Fix error codes in "the largest file" include commentsDirectoryController.java:15: errorDirectoryController.java:25 modify code to sucessfully compile Include GUI components to display user input errors show local time for the "Last Modified" time stamp. "LargestFileA7.java" public class LargestFileA7 { public static void main(String[] args) { // Create instances of the model, view, and controller DirectoryModel model = new DirectoryModel(); DirectoryView view = new DirectoryView(); DirectoryController controller = new DirectoryController(model, view); }}"DirectoryView.java" import javax.swing.*;import javax.swing.border.EmptyBorder;import java.awt.*;import java.awt.event.*;import java.io.IOException;import java.nio.file.*;import java.text.SimpleDateFormat;import java.util.Date; public class DirectoryView extends JFrame { private JTextField directoryField; private JTextArea resultArea; private JButton browseButton; public DirectoryView() { setTitle("Directory Explorer"); setSize(400, 300); setDefaultCloseOperation(EXIT_ON_CLOSE); JPanel panel = new JPanel(); panel.setLayout(new BorderLayout()); JLabel directoryLabel = new JLabel("Enter Directory Path:"); directoryField = new JTextField(20); browseButton = new JButton("Browse"); browseButton.addActionListener(new BrowseListener()); resultArea = new JTextArea(10, 30); resultArea.setEditable(false); JPanel inputPanel = new JPanel(new BorderLayout()); inputPanel.add(directoryLabel, BorderLayout.WEST); inputPanel.add(directoryField, BorderLayout.CENTER); inputPanel.add(browseButton, BorderLayout.EAST); panel.add(inputPanel, BorderLayout.NORTH); JScrollPane scrollPane = new JScrollPane(resultArea); panel.add(scrollPane, BorderLayout.CENTER); add(panel); setVisible(true); } // Method to get the directory path entered by the user public String getDirectoryPath() { return directoryField.getText(); } // Method to display the result in the text area public void displayResult(String result) { resultArea.setText(result); } // Method to display error message in a dialog box public void displayErrorMessage(String message) { JOptionPane.showMessageDialog(this, message, "Error", JOptionPane.ERROR_MESSAGE); } // Method to disable the Browse button during file traversal public void disableBrowseButton() { browseButton.setEnabled(false); } // Method to enable the Browse button after file traversal public void enableBrowseButton() { browseButton.setEnabled(true); } // ActionListener for Browse button class BrowseListener implements ActionListener { public void actionPerformed(ActionEvent e) { // Action to perform when Browse button is clicked JFileChooser fileChooser = new JFileChooser(); fileChooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY); int returnValue = fileChooser.showOpenDialog(null); if (returnValue == JFileChooser.APPROVE_OPTION) { directoryField.setText(fileChooser.getSelectedFile().getAbsolutePath()); } } }} Please complete "DirectoryModel.Java" import java.nio.file.*;import java.nio.file.attribute.BasicFileAttributes;import java.io.IOException; public class DirectoryModel { private Path largestFilePath; private long largestFileSize; public DirectoryModel() { largestFilePath = null; largestFileSize = 0; } public void findLargestFile(Path directoryPath) { try { Files.walkFileTree(directoryPath, new SimpleFileVisitor<Path>() { @Override public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException { if (attrs.size() > largestFileSize) { largestFileSize = attrs.size(); largestFilePath = file; } return FileVisitResult.CONTINUE; Please include the "DirectoryController.java" file
Fix error codes in "the largest file" include comments
DirectoryController.java:15: error
DirectoryController.java:25
- modify code to sucessfully compile
- Include GUI components to display user input errors
- show local time for the "Last Modified" time stamp.
