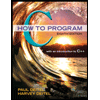
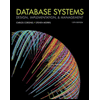
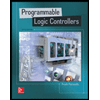