Ex. If the input is: The Hobbit J. R. R. Tolkien George Allen & Unwin 21 September 1937 The Illustrated Encyclopedia of the Universe James W. Guthrie Watson-Guptill 2001 2nd 1 the output is: Book Information: Book Title: The Hobbit Author: J. R. R. Tolkien Publisher: George Allen & Unwin Publication Date: 21 September 1937 Book Information: Book Title: The Illustrated Encyclopedia of the Universe Author: James W. Guthrie Publisher: Watson-Guptill Publication Date: 2001 Edition: 2nd Number of Volumes: 1 Note: Indentations use 3 spaces. import java.util.Scanner; public class BookInformation public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Book myBook = new Book(); Encyclopedia myEncyclopedia = new Encyclopedia(); string title, author, publisher, publicationDate; string eTitle, eAuthor, ePublisher, ePublicationDate, edition; int numvolumes; title= scnr.nextLine(); author = scnr.nextLine(); publisher scnr.nextLine(); publicationDate = scnr.nextLine(); eTitle scnr.nextLine(); eAuthor scnr.nextLine(); ePublisher scnr.nextLine(); ePublicationDate = scnr.nextLine(); edition scnr.nextLine(); numvolumes scnr.nextInt(); myBook.setTitle(title); myBook.setAuthor (author); myBook.setPublisher (publisher); myBook.setPublicationDate(publicationDate); myBook.printInfo(); myEncyclopedia.setTitle(eTitle); myEncyclopedia.setAuthor (eAuthor); myEncyclopedia.setPublisher(ePublisher); myEncyclopedia.setPublicationDate(ePublicationDate); myEncyclopedia.setEdition(edition); myEncyclopedia.setNumvolumes (numvolumes); myEncyclopedia.printInfo(); public class Book protected string title; protected string author; protected string publisher; protected string publicationDate; Read Only public void setTitle(String userTitle) { title= userTitle; } public string getTitle() { return title; } public void setAuthor(String userAuthor) { author userAuthor; } public string getAuthor() { return author; = } public void setPublisher (String userPublisher) { publisher userPublisher; Read Only } public string getPublicationDate() { return publicationDate; } public string getPublisher() { return publisher; } public void setPublicationDate(String userPublicationDate) { publicationDate = userPublicationDate; } public void printInfo() { System.out.println("Book Information: "); Author: " + author); System.out.println(" Book Title: " + title); System.out.println(" Author: System.out.println(" Publisher: " + publisher); System.out.println(" Publication Date: " + publicationDate);
Ex. If the input is: The Hobbit J. R. R. Tolkien George Allen & Unwin 21 September 1937 The Illustrated Encyclopedia of the Universe James W. Guthrie Watson-Guptill 2001 2nd 1 the output is: Book Information: Book Title: The Hobbit Author: J. R. R. Tolkien Publisher: George Allen & Unwin Publication Date: 21 September 1937 Book Information: Book Title: The Illustrated Encyclopedia of the Universe Author: James W. Guthrie Publisher: Watson-Guptill Publication Date: 2001 Edition: 2nd Number of Volumes: 1 Note: Indentations use 3 spaces. import java.util.Scanner; public class BookInformation public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Book myBook = new Book(); Encyclopedia myEncyclopedia = new Encyclopedia(); string title, author, publisher, publicationDate; string eTitle, eAuthor, ePublisher, ePublicationDate, edition; int numvolumes; title= scnr.nextLine(); author = scnr.nextLine(); publisher scnr.nextLine(); publicationDate = scnr.nextLine(); eTitle scnr.nextLine(); eAuthor scnr.nextLine(); ePublisher scnr.nextLine(); ePublicationDate = scnr.nextLine(); edition scnr.nextLine(); numvolumes scnr.nextInt(); myBook.setTitle(title); myBook.setAuthor (author); myBook.setPublisher (publisher); myBook.setPublicationDate(publicationDate); myBook.printInfo(); myEncyclopedia.setTitle(eTitle); myEncyclopedia.setAuthor (eAuthor); myEncyclopedia.setPublisher(ePublisher); myEncyclopedia.setPublicationDate(ePublicationDate); myEncyclopedia.setEdition(edition); myEncyclopedia.setNumvolumes (numvolumes); myEncyclopedia.printInfo(); public class Book protected string title; protected string author; protected string publisher; protected string publicationDate; Read Only public void setTitle(String userTitle) { title= userTitle; } public string getTitle() { return