"LargestFileA7.java"
public class LargestFileA7 {
public static void main(String[] args) {
// Create instances of the model, view, and controller
DirectoryModel model = new DirectoryModel();
DirectoryView view = new DirectoryView();
DirectoryController controller = new DirectoryController(model, view);
}
}
"DirectoryView.java"
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
import java.awt.event.*;
import java.io.IOException;
import java.nio.file.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class DirectoryView extends JFrame {
private JTextField directoryField;
private JTextArea resultArea;
private JButton browseButton;
public DirectoryView() {
setTitle("Directory Explorer");
setSize(400, 300);
setDefaultCloseOperation(EXIT_ON_CLOSE);
JPanel panel = new JPanel();
panel.setLayout(new BorderLayout());
JLabel directoryLabel = new JLabel("Enter Directory Path:");
directoryField = new JTextField(20);
browseButton = new JButton("Browse");
browseButton.addActionListener(new BrowseListener());
resultArea = new JTextArea(10, 30);
resultArea.setEditable(false);
JPanel inputPanel = new JPanel(new BorderLayout());
inputPanel.add(directoryLabel, BorderLayout.WEST);
inputPanel.add(directoryField, BorderLayout.CENTER);
inputPanel.add(browseButton, BorderLayout.EAST);
panel.add(inputPanel, BorderLayout.NORTH);
JScrollPane scrollPane = new JScrollPane(resultArea);
panel.add(scrollPane, BorderLayout.CENTER);
add(panel);
setVisible(true);
}
// Method to get the directory path entered by the user
public String getDirectoryPath() {
return directoryField.getText();
}
// Method to display the result in the text area
public void displayResult(String result) {
resultArea.setText(result);
}
// Method to display error message in a dialog box
public void displayErrorMessage(String message) {
JOptionPane.showMessageDialog(this, message, "Error", JOptionPane.ERROR_MESSAGE);
}
// Method to disable the Browse button during file traversal
public void disableBrowseButton() {
browseButton.setEnabled(false);
}
// Method to enable the Browse button after file traversal
public void enableBrowseButton() {
browseButton.setEnabled(true);
}
// ActionListener for Browse button
class BrowseListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
// Action to perform when Browse button is clicked
JFileChooser fileChooser = new JFileChooser();
fileChooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
int returnValue = fileChooser.showOpenDialog(null);
if (returnValue == JFileChooser.APPROVE_OPTION) {
directoryField.setText(fileChooser.getSelectedFile().getAbsolutePath());
}
}
}
}
Please complete "DirectoryModel.Java"
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.io.IOException;
public class DirectoryModel {
private Path largestFilePath;
private long largestFileSize;
public DirectoryModel() {
largestFilePath = null;
largestFileSize = 0;
}
public void findLargestFile(Path directoryPath) {
try {
Files.walkFileTree(directoryPath, new SimpleFileVisitor<Path>() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
if (attrs.size() > largestFileSize) {
largestFileSize = attrs.size();
largestFilePath = file;
}
return FileVisitResult.CONTINUE;
Please include the "DirectoryController.java" file

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

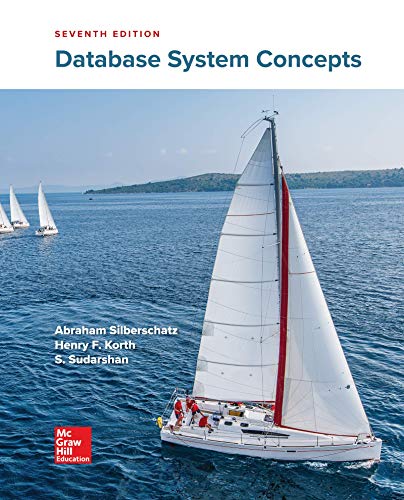
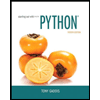
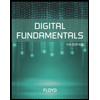
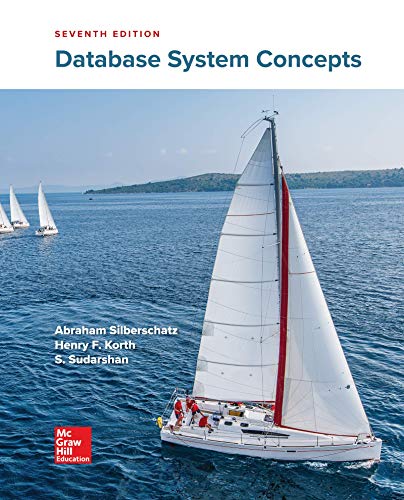
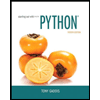
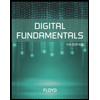
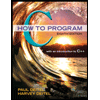
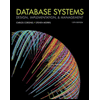
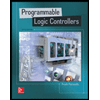