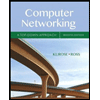
I have this code. Everything on the code runs just the way it needs to, except for one thing. It keeps looping even through the program should be over. Can anyone help figure this out. I've talked to three tutors on here and each one keeps telling me they can't help. Maybe someone else can.
#include <iostream>
#include <string>
#include <bits/stdc++.h>
using namespace std;
int binarySearch(string arrayA[], string search_value, int sizeA);
int main()
{
int sizeA = 10;
int index;
string arrayA[] = {"Mike","Joe","John","Drew","Jenn","Anne", "Susan", "Debra", "Candy", "Aaron"};
string search_value;
int number;
cout << "This program attempts to search for a user entered name." << endl;
for(index = 0; index <= sizeA - 1; index++)
{
cout << "Enter how many names you would like to search for: ";
cin >> number;
int arrayNumber[number];
for(int i=0;i<number;i++){
cout << "Please enter the names you would like to search for: ";
cin >> search_value;
int result = binarySearch(arrayA, search_value, sizeA);
if (result == -1)
cout << "The name " << search_value << " was not found within the array using binary search." << endl;
else
cout << "The name " << search_value << " was found within the array using binary search." << result;
}
}
}
int binarySearch(string arrayA[], string search_value, int sizeA)
{
sort(arrayA, arrayA + sizeA);
int left = 0 ;
int right = sizeA - 1;
while (left <= right)
{
int middle = left + (right - 1) / 2;
int res = 101;
if (search_value == (arrayA[middle]))
res = 0;
if (res == 0)
return middle;
if (search_value > (arrayA[middle]))
left = middle + 1;
else
right = middle - 1;
}
return -1;
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Help me homework c++ The shots file holds a list of shots (imagine some hitscan weapon in a video game like a shotgun or something). Each shot has an origin and a direction. The origin is an (x,y) coordinate, like (5,3). The direction is a slope and whether the shot is traveling along that slope or in the reverse. A slope of "Vertical" means that the shot is travellingstraight up and down. The format is:x_location y_location slope(either a number like 2.1 or a non-number meaning"Vertical") forwards(1 meaning forwards, 0 meaning backwards) 0 0 0 00 0 0 10 0 Vertical 00 0 Squirrel 110 10 -1 1-10.1 -100.01 2.1 0 The first line is a horizontal line shooting left from the origin (0,0).The second line is a shot also travelling horizontally from the origin, but forward along the x axis instead of backwards.The third line is shooting straight down out of the originThe fourth line is shooting straight up out of the origin (any non-number means Vertical, not just Vertical)The fifth line is…arrow_forwardI need help making this program in C programming. Please look at the pictures provided at that helps. Thank You!arrow_forwardThis assignment will give you a chance to perform some simple tasks with pointers. The tasks are only loosely related to each other. Start the assignment by copying the code below and pasting it into a .cpp file, then add statements to accomplish each of the tasks listed. Don't delete any of the given code or comments. Some of the tasks will only require a single C++ statement, others will require more than one. No documentation is required for this part of the assignment. /* Type your code after each of the commented instructions below (except that the statements for instructions 11 and 21 should be written where the instructions indicate). I have written the first statement for you. */ #include <iostream> using namespace std; int main() { // 1. Create two integer variables named x and y. int x; // 2. Create an int pointer named p1. // 3. Store the address of x in p1. // 4. Use only p1 (not x) to set the value of x to 99. // 5. Using cout and x (not p1), display the value of x.…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
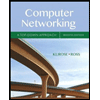
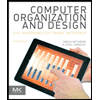
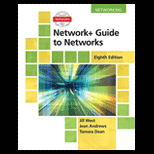
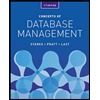
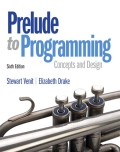
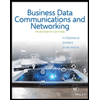