title; } public void setAuthor(String userAuthor) { author userAuthor; } public string getAuthor() { return author; = } public void setPublisher (String userPublisher) { publisher userPublisher; Read Only } public string getPublicationDate() { return publicationDate; } public string getPublisher() { return publisher; } public void setPublicationDate(String userPublicationDate) { publicationDate = userPublicationDate; } public void printInfo() { System.out.println("Book Information: "); Author: " + author); System.out.println(" Book Title: " + title); System.out.println(" Author: System.out.println(" Publisher: " + publisher); System.out.println(" Publication Date: " + publicationDate);
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Write in Java
Given main() and a base Book class, define a derived class called Encyclopedia. Within the derived Encyclopedia class, define a printInfo() method that overrides the Book class' printInfo() method by printing not only the title, author, publisher, and publication date, but also the edition and number of volumes.
![Ex. If the input is:
The Hobbit
J. R. R. Tolkien
George Allen & Unwin
21 September 1937
The Illustrated Encyclopedia of the Universe
James W. Guthrie
Watson-Guptill
2001
2nd
1
the output is:
Book Information:
Book Title: The Hobbit
Author: J. R. R. Tolkien
Publisher: George Allen & Unwin
Publication Date: 21 September 1937
Book Information:
Book Title: The Illustrated Encyclopedia of the Universe
Author: James W. Guthrie
Publisher: Watson-Guptill
Publication Date: 2001
Edition: 2nd
Number of Volumes: 1
Note: Indentations use 3 spaces.
import java.util.Scanner;
public class BookInformation {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Book myBook = new Book();
Encyclopedia myEncyclopedia = new Encyclopedia();
string title, author, publisher, publicationDate;
string eTitle, eAuthor, ePublisher, ePublicationDate, edition;
int numvolumes;
title= scnr.nextLine();
author scnr.nextLine();
publisher = scnr.nextLine();
publicationDate= scnr.nextLine();
eTitle scnr.nextLine();
eAuthor scnr.nextLine();
ePublisher scnr.nextLine();
ePublicationDate = scnr.nextLine();
edition scnr.nextLine();
numvolumes = scnr.nextInt();
myBook.setTitle(title);
myBook.setAuthor(author);
myBook.setPublisher (publisher);
myBook.setPublicationDate (publicationDate);
myBook.printInfo();
myEncyclopedia.setTitle(eTitle);
myEncyclopedia.setAuthor(eAuthor);
myEncyclopedia.setPublisher (ePublisher);
Read Only
myEncyclopedia.setPublicationDate(ePublicationDate);
myEncyclopedia.setEdition (edition);
myEncyclopedia.setNumvolumes (numvolumes);
myEncyclopedia.printInfo();
public class Book
protected string title;
protected string author;
protected string publisher;
protected string publicationDate;
=
Read Only
public void setTitle(String userTitle) {
title= userTitle;
}
public string getTitle() {
return title;
}
public void setAuthor(String userAuthor) {
author userAuthor;
}
public string getAuthor(){
return author;
}
public void setPublisher (String userPublisher) {
publisher = userPublisher;
}
public string getPublisher() {
return publisher;
}
public void setPublicationDate(String userPublicationDate) {
publicationDate= userPublicationDate;
}
public string getPublicationDate() {
return publicationDate;
}
public void printInfo() {
System.out.println("Book Information: ");
System.out.println(" Book Title: " + title);
System.out.println(" Author: " + author);
System.out.println(" Publisher: " + publisher);
System.out.println(" Publication Date: " + publicationDate);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1c870776-4927-4fbd-b218-f5b27740c439%2F394928f0-33c1-43d2-9068-9b0fa47faa52%2Fkrfhtc_processed.png&w=3840&q=75)
Transcribed Image Text:Ex. If the input is:
The Hobbit
J. R. R. Tolkien
George Allen & Unwin
21 September 1937
The Illustrated Encyclopedia of the Universe
James W. Guthrie
Watson-Guptill
2001
2nd
1
the output is:
Book Information:
Book Title: The Hobbit
Author: J. R. R. Tolkien
Publisher: George Allen & Unwin
Publication Date: 21 September 1937
Book Information:
Book Title: The Illustrated Encyclopedia of the Universe
Author: James W. Guthrie
Publisher: Watson-Guptill
Publication Date: 2001
Edition: 2nd
Number of Volumes: 1
Note: Indentations use 3 spaces.
