Enter rows of the matrices: 2 Enter Column of the matrices: 2 Enter elements in first matrix of size 2x2 Enter element (0 0): 1 Enter element (0 1): 2 Enter element (1 0): 3 Enter element (1 1): 4 Enter elements in second matrix of size 2x2 Enter element (0 0): 5 Enter element (0 1): 6 Enter element (1 0): 0 Enter element (1 1): 7 First Matrix is: 1 2 з 4 Second Matrix is: 5 6 0 7 Product of both matrices is : 5 20 15 46
In this c langauge program, please change the code but same output.
Source Code:
/**
* C program to multiply two matrix using pointers
*/
#include <stdio.h>
#define ROW 100
#define COL 100
/* Function declarations */
void matrixInput(int mat[][COL],int,int);
void matrixPrint(int mat[][COL],int,int);
void matrixMultiply(int mat1[][COL], int mat2[][COL], int res[][COL],int,int);
int main()
{
int mat1[ROW][COL];
int mat2[ROW][COL];
int product[ROW][COL];
int r,c;
printf("Enter rows of the matrices: ");
scanf("%d",&r);
printf("Enter Column of the matrices: ");
scanf("%d",&c);
/*
* Input elements in matrices.
*/
printf("Enter elements in first matrix of size %dx%d\n", r, c);
matrixInput(mat1,r,c);
printf("Enter elements in second matrix of size %dx%d\n", r, c);
matrixInput(mat2,r,c);
// Call function to multiply both matrices
matrixMultiply(mat1, mat2, product,r,c);
// Print first matrix
printf("First Matrix is: \n");
matrixPrint(mat1,r,c);
// Print Second matrix
printf("Second Matrix is: \n");
matrixPrint(mat2,r,c);
// Print product of both matrix
printf("Product of both matrices is : \n");
matrixPrint(product,r,c);
return 0;
}
/**
* Function to input elements in matrix from user.
*/
void matrixInput(int mat[][COL],int row,int col)
{
int i,j;
for (i = 0; i < row; i++)
{
for (j = 0; j < col; j++)
{
printf("Enter element (%d %d): ",i,j);
scanf("%d", (*(mat + i) + j));
}
}
}
/**
* Function to print elements in a two-dimensional array.
*/
void matrixPrint(int mat[][COL],int row,int col)
{
int i,j;
for (i = 0; i < row; i++)
{
for (j = 0; j < col; j++)
{
printf("%d ", *(*(mat + i) + j));
}
printf("\n");
}
}
/**
* Function to multiply two matrices.
*/
void matrixMultiply(int mat1[][COL], int mat2[][COL], int res[][COL],int row,int col)
{
int i,j,k;
int sum;
for (i = 0; i < row; i++)
{
for (j = 0; j < col; j++)
{
sum = 0;
/*
* Find sum of product of each elements of
* rows of first matrix and columns of second
* matrix.
*/
for (k = 0; k < col; k++)
{
sum += (*(*(mat1 + i) + k)) * (*(*(mat2 + k) + j));
}
/*
* Store sum of product of row of first matrix
* and column of second matrix to resultant matrix.
*/
*(*(res + i) + j) = sum;
}
}
}
See the picture below that's the output of this program. Thank you


Step by step
Solved in 2 steps with 1 images

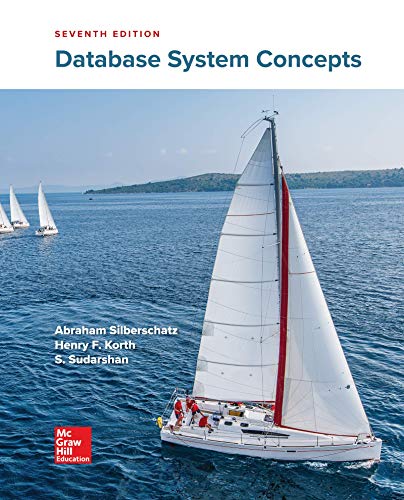
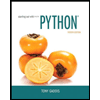
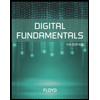
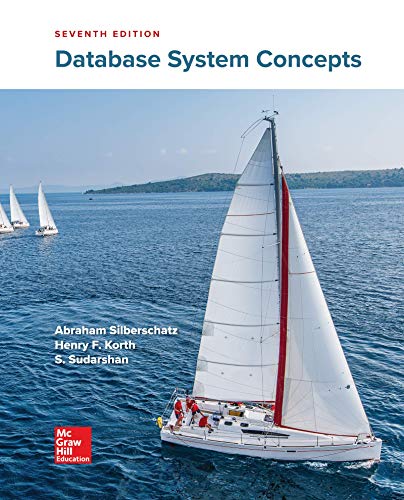
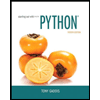
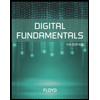
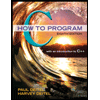
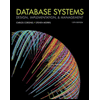
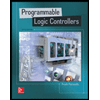