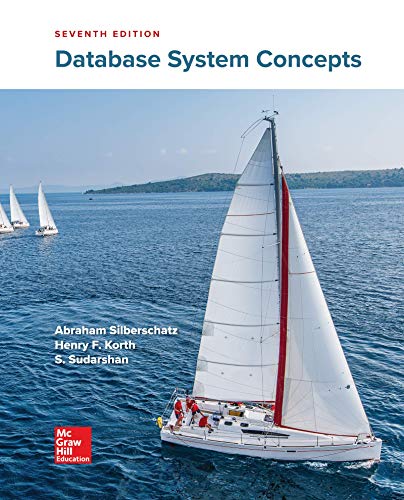
First, launch NetBeans and close any previous projects that may be open (at the top menu go to File ==> Close All Projects).
Then create a new Java application called "WeightedAvgDataAnalyzer" (without the quotation marks), that modifies the DataAnalyzer.java in Horstmann Section 7.5, pp. 350-351 according to the specifications below.
The input file should be called 'data.txt' and should be created according to the highlighted instructions below. Note that even though you know the name of the input file, you should not hard-code this name into your program. Instead, prompt the user for the name of the input file.
The input file should contain (in order): the weight (a number greater than zero and less than or equal to 1), the number, n, of lowest numbers to drop, and the numbers to be averaged after dropping the lowest n values.
You should also prompt the user for the name of the output file, and then print your results to an output file with the name that the user specified.
- Your program should allow the user to re-enter the input file name if one or more of the exceptions in the catch clauses are caught.
- Your methods for getting data and printing results should each throw a FileNotFoundException which should be caught in the main method.
- Use try-with-resources statements (Links to an external site.) in your methods for getting and printing the data, and so avoid the need to explicitly close certain resources.
- In your readData method, use hasNextDouble to check ahead of time whether there's a double in the data. That way when you try to get the nextDouble, your code won't throw a NoSuchElementException.
You can use a writeFile method that does all the work (i.e., does not call a writeData method the way that Horstmann’s readFile method calls a readData method). Use a try-with-resources statement in your writeFile method when creating a new PrintWriter.
The inputValues come from a single line in a text file (data.txt) such as the following:
0.5 3 10 70 90 80 20
The output in the output file must give the weighted average, the data and weight that were used to calculate the weighted average, and the number of values dropped before the weighted average was calculated.
Your output should look very much like the following: "The weighted average of the numbers is 42.5, when using the data 10.0, 70.0, 90.0, 80.0, 20.0, where 0.5 is the weight used, and the average is computed after dropping the lowest 3 values."
Write the output to a file with the filename that the user chose to name the output file (e.g., output.txt). Don't hard-code the output file name in your program.
Creating the Input File
To create the input file, while in NetBeans with your project open, first click to highlight the top-level folder of your project, which should be called WeightedAvgDataAnalyzer.
Then from the File menu do this:
Keep the Project name at the top; keep Filter blank
Categories choose Other (at the bottom of the categories list)
File Types choose Empty File (at the bottom of the files list)
Next->
FileName: data.txt
Folder: this should be blank; if it's not, delete whatever's there.
Finish
In the empty file data.txt that you just created, add a single line of data like that shown in the example above, where the weight is a double (greater than 0.0 and less than or equal to 1.0) and the other numbers are the number, n, of lowest values to drop and then the numbers to be averaged after dropping the lowest n values.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- I'm not sure how to fix my error import csv #This is the classclass ConvertCSVToJSON: def __init__(self, headings, ID, linesFromFile, inputFileName, outputFileName="Project7Output.txt"): self.headings=headings # Headings (row 1 of the file) self.ID=ID self.linesFromFile=linesFromFile # rows2-end of file (EOF) self.outputFileName=outputFileName self.inputFileName=inputFileName def createKeyValuePair(self, key, value): # Think about parameters and how the code will work result="\""+key+"\""+":"+value return result #create a line function/method in class to have access to headings and file. def createLine(self, ID, line): #splits the line into different components lineComponets=line.split(",") dictionary={}#creats a dictionary for the componets for i in range(len(self.heading)): dictionary[self.headings[i]]=lineComponents[i] #adds ID as a keyvalue pair dictionary["ID"]=str(ID) #should convert to json…arrow_forwardThere is a csv file called Air_Quality.csv. It has 12 columns and 17,000 rows. One column titled 'Name' which has a various emissions listed in it. Another column titled 'Geo Place Name' has a list of cities. Write a code (in the most basic Python) which finds the two emissions called PM2.5-Attributable Respiratory Hospitalizations (Adults 20 Yrs and Older) and PM2.5-Attributable Respiratory Hospitalizations (Adults 40 Yrs and Older)within the column and prints out which city has the respiratory hospitalizations for both 20 years and older and 40 years and older based on a column called 'Data Value' Below is a picture of a small chunk of the csv file.arrow_forwardin java but don't write as a GUI (Graphical User Interface) Write a program that reads a file named input.txt and writes a file that contains the same contents, but is named output.txt. The input file will contain more than one line when I test this and so should your output file. Do not use a path name when opening these files. This means the files should be located in the top level folder of the project. This would be the folder that contains the src folder, probably named FileCopy depending on what name you gave the project. Do not use a copy method that is supplied by Java. Your program must read the file line by line and write the file itself. Class should be named FileCopy do not write to input.txt!arrow_forward
- Before starting this question, first, download the data file pokemonTypes.txt from the class Moodle. Make sure to put it in the same folder as your a5.py python code. Write a function called read_pokedata() that takes as parameter(s): • a string indicating the name of a pokemon type data file This function should return a database (i.e. a dictionary-of-dictionaries) that stores all of the Pokemon data in a format that we will describe further below. You can review section 11.1.9 of the text for a refresher on what databases look like using dictionaries. Input File Format The data file for this question looks like this: bulbasaur,grass,South America ivysaur,grass,Asia,Antarctica Each line of the file contains all of the data for a single pokemon. The first item on the line is always the pokemon's name; names are guaranteed to be unique. The second item is always the pokemon's type, which in the example above, is the type grass. Following that are one or more continents where that…arrow_forwardA miniature robot designed to mimic the behavior of an ant is being tested to evaluate the robot’s ability to avoid a chemical repellant (the robot has a chemical sensor, and a control loop that makes the robot avoid moving in a direction that will result in the sensor detecting a chemical concentration above a certain limit). The x and y positions of the robot are measured with time, and the resulting data is saved to a file called data.txt which contains 3 columns: the time of the measurement (in seconds), the x position (in cm), and the y position (in cm). The first line of the file is a “header”: it has a single integer that specifies how many lines of data follow. Write a C++ program called ant.cpp that reads files of this type (i.e., your program should work with another, but similar, file!) and then does the following: it asks for an X and Y position, and then prints to screen the time at which the ant robot was closest to this position. For example, if we wish to know when the…arrow_forwardA debugging question, thank you!!arrow_forward
- No written by hand solutionarrow_forwardIn c++ Create a new project named lab9_2. You will continue to use the Courses class, but this time you will create a vector of Courses. The file you will read from is below: 6CSS 2A 1111 35CSS 2A 2222 20CSS 1 3333 40CSS 1 4444 33CSS 3 5555 15CSS 44 6666 12 Read this information into a vector of Courses. Then, print out a summary of your vector. Here's a sample driver: #include <iostream>#include <string>#include <fstream>#include <vector>#include <cstdlib>#include "Course.h"using namespace std;int main(){vector<Course> myclass;string dep, c_num;int classes, sec, num_stus;ifstream fin("sample.txt");if (fin.fail()){cout << "File path is bad\n";exit(1);}fin >> classes;for (int i = 0; i < classes; i++){fin >> dep >> c_num >> sec >> num_stus;// Now how do you create a Course object// that contains the information you just read in// and add it to your myclass vector?}cout << "Here are the college courses: "…arrow_forwardJava : Create a .txt file with 3 rows of various movies data of your choice in the following table format: Movie ID number of viewers rating release year Movie name 0000012211 174 8.4 2017 "Star Wars: The Last Jedi" 0000122110 369 7.9 2017 "Thor: Ragnarok" Create a class Movie which instantiates variables corresponds to the table columns. Implement getters, setters, constructors and toString. Implement 2 readData() methods : the first one will return an array of Movies and the second one will return a List of movies . Test your methods.arrow_forward
- This project involves generating a boarding pass ticket and storing it in a file. The application should take the passenger details as input. The details of the boarding pass are to be stored in a file. The details should include valid data such as: name, email, phone number, gender, age, boarding pass number, date, origin, destination, estimated time of arrival (ETA), departure time. The application should generate a boarding pass ticket using the boarding pass details. The generated ticket should contain the following information: Boarding Pass Number, Date, Origin, Destination, Estimated time of arrival (ETA), Departure Time Name, Email, Phone Number, Gender, Age Total Ticket Price The user will be required to enter their Name, Email, Phone Number, Gender, Age, Date, Destination, and Departure Time into the console or GUI. From the input the computer must generate the ETA and Ticket Price. The computer must generate the boarding pass number ensuring the number is unique. All…arrow_forwardThe downloads include a python starter program (P9HashTable1.py) that reads an employee file, sets upthe data structures and then allows the user to search, add or delete employees from the database. Youmust store the employee file (EmployeeDB.csv) in the same folder as your python program. The pythonstarter program has some missing functions that you must provideThe deliverables this week are:1. A search function that will find an employee record based on the employee ID.• Use a hash function Mod(12) to convert empID to a position in the Hash Dictionary• Using the beginChain value in the Hash Dictionary, traverse the Employee linked list2. An insert function that will add a new employee to the database.• Check to see if this is the first node of the chain and process differently if it is3. A delete function that will remove an employee from the database.• You’re not physically removing the employee record, you’re just using the ptr to passover records that are deleted.4. After a…arrow_forwardNEED HELP DEBUGGING THE FOLLOW JS CODE JS CODE /* JavaScript 7th Edition Chapter 3 Project 03-05 Application to generate a horizontal bar chart Author: Date: Filename: project03-05.js*/ // Array of phone models sold by the companylet phones = ("Photon 6E", "Photon 6X", "Photon 7E", "Photon 7X", "Photon 8P"); // Units sold in the previous quarterlet sales = (10281, 12255, 25718, 21403, 16142); // Variable to calculate total saleslet totalSales = 0; // Use the forEach() method to sum the sales for each phone model and add it to the totalSales variablesales.forEach(addtoTotal); // For loop to generate bar chart of phone salesfor (let i = 1; i <= phones.length; i++) { let barChart = ""; // Variable to store HTML code for table cells used to create bar chart // Calculate the percent of total sales for a particular phone model let barPercent = 100*sales/totalSales; let cellTag; // Variable that will define the class of td…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
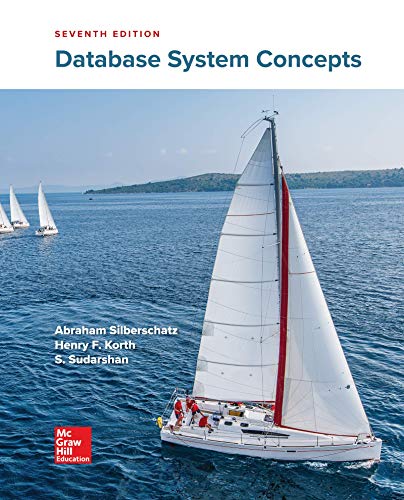
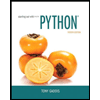
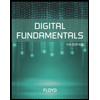
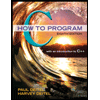
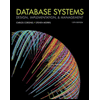
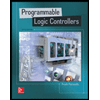