import java.util.Scanner;
public class BookInformation {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Book myBook = new Book();
Encyclopedia myEncyclopedia = new Encyclopedia();
string title, author, publisher, publicationDate;
string eTitle, eAuthor, ePublisher, ePublicationDate, edition;
int numvolumes;
title= scnr.nextLine();
author scnr.nextLine();
publisher = scnr.nextLine();
publicationDate= scnr.nextLine();
eTitle scnr.nextLine();
eAuthor scnr.nextLine();
ePublisher scnr.nextLine();
ePublicationDate = scnr.nextLine();
edition scnr.nextLine();
numvolumes = scnr.nextInt();
myBook.setTitle(title);
myBook.setAuthor(author);
myBook.setPublisher (publisher);
myBook.setPublicationDate (publicationDate);
myBook.printInfo();
myEncyclopedia.setTitle(eTitle);
myEncyclopedia.setAuthor(eAuthor);
myEncyclopedia.setPublisher (ePublisher);
Read Only
myEncyclopedia.setPublicationDate(ePublicationDate);
myEncyclopedia.setEdition (edition);
myEncyclopedia.setNumvolumes (numvolumes);
myEncyclopedia.printInfo();
public class Book
protected string title;
protected string author;
protected string publisher;
protected string publicationDate;
=
Read Only
public void setTitle(String userTitle) {
title= userTitle;
}
public string getTitle() {
return title;
}
public void setAuthor(String userAuthor) {
author userAuthor;
}
public string getAuthor(){
return author;
}
public void setPublisher (String userPublisher) {
publisher = userPublisher;
}
public string getPublisher() {
return publisher;
}
public void setPublicationDate(String userPublicationDate) {
publicationDate= userPublicationDate;
}
public string getPublicationDate() {
return publicationDate;
}
public void printInfo() {
System.out.println("Book Information: ");
System.out.println(" Book Title: " + title);
System.out.println(" Author: " + author);
System.out.println(" Publisher: " + publisher);
System.out.println(" Publication Date: " + publicationDate);

Transcribed Image Text:public class Encyclopedia extends Book {
// TODO: Declare private fields: edition, numvolumes
// TODO: Define mutator methods -
//
setEdition (), setNumvolumes ()
// TODO: Define accessor methods -
getEdition (), getNumVolumes ()
// TODO: Define a printInfo() method that overrides
the printinfo in Book class
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
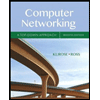
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
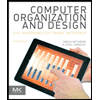
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
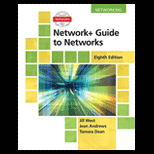
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
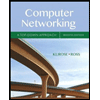
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
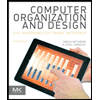
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
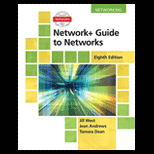
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
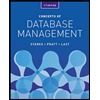
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
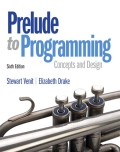
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
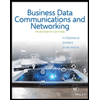
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY